How to Create a Folder if It Doesn't Exist in PHP
-
file_exists()
to Check if a File or Directory Exists in PHP -
is_dir()
to Check if a File or Directory Exists in PHP -
file_exists()
vsis_dir()
in PHP -
mkdir()
in PHP
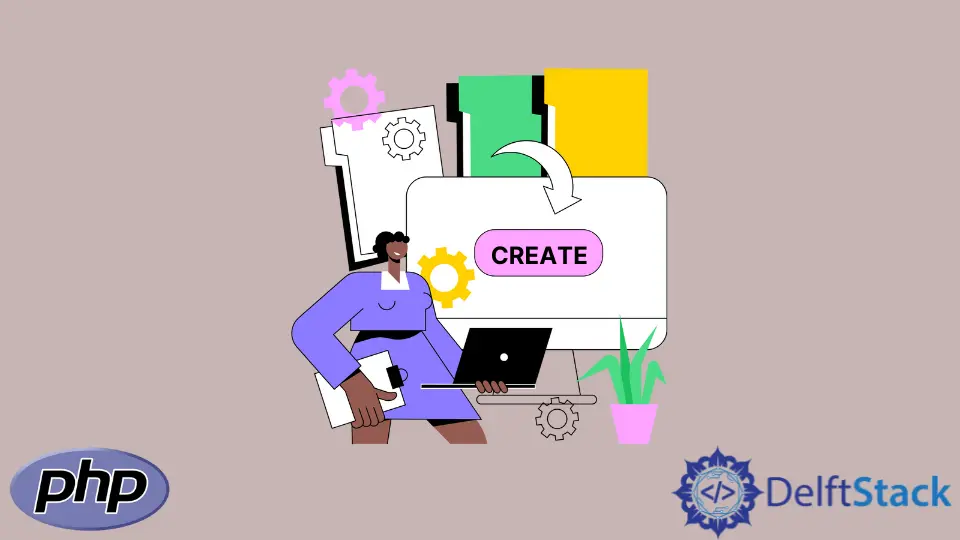
It is possible to create a folder and set the proper permission using PHP, specifically using mkdir()
function.
The default permission mode is 0777
(widest possible access). Before creating a directory, it’s importing to check first if the directory or a file exists or not. In PHP, it can be done using file_exists
or is_dir
.
file_exists()
to Check if a File or Directory Exists in PHP
The file_exists
function is a built-in function to check where a directory or a file exists or not. It accepts a parameter of a path which returns true
if it already exists or false
if not.
Example using file_exists()
:
$path = "sample/path/newfolder";
if (!file_exists($path)) {
mkdir($path, 0777, true);
}
In the above example, it checks the existence of the directory using file_exists()
function, then creates the newfolder
directory if the result is false, with the permission of 0777
.
is_dir()
to Check if a File or Directory Exists in PHP
This function is also similar to file_exists
, and the only difference is that it will only return true
if the passed string is a directory and it will return false
if it’s a file.
Example using is_dir
:
$path = "sample/path/newfolder";
if (!is_dir($path)) {
mkdir($path, 0777, true);
}
In the above example, is_dir
checks whether the folder already exists before creating a new folder using mkdir
.
file_exists()
vs is_dir()
in PHP
Both functions check the existence of the directory, the only difference is file_exists()
also return true
if the passed parameter is a file. On the other hand, is_dir
is a bit faster than file_exists
.
mkdir()
in PHP
This function creates a directory that is specified by pathname which is passed as a parameter. The expected return value is true
or false
.
Example implementation:
mkdir($path, $mode, $recursive, $context);
Parameter Values
Parameter | Values |
---|---|
path (required) |
Directory or path to create |
mode (optional) |
Directory or file permission. By default, the mode is 0777 (widest possible access). The mode is consists of four numbers:1st - Always set to 0 2nd - Specifies the permission of the owner of the directory or file. 3rd - Specifies the permission of the owner’s user group 4th - Specifies the permission of everyone else. |
recursive (optional) |
(true or false ) To create the nested structure, the recursive parameter must set to true . |
context (optional) |
Set of parameters that enhance or modify the stream’s behavior. |
Note: PHP checks if the operating script in the directory has the same UID(owner) in the directory when safe mode
is enabled.