How to Find the Foreach Index in PHP
-
Finding the
index
Using theforeach
Loop in PHP -
Use the
key
andvalue
Variables to Find theforeach
Index in PHP -
Use a Counter Variable to Find the
foreach
Index in PHP -
Use the
array_keys
Function to Find theforeach
Index in PHP -
Use the
array_values
Function to Find theforeach
Index in PHP - Conclusion
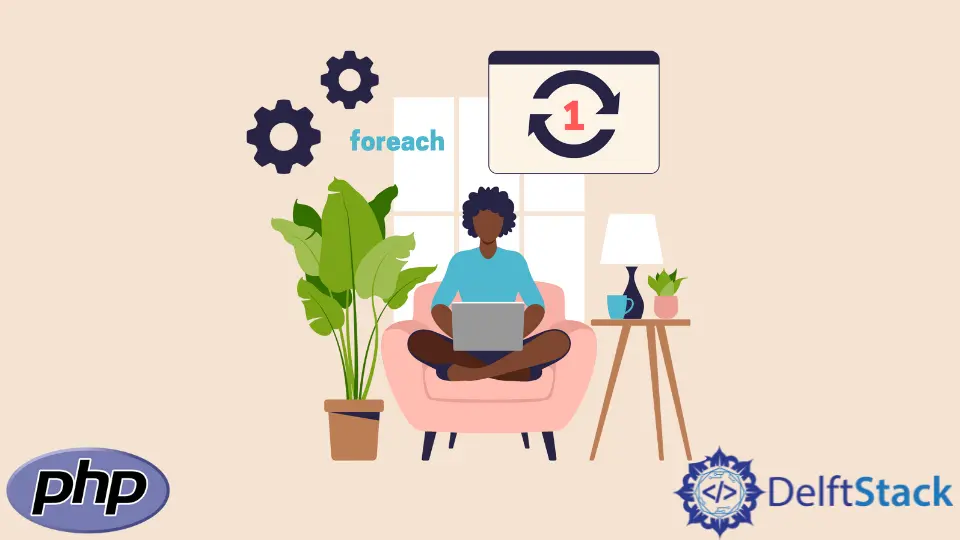
In the realm of PHP development, array manipulation is a key skill.
The versatile foreach
loop simplifies accessing each element in an array without the need for complex indexing logic. However, it doesn’t directly expose the index of each element during iteration.
This article guides developers to find the foreach
index using various methods. From classic techniques using key
and value
variables to leveraging specialized functions like array_keys
and array_values
, this guide equips developers with the tools to enhance array handling.
Finding the index
Using the foreach
Loop in PHP
The foreach
loop provides an efficient way to iterate over each element in an array, making it easier to perform actions or manipulations on the array’s contents in a clear and organized manner within your PHP code.
Syntax:
foreach($array as $value){
//code
}
The PHP code above utilizes the foreach
loop to sequentially go through every element present in an array. The structure of the loop is simple: for each element in the array, the loop executes a specified set of instructions.
Within the loop, the variable $value
serves as a temporary container for the current array element being processed. It’s like a placeholder that changes with each iteration, taking on the value of the current array element.
The section marked with // code
is where you can include the specific instructions or operations you want to perform for each element in the array.
The loop keeps running until it has traversed through all the elements in the array, and during each cycle, $value
allows you to work with the individual element in focus.
Use the key
and value
Variables to Find the foreach
Index in PHP
The key
and value
variables within a foreach
loop provide a seamless way to access both the index and value of each element in an array during iteration.
This straightforward approach not only enhances the ability to understand the array structure but also provides a clear and concise method for extracting both the index and value effortlessly.
Take a look at the sample code below:
<?php
$array = array(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
foreach ($array as $key => $value) {
echo "The index is = " . $key . ", and value is = ". $value;
echo "\n";
}
The code above initializes an array named $array
containing the numbers from 1
to 10
. It then employs a foreach
loop to iterate through each element of the array.
Within the loop, the code uses the $key
variable to capture the index of the current array element and the $value
variable to obtain the actual value stored at that index.
For each iteration, it echoes a message to the console indicating the index and value of the array element. The output of the code will display a series of lines, each stating the index and corresponding value of the elements in the array.
Output:
The index is = 0, and value is = 1
The index is = 1, and value is = 2
The index is = 2, and value is = 3
The index is = 3, and value is = 4
The index is = 4, and value is = 5
The index is = 5, and value is = 6
The index is = 6, and value is = 7
The index is = 7, and value is = 8
The index is = 8, and value is = 9
The index is = 9, and value is = 10
As the output shows, this practical approach is foundational for tasks involving array manipulation and analysis in PHP, offering a clear and concise method for accessing array elements.
Use a Counter Variable to Find the foreach
Index in PHP
A counter variable is a variable used to keep track of a count within a program. It is commonly used in loops and iterative processes.
Sample counter variable:
// Initializing a counter variable
$index = 0;
In the code above, the value of $index
is initially 0
.
By initializing a counter variable ($index
) before the foreach
loop and incrementing it with each iteration, developers can effectively obtain the current index.
Take a look at the example code below:
<?php
// Declare an array
$arr = array(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
$index = 0;
foreach ($arr as $key => $val) {
echo "The index is $index";
$index++;
echo "\n";
}
This PHP code initializes an array named $arr
containing the values from 1
to 10
. It then declares a counter variable $index
and sets it to 0
.
A foreach
loop then iterates through each element of the array. Within the loop, it echoes a statement indicating the current value of the $index
variable.
After displaying the index, the code increments the $index
by 1
. This process continues until all elements in the array have been traversed.
The result is a series of output lines, each indicating the index of the corresponding array element.
Output:
The index is 0
The index is 1
The index is 2
The index is 3
The index is 4
The index is 5
The index is 6
The index is 7
The index is 8
The index is 9
Use the array_keys
Function to Find the foreach
Index in PHP
The array_keys
function in PHP is a built-in function that is used to retrieve all the keys or a subset of keys from an array. It takes an array as its argument and returns a new array containing the keys.
Syntax:
array_keys($array);
When used in the context of a foreach
loop, array_keys
becomes a powerful means of obtaining the indices of an array without directly iterating over the array itself.
Consider the example code below:
<?php
// Declare an array
$arr = array(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
$keys = array_keys($arr);
foreach ($keys as $index) {
// $index contains the current index
$value = $arr[$index];
echo "Index: $index, Value: $value\n";
}
The code begins by declaring an array named $arr
containing the values from 1
to 10
. Subsequently, it creates another array named $keys
using the array_keys
function, which retrieves all the keys or indices from the original array.
The code then enters a foreach
loop, iterating over each element in the $keys
array.
Within the loop, the variable $index
represents the current index being processed. Using this index, the value from the original array $arr
is accessed and assigned to the variable $value
.
For each iteration, the code prints a message that includes the current index ($index
) and its value ($value
). This process repeats until all indices in the array have been traversed.
Output:
Index: 0, Value: 1
Index: 1, Value: 2
Index: 2, Value: 3
Index: 3, Value: 4
Index: 4, Value: 5
Index: 5, Value: 6
Index: 6, Value: 7
Index: 7, Value: 8
Index: 8, Value: 9
Index: 9, Value: 10
Use the array_values
Function to Find the foreach
Index in PHP
The array_values
function in PHP is used to return all the values of an array while discarding the keys. It essentially reindexes the array numerically, starting from zero.
Syntax:
array_values($array);
In the syntax above, the array_values
function takes an associative array as input and returns a new array containing only the values, reindexed numerically.
When used in the context of a foreach
loop, array_values
can indirectly help in obtaining the index of the current element. This allows developers to access the indices indirectly through the loop variable.
To understand this better, take a look at the example code below:
<?php
// Declare an array
$arr = array(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
$values = array_values($arr);
foreach ($values as $index => $value) {
// $index contains the current index
$value = $arr[$index];
echo "Index: $index, Value: $value\n";
}
This PHP code begins by declaring an array named $arr
containing the integers from 1
to 10
.
Following this, a new array named $values
is created using the array_values()
function, which extracts the values from the original array and reindexes them numerically.
The code then runs a foreach
loop, iterating through each element in the $values
array. Within the loop, two variables, $index
and $value
, are used to represent the current index and value of the array element, respectively.
The $index
variable is used to access the value from the original array $arr
. That value is then stored in the $value
variable.
Next, the echo
statement outputs the current index and value in a formatted string. This process continues until all elements in the array have been traversed.
Output:
Index: 0, Value: 1
Index: 1, Value: 2
Index: 2, Value: 3
Index: 3, Value: 4
Index: 4, Value: 5
Index: 5, Value: 6
Index: 6, Value: 7
Index: 7, Value: 8
Index: 8, Value: 9
Index: 9, Value: 10
Take note that while array_values
can indirectly help in obtaining indices in a foreach
loop, it’s not the only method available, and the choice of method depends on the specific use case and preference.
Conclusion
In wrapping up, this article uncovers finding the foreach
index in PHP. The methods explored — using key
and value
, a counter variable, array_keys
, and array_values
— provide diverse ways to streamline array iteration.
Understanding these methods not only enhances the ability to navigate arrays efficiently but also underscores the flexibility and adaptability of PHP’s foreach
loop.
The simplicity of the foreach
loop, combined with the techniques discussed, empowers developers to write concise and readable code while effortlessly managing array traversal.