在 PHP 中使用 MySQL 表插入表单数据
John Wachira
2024年2月15日
PHP
MySQL
PHP Insert
MySQL Table
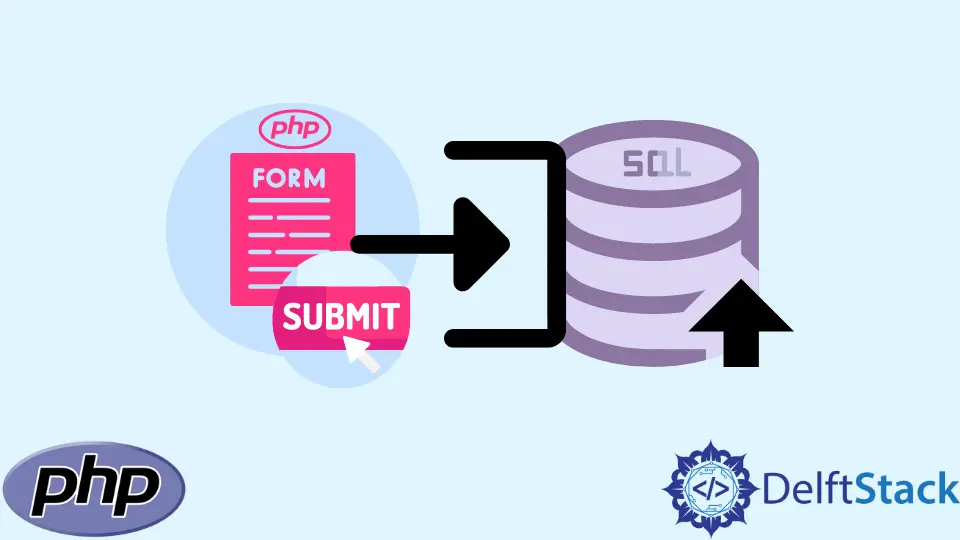
本教程将介绍如何将数据从 HTML 表单发送到数据库中的表。
为此,我们将使用以下步骤:
- 首先,我们将创建一个基本的 HTML 表单来收集数据。
- 然后我们将使用
POST
方法将我们的 HTML 表单中的数据发送到我们数据库中的一个表中。
我们的数据库 sample tutorial
中有一个名为 parkinglot1
的表。下面是表格的样子:
假设我们有一个新条目要添加到表中。我们的新条目如下,BrandName
为 Ferrari
,OwnersName
为 Alex
,RegistrationNumber
为 KJG564R
。
我们如何处理这个问题?首先是创建一个 HTML 表单来收集新数据。
创建一个 HTML 表单来收集数据
我们将在 HTML 表单中拥有三个输入数据的插槽和一个提交按钮。我们不需要包含 CarID
插槽,因为 PHP 会自动更新条目。
<!DOCTYPE html>
<html lang="en">
<head>
<title>Form- Store Data</title>
</head>
<body>
<center>
<h1>This Form is For Storing Data for OUR Database</h1>
<form action="includes/insert.php" method="POST">
<p>
<label for="CarID">Car ID:</label>
<input type="text" name="CarID" id="CarID">
</p>
<p>
<label for="BrandName">Brand Name:</label>
<input type="text" name="BrandName" id="BrandName">
</p>
<p>
<label for="OwnersName">Owners Name:</label>
<input type="text" name="OwnersName" id="OwnersName">
</p>
<p>
<label for="RegistrationNumber">Registration Number:</label>
<input type="text" name="RegistrationNumber" id="RegistrationNum">
</p>
<input type="submit" value="Submit">
</form>
</center>
</body>
</html>
输出:
现在我们可以编写代码将数据发送到我们的数据库。
使用 POST
方法将 HTML 表单中的数据发送到 PHP 数据库中的表中
<?php
$user = 'root';
$pass = '';
$db = 'sample tutorial';
//Replace 'sample tutorial' with the name of your database
$con = mysqli_connect("localhost", $user, $pass, $db);
if ($con->connect_error) {
die("Connection failed: " . $con->connect_error);
}
$CarID = $_POST['CarID'];
$BrandName = $_POST['BrandName'];
$OwnersName = $_POST['OwnersName'];
$RegistrationNumber = $_POST['RegistrationNumber'];
$sql = "INSERT INTO parkinglot1 (CarID,BrandName,OwnersName, RegistrationNumber)
VALUES ('$CarID','$BrandName','$OwnersName', '$RegistrationNumber')";
mysqli_query($con, $sql);
header("Location: ../form.php"); // Return to where our form is stored
?>
header()
函数将返回我们的 HTML 表单,我们可以在其中填写我们想要插入的值,如下所示:
提交数据后,更新后的表格应如下所示:
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: John Wachira
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn