How to Use jQuery in PHP
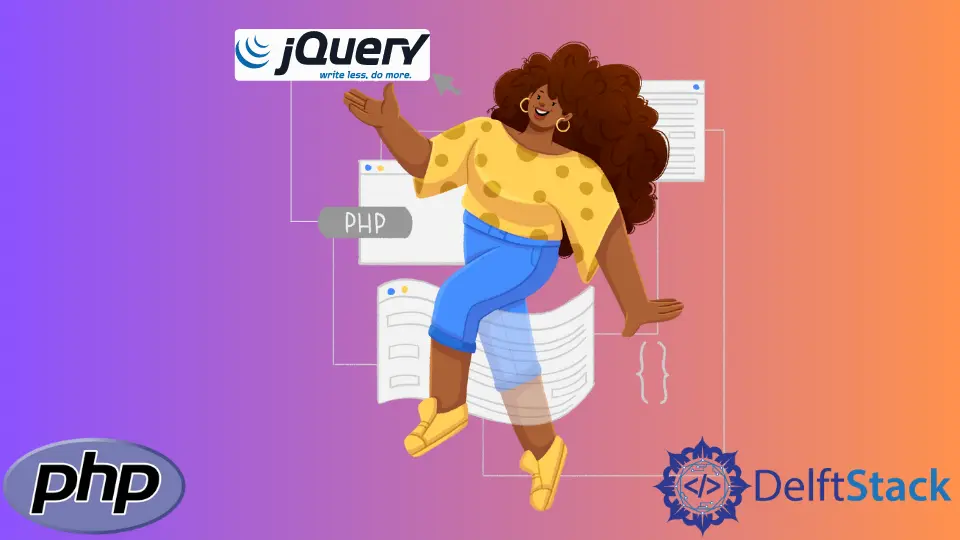
We can use different JavaScript libraries within our PHP application, and jQuery is popular. Though people now use React or Vue more, there are still more than 150,262,730 websites using jQuery.
For some DOM manipulation, jQuery provides functions and features that make it easy to achieve our goals, especially within our PHP application.
This article will show you how to use jQuery within your PHP code.
Use <script>
to Use jQuery in PHP
As with any JavaScript file, which jQuery is, we need the <script>
tag to include it. You can place the <script>
tag within your HTML or PHP file using the src
attribute to specify the location of the jQuery library.
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
The above code makes use of the jQuery version 3.6.0 CDN. If we intend to use it locally, we can make use of the below code (depending on where we place the jQuery library within our environment, the path).
<script src="jquery-3.6.0.min.js"></script>
Where we place the <script>
matters; we should always place it towards the end of our PHP or HTML file (especially before the end of the body tag).
This is to improve rendering performance and prevent slow loading in the case of slow JavaScript response.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<?php
echo "Hello, PHP Admin";
?>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
</body>
</html>
Also, you can save the location of your jQuery file within a PHP variable and echo
the location where you need it.
<script src="<?php echo $jQuery; ?>"></script>
Let’s play with jQuery within a PHP codebase and hide a paragraph using the jQuery library.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<?php
$user = "Jinku";
echo "<h1>Hello " . $user . ", PHP Admin</h1>";
?>
<h2>This is a heading</h2>
<p>This is a paragraph.</p>
<p id="test">This is another paragraph.</p>
<button>Click me</button>
<?php
$jQuery = "https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js";
?>
<script src="<?php echo $jQuery; ?>"></script>
<script>
$(document).ready(function() {
$("button").click(function() {
$("#test").hide();
});
});
</script>
</body>
</html>
The output of the code in a browser before clicking the button.
After clicking the button, the paragraph hides.
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn