在 PHP 中計算數字的平均數
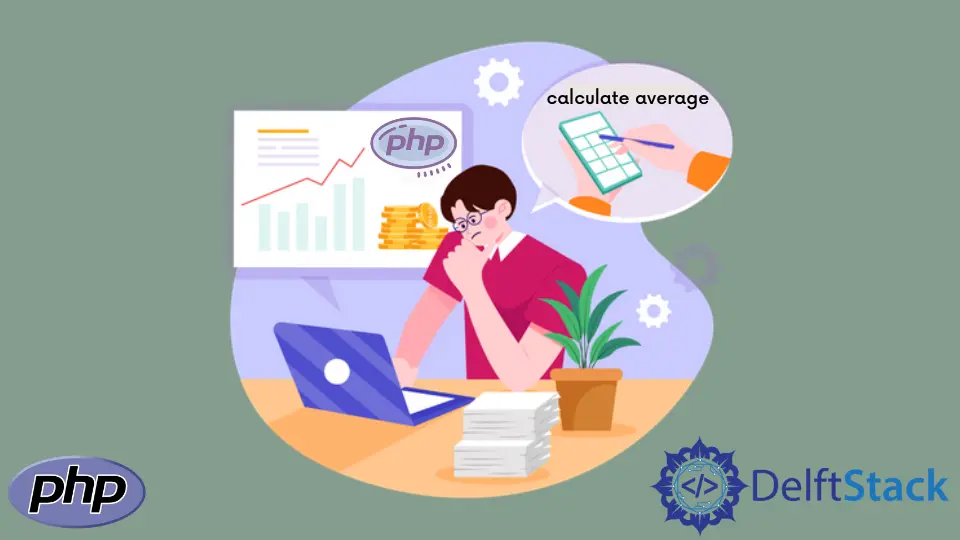
當我們在 PHP 中編碼時,我們會遇到需要執行的不同數學運算。加法、減法、乘法和除法是原生的。
一個典型的就是平均數,也叫均值。在本文中,我們考慮使用 PHP 內建函式來計算一組已知數字和一組連續數字的平均值。
對特定數字集使用 array_sum()
和 count()
簡單的平均公式是數字的總和除以數字的頻率(計數)。因此,要找到 1、3、4、5 和 6 的平均值,我們將新增 1+3+4+5+6,得到 19,然後將 19 除以數字的頻率 5,平均值將為 3.8。
程式碼 - PHP:
<?php
$sum = 1 + 3 + 4 + 5 + 6;
$frequency = 5;
$average = $sum/$frequency;
?>
但是,當我們可以輕鬆計算數字的頻率時,這相當簡單。因此,我們需要諸如 array_sum()
和 count()
之類的函式。
使用這兩個內建函式,你可以輕鬆計算陣列中數字的總和,因為陣列是一種更好的資料結構,可以儲存整數和浮點數等元素序列。
讓我們將相同的數字儲存在一個陣列中並計算平均值。
程式碼:
<?php
$numbers = [1, 3, 4, 5, 6];
$average = array_sum($numbers)/count($numbers);
print_r($average);
?>
輸出:
3.8
讓我們更高階一點,我們自動生成一些隨機數。
<?php
$numbers = array_map(function () {
return rand(0, 100);
}, array_fill(0, 193, null));
$average = array_sum($numbers)/count($numbers);
print_r($average);
?>
輸出:
49.331606217617
你的輸出將與我們的不同,因為陣列中的數字是隨機生成的。
使用 while
迴圈進行連續數字集
前面的例子涵蓋了一個已知數字的列表。但是,在某些情況下,你可能希望隨時計算平均值,例如在用於教育儀表板的 PHP 應用程式中。
假設你想計算學生在該路徑上的每週平均數。我們可以使用 while
迴圈不斷地詢問數字並在每個間隔計算數字。
在這個例子中,我們使用了 readline()
函式和典型的 array_sum()
和 count()
函式。此外,我們將通過以下 shell 語句使用互動式 PHP shell。
php main.php
程式碼 - main.php
:
<?php
$temp = [];
echo "\nAdding numbers repeatedly to get the average at each intervals";
echo "\nIf you want to terminate the program, type 000";
while (True) {
echo "\n\n";
$a = (float)readline("Enter a number: ");
if ($a != 000) {
array_push($temp, $a);
$average = array_sum($temp)/count($temp);
echo "Current average is ". $average;
echo "\n";
} else {
break;
}
}
$frequency = count($temp);
echo "\nAverage of all the numbers ($frequency) is $average.";
?>
$temp
陣列將儲存使用者將輸入的數字。while
迴圈允許我們永遠不斷地請求一個新數字,除非滿足中斷條件,即零 (0
)。
下面的程式碼通過 readline()
函式進行使用者輸入,並確保它是接收到的浮點數。
$a = (float)readline("Enter a number: ");
以下命令將接收一個整數。
$a = (int)readline("Enter a number: ");
我們使用 array_push()
函式將使用者的號碼新增到 $temp
陣列中。之後,我們像之前一樣使用 array_sum()
和 count()
函式計算平均值。
完成後,我們可以輸入 0
來結束程式,這將啟動 break
語句。最後,我們列印所有使用者輸入數字的平均值。
$frequency = count($temp);
echo "\nAverage of all the numbers ($frequency) is $average.";
互動式外殼輸出:
> php main.php
Adding numbers repeatedly to get the average at each interval
If you want to terminate the program, type 0
Enter a number: 11
Current average is 1
Enter a number: 3
Current average is 2
Enter a number: 6
Current average is 3.3333333333333
Enter a number: 88
Current average is 4.5
Enter a number: 1010
Current average is 5.6
Enter a number: 0
Average of all the numbers (5) is 5.6.
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn