How to Calculate Average of Numbers in PHP
-
Calculate the Average of Numbers in PHP Using
array_sum()
andcount()
-
Calculate the Average of a Continuous Number Set in PHP Using a
while
Loop -
Calculate the Average of Numbers in PHP Using a
foreach
Loop -
Calculate the Average of Numbers in PHP Using
array_reduce
-
Calculate the Average of Numbers in PHP Using
array_map
andarray_sum
- Conclusion
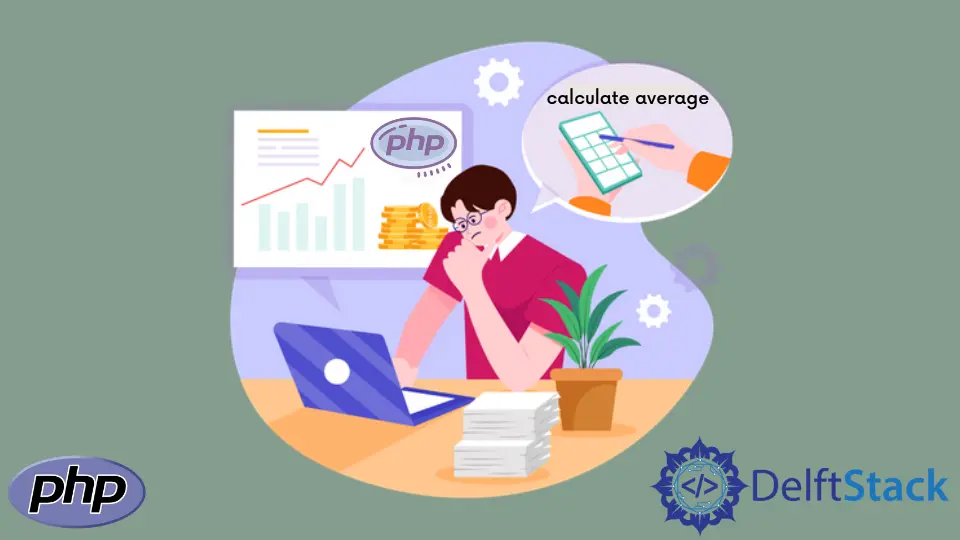
Calculating the average of a set of numbers is a fundamental operation in many programming tasks. PHP, being a versatile and widely used scripting language, offers several methods to compute the average.
In this comprehensive guide, we will explore various approaches to calculating the average of numbers in PHP.
Calculate the Average of Numbers in PHP Using array_sum()
and count()
In PHP, there are several ways to calculate the average of a set of numbers, but one of the simplest and most efficient methods involves using the array_sum()
and count()
functions. These functions allow you to calculate the average without the need for complex loops or extensive coding.
Before we dive into the process of calculating the average, let’s first understand these two essential PHP functions:
The array_sum()
function is used to calculate the sum of all the numeric values in an array. It takes an array as its argument and returns the sum of all the elements in that array.
This function is particularly handy when you have a list of numbers, and you want to find their total.
Here’s the basic syntax of array_sum()
:
$sum = array_sum($array);
$sum
: Stores the sum of the elements in the input array.$array
: Input array containing numeric values that you want to sum.
On the other hand, the count()
function is used to count the number of elements in an array. It returns the count of elements or the length of the array.
This function is helpful when you need to determine how many values are in your array.
Here’s the basic syntax of count()
:
$elementCount = count($array);
$elementCount
: Holds the count of elements in the input array.$array
: Input array whose elements you want to count.
Now that we have a clear understanding of array_sum()
and count()
, let’s see how we can use them to calculate the average of a set of numbers in PHP:
$numbers = [10, 15, 20, 25, 30];
$sum = array_sum($numbers);
$count = count($numbers);
$average = $sum / $count;
echo "The average is: " . $average;
In this example, we have an array of numbers called $numbers
. We first use array_sum()
to find the sum of these numbers and store it in the variable $sum
.
Next, we use count()
to determine how many elements are in the array and store the count in the variable $count
.
Finally, we calculate the average by dividing the sum by the count and storing it in the variable $average
. The result is then displayed on the screen.
To take things a step further, we can generate an array of random numbers and calculate the average. Here’s how it can be done:
$numbers = array_map(function () {
return rand(0, 100);
}, array_fill(0, 193, null));
$sum = array_sum($numbers);
$count = count($numbers);
$average = $sum / $count;
echo "Average: " . $average;
Output:
Average: 51.00518134715
In this case, we’ve created an array of 193
random numbers between 0
and 100
, and the average is calculated as 51.00518134715
.
Note that the value will vary each time due to the random nature of the data.
Handle Edge Cases
When using array_sum()
and count()
to calculate the average of numbers, you should be aware of potential edge cases. Here are some things to consider:
If the input array is empty, both array_sum()
and count()
will return zero. In order to avoid division by zero errors, it’s essential to check if the array is empty before calculating the average.
if (count($numbers) > 0) {
// Calculate the average
} else {
echo "The array is empty.";
}
If the input array contains non-numeric values, you may get unexpected results. To ensure accurate calculations, it’s a good practice to validate the contents of the array and filter out any non-numeric values before calculating the sum and count.
// Filter out non-numeric values
$numbers = array_filter($numbers, 'is_numeric');
Calculate the Average of a Continuous Number Set in PHP Using a while
Loop
While you might often have a pre-defined set of numbers to work with (like in the previous example), there are cases where you need to calculate averages dynamically, especially in applications like education dashboards where you want to continuously track student progress.
In such situations, using a while
loop in PHP can be a powerful tool to calculate the average as new data becomes available.
Imagine you are building an educational platform, and you want to calculate the average weekly performance of a student for as long as they are on their learning path. You don’t know how many weeks they’ll be learning, but you want to calculate the average as they progress.
In this example, we’ll use a while
loop to continuously prompt the user for their weekly performance scores, calculate the average, and display it. To facilitate user input and output, we’ll use the readline()
function and rely on PHP’s array_sum()
and count()
functions to do the heavy lifting.
Let’s create an interactive PHP script that accomplishes this task. You can run this script via the command line using the following command:
php main.php
Example code (main.php
):
<?php
$performanceData = [];
echo "\nAdding numbers repeatedly to get the average at each interval";
echo "\nIf you want to terminate the program, type 0";
while (true) {
echo "\n\n";
$score = (float)readline("Enter a weekly performance score: ");
if ($score !== 0) {
$performanceData[] = $score;
$average = array_sum($performanceData) / count($performanceData);
echo "Current average is " . $average . "\n";
} else {
break;
}
}
$totalEntries = count($performanceData);
$finalAverage = array_sum($performanceData) / $totalEntries;
echo "\nAverage of all the performance scores ($totalEntries) is $finalAverage.";
?>
Initially, an empty array called $performanceData
is created. This array will be used to store the weekly performance scores entered by the user.
The program displays instructions to the user, informing them that they can continually input weekly performance scores and, if they wish to exit, they can do so by typing 0
.
The code enters a while
loop, which will run indefinitely (or until the user decides to exit) as indicated by while (true)
. Within this loop, the program performs the following steps:
- The user is prompted to enter a weekly performance score using the
readline()
function. The entered value is cast to a floating-point number using(float)
to ensure it can handle decimal values. - If the entered score is not equal to
0
, it is considered a valid input. The code then appends the score to the$performanceData
array, calculates the current average by summing all the scores and dividing by the count of scores in the array, and displays this current average on the screen. - If the user enters
0
, the loop is terminated using thebreak
statement, allowing them to exit the program.
After the loop concludes, the program proceeds to calculate the final average and the total number of entries in the $performanceData
array. This information is then displayed on the screen as the Average of all the performance scores
along with the number of entries.
Here’s an example of the script’s interactive shell output:
> php main.php
Adding numbers repeatedly to get the average at each interval
If you want to terminate the program, type 0
Enter a weekly performance score: 11
Current average is 11
Enter a weekly performance score: 3
Current average is 7
Enter a weekly performance score: 6
Current average is 6.6666666666667
Enter a weekly performance score: 88
Current average is 27
Enter a weekly performance score: 1010
Current average is 223.6
Enter a weekly performance score: 0
Average of all the performance scores (5) is 223.6.
In this example, the script keeps updating the average as new performance scores are entered. This dynamic averaging capability can be a valuable feature in various applications, such as educational platforms, sports analytics, and more.
Calculate the Average of Numbers in PHP Using a foreach
Loop
This approach involves iterating through the array of numbers, summing them up, and then dividing by the count of elements in the array.
Here’s a detailed guide on how to do this:
Start by defining an array that contains the series of numbers for which you want to calculate the average. These numbers can be integers, floating-point numbers, or a combination of both.
$numbers = [1, 2.5, 3, 4, 5.5];
Create two variables, $sum
and $count
, to keep track of the sum of the numbers and the count of elements in the array, respectively. Initialize both variables to zero.
$sum = 0;
$count = 0;
Use a foreach
loop to iterate through the array of numbers. In each iteration, add the current number to the $sum
variable and increment the $count
variable.
foreach ($numbers as $number) {
$sum += $number;
$count++;
}
Once the loop has processed all the numbers, calculate the average by dividing the sum ($sum
) by the count ($count
).
$average = $sum / $count;
You can now display the calculated average to the user or use it for further processing in your application.
echo "Average: $average";
Here’s the complete PHP code that calculates the average of a series of numbers using a loop:
<?php
$numbers = [1, 2.5, 3, 4, 5.5];
$sum = 0;
$count = 0;
foreach ($numbers as $number) {
$sum += $number;
$count++;
}
$average = $sum / $count;
echo "Average: $average";
?>
Output:
Average: 3.2
As we can see, the output displayed is "Average: 3.2"
because the sum of the numbers (1
+ 2.5
+ 3
+ 4
+ 5.5
) is 16
, and there are five numbers in the array, resulting in an average of 16 / 5
, which is 3.2
.
Calculate the Average of Numbers in PHP Using array_reduce
PHP’s array_reduce
function is a powerful tool for performing operations on the elements of an array and accumulating a result. It allows us to apply a user-defined callback function to each element of the array, gradually building a final result.
In the case of calculating the average, we can use array_reduce
to both sum the numbers and count the elements in a single operation.
The array_reduce
function in PHP has the following syntax:
array_reduce(array $array, callable $callback, $initial = null): mixed
Let’s break down each part of the syntax:
-
array
: This is the input array that you want to reduce or iterate over. It contains the elements you want to process. -
callback
: The callback function is a callable that defines how the reduction operation is performed. It takes two parameters:$carry
, which accumulates the result, and$item
, which is the current element being processed.The callback function returns the updated value of the
$carry
after processing the current element. -
initial
(optional): This is an optional parameter that sets the initial value of the$carry
.If specified, the initial value is used as the initial
$carry
value. If not provided, the first element of the array is used as the initial$carry
value, and the reduction starts from the second element. -
mixed
:array_reduce
returns the final result of the reduction, which can be of any data type (hence,mixed
).
Start by defining an array that contains the series of numbers for which you want to calculate the average. This array can contain integers, floating-point numbers, or a combination of both.
$numbers = [1, 2.5, 3, 4, 5.5];
The array_reduce
function accepts the array of numbers and a callback function as its arguments. The callback function takes two parameters: $carry
, which accumulates the result, and $number
, which represents the current number being processed.
$result = array_reduce($numbers, function($carry, $number) {
return ['sum' => $carry['sum'] + $number, 'count' => $carry['count'] + 1];
}, ['sum' => 0, 'count' => 0]);
In this example, we use an associative array to keep track of two values, 'sum'
and 'count'
. The 'sum'
value accumulates the sum of the numbers, and the 'count'
value tracks the number of elements in the array.
After the array_reduce
operation is complete, we can calculate the average by dividing the sum ('sum'
) by the count ('count'
).
$average = $result['sum'] / $result['count'];
You can display the calculated average to the user or use it for further processing in your application.
echo "Average: $average";
Here’s the complete PHP code that calculates the average of a series of numbers using array_reduce
:
<?php
$numbers = [1, 2.5, 3, 4, 5.5];
$result = array_reduce($numbers, function($carry, $number) {
return ['sum' => $carry['sum'] + $number, 'count' => $carry['count'] + 1];
}, ['sum' => 0, 'count' => 0]);
$average = $result['sum'] / $result['count'];
echo "Average: $average";
?>
Output:
Average: 3.2
As we can see, the output displayed is "Average: 3.2"
because the sum of the numbers is 16
, and there are five numbers in the array, resulting in an average of 16 / 5
, which is 3.2
.
Calculate the Average of Numbers in PHP Using array_map
and array_sum
The array_map
and array_sum
functions are versatile tools in PHP, allowing you to manipulate arrays and calculate sums efficiently.
The array_map
function allows you to apply a specified callback function to each element of an array. It creates a new array by applying the callback function to each element and collecting the results.
Here’s a closer look at array_map
:
Syntax:
array_map(callable $callback, array $array, array ...$arrays): array
Parameters:
$callback
: This is the callback function to be applied to each element of the array(s). It can be a user-defined function, a built-in function, or an anonymous function.$array
: This is the input array on which you want to apply the callback function.
The array_map
function returns a new array where each element is the result of applying the callback function to the corresponding element in the input array.
In the context of calculating the average, array_map
is used to convert the elements in an array to float values. This ensures data uniformity, allowing you to calculate an accurate average even if the input array contains a mix of integers and floating-point numbers.
In the context of calculating an average, array_map
is used to convert all elements in the array to floats (in case some of them are integers), and array_sum
is used to calculate the sum of the elements.
Here’s a guide on how to calculate the average of a series of numbers using these functions:
Begin by defining an array that contains the series of numbers you want to calculate the average for. This array can include integers, floating-point numbers, or a combination of both.
$numbers = [1, 2.5, 3, 4, 5.5];
To ensure consistent data types (floats), use array_map
to convert all the elements in the array to float values.
$floatNumbers = array_map('floatval', $numbers);
Next, use the array_sum
function to calculate the sum of the float values in the array.
$sum = array_sum($floatNumbers);
Obtain the count of elements in the array. In this case, it’s the count of float values after conversion.
$count = count($floatNumbers);
Now that you have the $sum
and the $count
values calculate the average by dividing the sum by the count.
$average = $sum / $count;
You can display the calculated average to the user or use it for further processing in your application.
echo "Average: $average";
Here’s the complete PHP code for calculating the average of a series of numbers using array_map
and array_sum
:
<?php
$numbers = [1, 2.5, 3, 4, 5.5];
$floatNumbers = array_map('floatval', $numbers);
$sum = array_sum($floatNumbers);
$count = count($floatNumbers);
$average = $sum / $count;
echo "Average: $average";
?>
Output:
Average: 3.2
Similar to the previous examples, the output displayed is "Average: 3.2"
because the sum of the numbers is 16
, and there are five numbers in the array, resulting in an average of 3.2
.
Conclusion
Calculating the average of numbers is a common task in PHP, and there are multiple approaches to achieve this. You can choose the method that best suits your specific requirements and coding style.
Whether you prefer using loops, built-in functions, custom functions, or a combination of these, PHP offers the flexibility to calculate averages efficiently.
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn