Usar estilo CSS en PHP
- Use CSS en un archivo solo de PHP
- Usar CSS en un archivo PHP+HTML
-
Use CSS en línea en las declaraciones
echo
de PHP
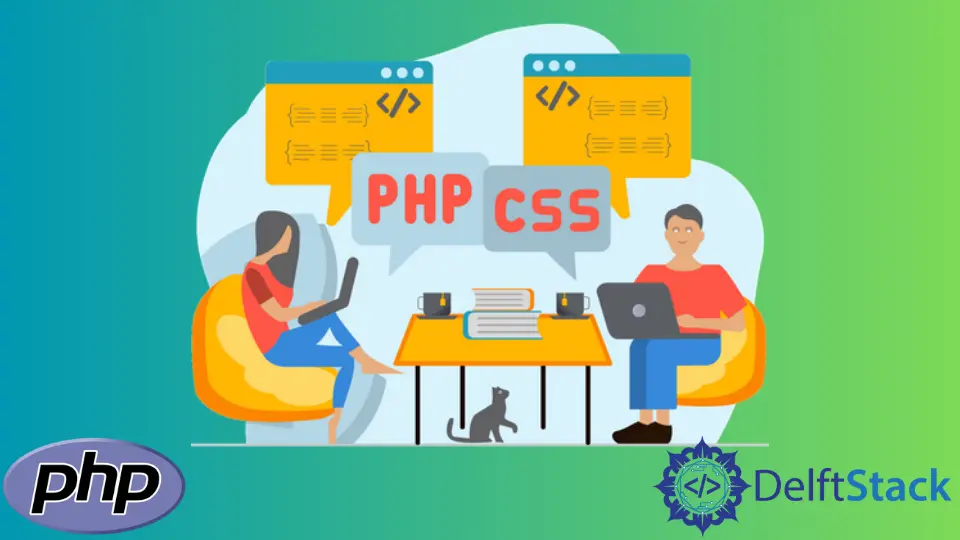
Este artículo te enseñará tres métodos que te ayudarán a usar estilos CSS en PHP.
El primer método es a través de un archivo solo de PHP, y el segundo es incrustar PHP en un archivo HTML+CSS. Luego, el tercer método usará CSS en línea en las declaraciones echo
de PHP.
Use CSS en un archivo solo de PHP
Un archivo HTML estándar puede incrustar estilos CSS en el elemento <head>
o enlazar a un archivo CSS externo. Por defecto, este archivo CSS tendrá la extensión css
, pero también puede tener la extensión php
.
Esto significa que puede escribir código CSS, guardarlo como un archivo PHP y vincularlo a su HTML. En este archivo PHP, puede hacer más de lo que haría en un archivo CSS; puedes escribir código PHP.
Primero, puede definir un bloque de código PHP con propiedad CSS y valores almacenados como variables. Luego, fuera del bloque de PHP, puede escribir CSS normal que usará las variables como valores de las propiedades de CSS.
Lo hemos hecho a continuación; guárdalo como styles.php
.
<?php
// The "header" is the most important part of
// this code. Without it, it will not work.
header("Content-type: text/css");
$font_family = 'Trebuchet MS, Verdana, Helvetica';
$font_size = '1.2em';
$background_color = '#000000';
$font_color = '#ffffff';
// Close the PHP code block.
?>
body {
background-color: <?php echo $background_color; ?>;
color: <?php echo $font_color; ?>;
font-size: <?php echo $font_size; ?>;
font-family: <?php echo $font_family; ?>;
}
Guarde el siguiente HTML y asegúrese de haber vinculado styles.php
en la etiqueta <link>
.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Webpage using CSS styles generated with PHP</title>
<!-- Link styles.php as the CSS file -->
<link rel="stylesheet" type="text/css" href="styles.php">
</head>
<body>
<h1>Hello, We styled this page using CSS in a PHP file!</h1>
<h1>How cool is that?</h1>
</body>
</html>
Producción:
Usar CSS en un archivo PHP+HTML
HTML puede usar CSS a través de la etiqueta <estilo>
o la etiqueta <enlace>
, que puede contener PHP en un bloque PHP dedicado. Si el código PHP genera o manipula código HTML, el código CSS vinculado puede diseñar el HTML.
Por ejemplo, puede diseñar la tabla usando CSS si PHP extrae registros de la base de datos para crear una tabla HTML. Para mostrar cómo hacer esto, cree una base de datos llamada mi_sitio web
en MySQL.
A continuación, cree una tabla site_users
en my_website
usando las siguientes consultas.
CREATE TABLE site_users (
user_id INT NOT NULL AUTO_INCREMENT,
username VARCHAR(50) NOT NULL,
email VARCHAR(100) NOT NULL,
PRIMARY KEY (user_id)) ENGINE = InnoDB;
Inserte datos en la tabla site_users
.
INSERT INTO site_users (username, email) VALUES ('user_1', 'user_1@gmail.com');
INSERT INTO site_users (username, email) VALUES ('user_2', 'user_2@gmail.com');
INSERT INTO site_users (username, email) VALUES ('user_3', 'user_3@gmail.com');
Ahora, a continuación, tenemos HTML, PHP y CSS. El CSS está en el elemento <head>
; el PHP está en un bloque de PHP dentro del HTML.
El PHP crea una tabla HTML usando registros de la tabla site_users
. Cuando el PHP genera la tabla, el CSS le dará estilo.
<!doctype html>
<head>
<meta charset="utf-8">
<title>Style MySQL table with CSS</title>
<style>
/* This CSS will style the table generated by PHP. */
table { border-collapse: collapse; width: 30em; font-size: 1.2em; }
table, th, td { border: 2px solid #1a1a1a; }
td,th { padding: 0.5em; }
/* Create a striped table. */
tr:nth-child(even) { background-color: #f2f2f2; }
/* General body styles. */
body { display: grid; justify-content: center; align-items: center; height: 100vh; }
</style>
</head>
<body>
<main>
<!--
We generated this table using PHP, and the CSS
style in the <style> tag will apply to it.
This is another way to use CSS in PHP.
-->
<?php
// Connect to the database. Change the connection
/// settings based on your environment.
$connect_to_mysql = new mysqli("localhost", "root", "", "my_website");
// Select the data from the site_users table.
$site_users = $connect_to_mysql->query("SELECT * FROM site_users")->fetch_all(MYSQLI_ASSOC);
// Get keys from the first row.
$table_header = array_keys(reset($site_users));
// Print the table.
echo "<table>";
// Print the table headers.
echo "<tr>";
foreach ($table_header as $value) {
echo "<th align='left'>" . $value . "</th>";
}
echo "</tr>";
// Print the table rows
foreach ($site_users as $row) {
echo "<tr>";
foreach ($row as $value) {
if (is_null($value)) {
echo "<td>NULL</td>";
} else {
echo "<td>" . $value . "</td>";
}
}
echo "</tr>";
}
echo "</table>";
?>
</main>
</body>
Producción:
Use CSS en línea en las declaraciones echo
de PHP
PHP funciona bien con HTML y tiene la instrucción echo
que puede enviar HTML con CSS en línea al navegador web. Esto es útil al depurar o enviar grandes fragmentos de HTML al navegador web.
A continuación se muestra cómo usar CSS en línea con PHP echo
. Definimos el texto y almacenamos tres colores en el $colors_array
.
Luego usamos foreach
para recorrer el $colors_array
, y establecemos el elemento de la matriz como el valor del CSS en línea. Cuando ejecuta el código, el texto aparece tres veces con diferentes colores.
<?php
$sample_text = "We generated this text color using inline CSS in PHP.";
$colors_array = ['red', 'green', 'blue'];
foreach ($colors_array as $value) {
// Use inline CSS with PHP echo.
echo "<p style='color: $value'>" . $sample_text . "</p>";
}
?>
Producción:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn