How to Insert Form Data Using MySQL Table in PHP
- Create an HTML Form to Collect the Data
-
Use the
POST
Method to Send the Data in Our HTML Form to a Table in Our Database in PHP
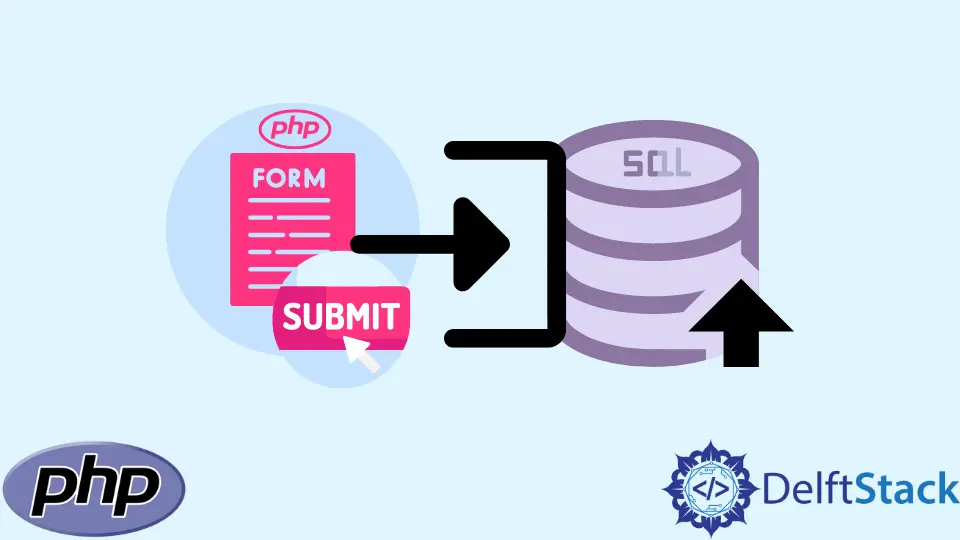
This tutorial will cover how we can send data from an HTML form to a table in our database.
To achieve this, we will use the following steps:
- First, we will create a basic HTML form to collect data.
- We will then use the
POST
method to send the data in our HTML form to a table in our database.
We have a table called parkinglot1
in our database sample tutorial
. Below is what the table looks like:
Let’s assume we have a new entry to add to the table. Our new entry will be as follows, Ferrari
for BrandName
, Alex
for OwnersName
and, KJG564R
for RegistrationNumber
.
How do we approach this? The first is to create an HTML form to collect the new data.
Create an HTML Form to Collect the Data
We will have three slots to input data and a submit button in our HTML form. We do not need to include a CarID
slot as PHP automatically updates the entry.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Form- Store Data</title>
</head>
<body>
<center>
<h1>This Form is For Storing Data for OUR Database</h1>
<form action="includes/insert.php" method="POST">
<p>
<label for="CarID">Car ID:</label>
<input type="text" name="CarID" id="CarID">
</p>
<p>
<label for="BrandName">Brand Name:</label>
<input type="text" name="BrandName" id="BrandName">
</p>
<p>
<label for="OwnersName">Owners Name:</label>
<input type="text" name="OwnersName" id="OwnersName">
</p>
<p>
<label for="RegistrationNumber">Registration Number:</label>
<input type="text" name="RegistrationNumber" id="RegistrationNum">
</p>
<input type="submit" value="Submit">
</form>
</center>
</body>
</html>
Output:
Now we can write the code to send data to our database.
Use the POST
Method to Send the Data in Our HTML Form to a Table in Our Database in PHP
<?php
$user = 'root';
$pass = '';
$db = 'sample tutorial';
//Replace 'sample tutorial' with the name of your database
$con = mysqli_connect("localhost", $user, $pass, $db);
if ($con->connect_error) {
die("Connection failed: " . $con->connect_error);
}
$CarID = $_POST['CarID'];
$BrandName = $_POST['BrandName'];
$OwnersName = $_POST['OwnersName'];
$RegistrationNumber = $_POST['RegistrationNumber'];
$sql = "INSERT INTO parkinglot1 (CarID,BrandName,OwnersName, RegistrationNumber)
VALUES ('$CarID','$BrandName','$OwnersName', '$RegistrationNumber')";
mysqli_query($con, $sql);
header("Location: ../form.php"); // Return to where our form is stored
?>
The header()
function will return us to our HTML form where we can fill in the values we want to insert as shown below:
After we submit the data, our updated table should look like this:
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn