How to Add Array to Array in PHP
-
Using the
for
andforeach
Loops to Add Array to Array in PHP -
Using the
array_merge()
Function to Add Array to Array in PHP -
Using the
array_push()
Function to Add Array to Array in PHP
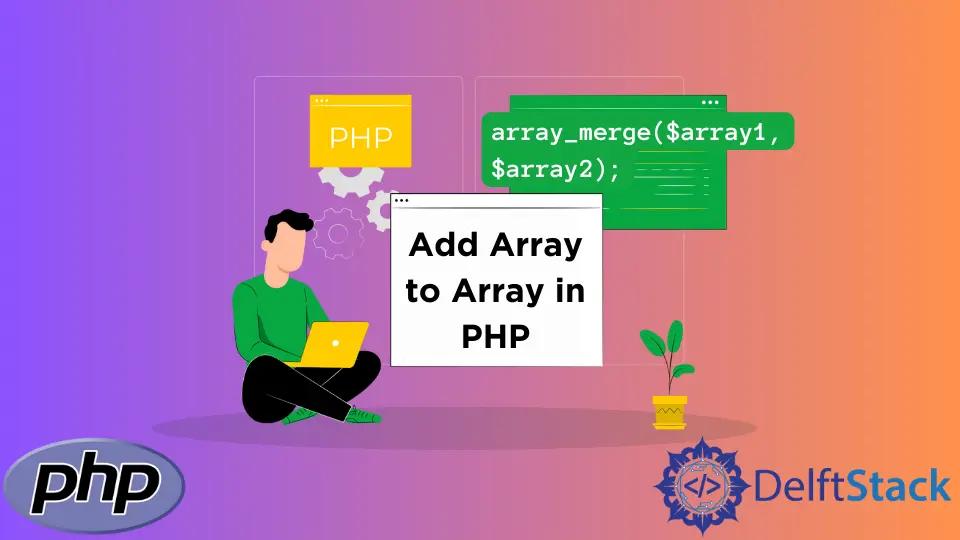
Arrays contain a series of indexed elements of the same data type, often for faster iteration and data management.
Typically, to access array elements, you will loop over the array. For example, in a PHP application, an array could hold data from the registration form, and another array could hold data from the account details section. To use both arrays in one sequence, we need to add both arrays. To achieve this, we need to append the second array to the first, and different functions behave differently.
This tutorial discusses the different methods to add two arrays together to form one array in PHP.
Using the for
and foreach
Loops to Add Array to Array in PHP
A simple method to add an array to another array is to select the second array, loop through all the elements, and append each element to the first array. However, this particular solution is rather long and inefficient for larger arrays.
$container = ["hair extension", "scissors"];
$shopping_lists = ["hair cream", "hair fryer", "makeup set"];
for($index = 0; $index < count($shopping_lists ); $index++){
array_push($container, $shopping_lists[$index]);
}
print_r($container)
Output:
Array
(
[0] hair extension
[1] scissors
[2] hair cream
[3] hair fryer
[4] makeup set
)
true
Also, you can apply the same approach to an associative array. However, it comes with the same inefficiency and complexity.
$customer = array(
"name" => "Garner",
"email" => "g.abded@gmail.com",
"age" => 34,
"gender" => "female",
"account_type" => "starter"
);
$account = array(
"current_course" => "Ruby Crash Course",
"payment_channel" => "Stripe",
"browser" => "Edge"
);
foreach($account as $key => $value) {
$customer[$key] = $value;
}
print_r($customer)
Output:
Array
(
[name] Garner
[email] g.abded@gmail.com
[age] 34
[gender] female
[account_type] starter
[current_course] Ruby Crash Course
[payment_channel] Stripe
[browser] Edge
)
true
Using the array_merge()
Function to Add Array to Array in PHP
The array_merge()
function merges two or more arrays and appends the elements of one array to the end of the previous array, and so on, till the last array. This function works for index, associative and multidimensional arrays. Unlike the previous method, this approach creates a new array and does not append to the first array.
This method can work with multiple arrays. In more detail, we can use this approach to add the key-value pair (associative arrays) to one another to form one single array. The same goes for index arrays.
$details = [
"name" => "Clement",
"email" => "clement@gmail.com",
"gender" => "male"
];
$accounts = [
"card" => "mastercard",
"processor" => "stripe",
"pro" => True
];
$account_details = array_merge($details, $accounts);
print_r($account_details);
Output:
Array
(
[name] Clement
[email] clement@gmail.com
[gender] male
[card] mastercard
[processor] stripe
[pro] 1
)
true
Here is how to use the array_merge()
function on three arrays.
$details = [
"name" => "Clement",
"email" => "clement@gmail.com",
"gender" => "male"
];
$accounts = [
"card" => "mastercard",
"processor" => "stripe",
"pro" => True
];
$functions = [
"movies" => "inferno"
];
$account_details = array_merge($details, $accounts, $functions);
print_r($account_details);
Output:
Array
(
[name] Clement
[email] clement@gmail.com
[gender] male
[card] mastercard
[processor] stripe
[pro] 1
[movies] inferno
)
true
This method is compatible with all PHP 7.0 versions and above.
Using the array_push()
Function to Add Array to Array in PHP
The array_push()
function pushes the array(s) onto the end of the array like a stack (LIFO). You can use this function to add an index array to an associative array, and it will automatically create a numerical index for the index array pushed to the associative array. If two index arrays are pushed, the first index array holds the numerical index of 0, and the second index array holds the numerical index of 1. For N arrays
pushed, the numerical index will be N-1
.
Additionally, you can push index arrays to an index array and associative arrays to an associative array.
$basic_data = ['Location' => 'Mumbai', 'Tier' => 'Three'];
$tools = ['Geology', 'Machine Learning'];
$BD_Tools = array_push($basic_data, $tools);
print_r($basic_data);
Output:
Array
(
[Location] Mumbai
[Tier] Three
[0] Array
(
[0] Geology
[1] Machine Learning
)
)
true
In addition, use the ...
operator within the array_push()
function to allow all the elements within the pushed array(s) to have their own numerical index rather than one for all.
$basic_data = ['Location' => 'Mumbai', 'Tier' => 'Three'];
$tools = ['Geology', 'Machine Learning'];
$BD_Tools = array_push($basic_data, ...$tools);
print_r($basic_data);
Output:
Array
(
[Location] Mumbai
[Tier] Three
[0] Geology
[1] Machine Learning
)
true
For associative array push operations, you can’t use the ...
operator, as it will throw an error.
TypeError: array_push() does not accept unknown named parameters null
Therefore, the only way to use array_push()
function with two or more arrays is via the default means.
$basic_data = ['Location' => 'Mumbai', 'Tier' => 'Three'];
$tools = ['Course' => 'Geology', 'Approach' => 'Machine Learning'];
$BD_Tools = array_push($basic_data, $tools);
print_r($basic_data);
Output:
Array
(
[Location] Mumbai
[Tier] Three
[0] Array
(
[Course] Geology
[Approach] Machine Learning
)
)
true
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn