How to Count Files in a Directory in PHP
-
Use the
FilesystemIterator
Class to Count the Files in a Directory in PHP -
Use the
glob()
andcount()
Functions to Count the Files in a Directory in PHP -
Use the
scandir()
andcount()
Functions to Count the Files in a Directory in PHP
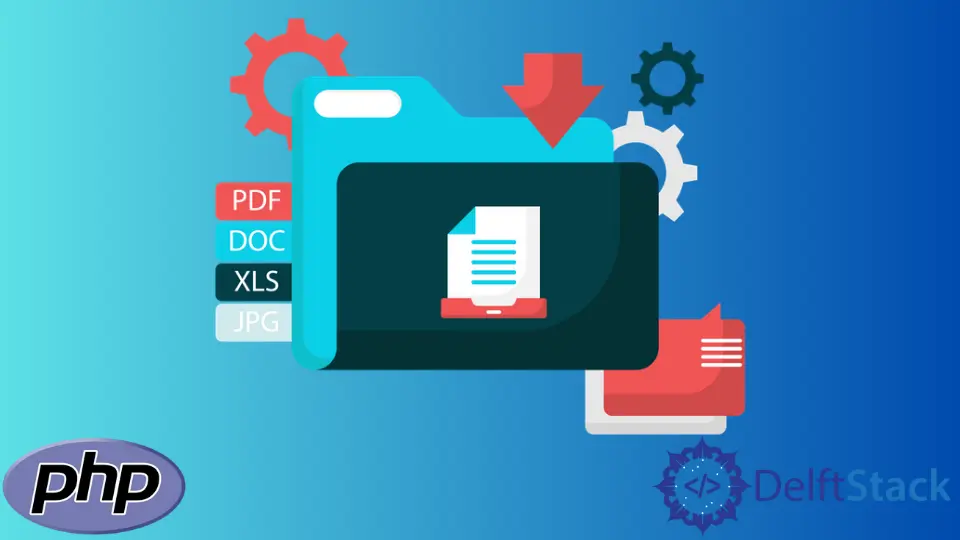
This article will introduce and demonstrate a few methods to count the files in a directory in PHP.
Use the FilesystemIterator
Class to Count the Files in a Directory in PHP
PHP provides the FilesystemIterator
class, an elegant way of counting the files in a directory. The class contains a lot of functions and constants that perform various operations for iterating a filesystem
.
The use of the iterator_count()
function counts the number of elements in the iterator.
Let’s consider the following directory structure.
myDir
├── abc.html
├── abc.jpeg
├── abc.php
└── abc.png
Firstly, create a variable $iterator
and store an instance of the FilesystemIterator()
. Set the first parameter as __DIR__
and use FilesystemIterator::SKIP_DOTS
as the second parameter in the constructor.
The __DIR__
represents the current directory where the script is located, and the second parameter is a flag that skips .
and ..
that exist in a directory. The $iterator
variable contains the filesystem
iterator for the current directory.
It can be iterated to get information like filenames, number of files, etc.
Next, use the iterator_count()
function to print the number of $iterator
in the directory.
Example Code:
$iterator = new FilesystemIterator(__DIR__, FilesystemIterator::SKIP_DOTS);
echo "There are ". iterator_count($iterator). " files in the directory";
Output:
There are 4 files in the directory
Therefore, we can use the FilesystemIterator()
class to count the files in a directory.
Use the glob()
and count()
Functions to Count the Files in a Directory in PHP
We can use the glob()
and count()
functions to get the number of files in a directory. The glob()
function gives an array of the files and the folders that match the specified pattern.
We can get all the files inside the directory using *
. Next, we can use the count()
function to get the number of elements in that array.
For example, create a $path
variable and store the exact path of a directory whose files are to be counted. Next, use the glob()
function to the $path
variable and concatenate the variable with /*
.
As a result, an array of all the files inside the myDir
is created. Finally, use the count()
function on the result of the glob()
function.
Thus, the number of files inside the myDir
directory can be found. The code example below represents the file structure used in the first example.
Example Code:
$path =$_SERVER['DOCUMENT_ROOT']."/myDir";
$num = count(glob($path . "/*"));
echo $num;
Output:
4
Use the scandir()
and count()
Functions to Count the Files in a Directory in PHP
This method is a similar approach to the second method. The only difference here is we use the scandir()
function instead of the glob()
function.
We can supply the directory path as the parameter to the scandir()
function. The function returns an array with all the contents inside the directory, including the .
and ..
.
Next, we can use the count()
function to get the number of the contents inside the directory. Finally, the important part is to subtract 2
from the result of the count()
function to remove the count of .
and ..
.
Thus, we can get the exact number of files inside a directory.
Example Code:
$path =$_SERVER['DOCUMENT_ROOT']."/myDir";
$num = count(scandir($path))-2;
echo "there are $num files in the directory";
Output:
there are 4 files in the directory
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn