在 PHP 中将数组添加到数组
-
在 PHP 中使用
for
和foreach
循环将数组添加到数组 -
在 PHP 中使用
array_merge()
函数将数组添加到数组 -
在 PHP 中使用
array_push()
函数将数组添加到数组
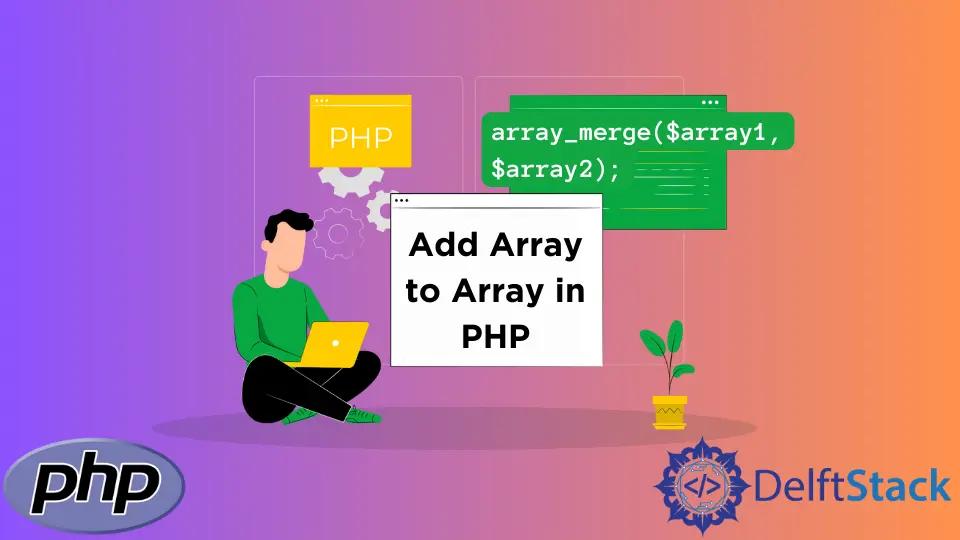
数组包含一系列相同数据类型的索引元素,通常用于更快的迭代和数据管理。
通常,要访问数组元素,你将遍历数组。例如,在 PHP 应用程序中,一个数组可以保存注册表单中的数据,而另一个数组可以保存帐户详细信息部分的数据。要在一个序列中使用两个数组,我们需要添加两个数组。为此,我们需要将第二个数组附加到第一个数组,不同的函数表现不同。
本教程讨论了在 PHP 中将两个数组相加形成一个数组的不同方法。
在 PHP 中使用 for
和 foreach
循环将数组添加到数组
将一个数组添加到另一个数组的一种简单方法是选择第二个数组,遍历所有元素,然后将每个元素附加到第一个数组。但是,对于较大的阵列,这种特殊的解决方案相当长且效率低下。
$container = ["hair extension", "scissors"];
$shopping_lists = ["hair cream", "hair fryer", "makeup set"];
for($index = 0; $index < count($shopping_lists ); $index++){
array_push($container, $shopping_lists[$index]);
}
print_r($container)
输出:
Array
(
[0] hair extension
[1] scissors
[2] hair cream
[3] hair fryer
[4] makeup set
)
true
此外,你可以将相同的方法应用于关联数组。但是,它具有同样的低效率和复杂性。
$customer = array(
"name" => "Garner",
"email" => "g.abded@gmail.com",
"age" => 34,
"gender" => "female",
"account_type" => "starter"
);
$account = array(
"current_course" => "Ruby Crash Course",
"payment_channel" => "Stripe",
"browser" => "Edge"
);
foreach($account as $key => $value) {
$customer[$key] = $value;
}
print_r($customer)
输出:
Array
(
[name] Garner
[email] g.abded@gmail.com
[age] 34
[gender] female
[account_type] starter
[current_course] Ruby Crash Course
[payment_channel] Stripe
[browser] Edge
)
true
在 PHP 中使用 array_merge()
函数将数组添加到数组
array_merge()
函数合并两个或多个数组,并将一个数组的元素附加到前一个数组的末尾,依此类推,直到最后一个数组。此函数适用于索引、关联和多维数组。与前面的方法不同,这种方法创建一个新数组并且不会追加到第一个数组。
此方法可以与多个数组一起使用。更详细地说,我们可以使用这种方法将键值对(关联数组)相互添加以形成一个单独的数组。索引数组也是如此。
$details = [
"name" => "Clement",
"email" => "clement@gmail.com",
"gender" => "male"
];
$accounts = [
"card" => "mastercard",
"processor" => "stripe",
"pro" => True
];
$account_details = array_merge($details, $accounts);
print_r($account_details);
输出:
Array
(
[name] Clement
[email] clement@gmail.com
[gender] male
[card] mastercard
[processor] stripe
[pro] 1
)
true
以下是如何在三个数组上使用 array_merge()
函数。
$details = [
"name" => "Clement",
"email" => "clement@gmail.com",
"gender" => "male"
];
$accounts = [
"card" => "mastercard",
"processor" => "stripe",
"pro" => True
];
$functions = [
"movies" => "inferno"
];
$account_details = array_merge($details, $accounts, $functions);
print_r($account_details);
输出:
Array
(
[name] Clement
[email] clement@gmail.com
[gender] male
[card] mastercard
[processor] stripe
[pro] 1
[movies] inferno
)
true
此方法兼容所有 PHP 7.0 及以上版本。
在 PHP 中使用 array_push()
函数将数组添加到数组
array_push()
函数将数组推送到数组的末尾,就像堆栈 (LIFO)。你可以使用【此功能】将索引数组添加到关联数组中,它会自动为推送到关联数组的索引数组创建一个数字索引。如果推送两个索引数组,第一个索引数组保存数字索引 0,第二个索引数组保存数字索引 1。对于推送的 N 个数组
,数字索引将为 N-1
。
此外,你可以将索引数组推送到索引数组,将关联数组推送到关联数组。
$basic_data = ['Location' => 'Mumbai', 'Tier' => 'Three'];
$tools = ['Geology', 'Machine Learning'];
$BD_Tools = array_push($basic_data, $tools);
print_r($basic_data);
输出:
Array
(
[Location] Mumbai
[Tier] Three
[0] Array
(
[0] Geology
[1] Machine Learning
)
)
true
此外,使用 array_push()
函数中的 ...
运算符允许被推入的数组中的所有元素都有自己的数字索引,而不是一个为所有的。
$basic_data = ['Location' => 'Mumbai', 'Tier' => 'Three'];
$tools = ['Geology', 'Machine Learning'];
$BD_Tools = array_push($basic_data, ...$tools);
print_r($basic_data);
输出:
Array
(
[Location] Mumbai
[Tier] Three
[0] Geology
[1] Machine Learning
)
true
对于关联数组推送操作,不能使用 ...
运算符,因为它会引发错误。
TypeError: array_push() 不接受未知的命名参数 null
因此,对两个或更多数组使用 array_push()
函数的唯一方法是通过默认方式。
$basic_data = ['Location' => 'Mumbai', 'Tier' => 'Three'];
$tools = ['Course' => 'Geology', 'Approach' => 'Machine Learning'];
$BD_Tools = array_push($basic_data, $tools);
print_r($basic_data);
输出:
Array
(
[Location] Mumbai
[Tier] Three
[0] Array
(
[Course] Geology
[Approach] Machine Learning
)
)
true
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn