Cómo encontrar el índice de foreach en PHP
-
Usando la variable
key
para encontrar el índice foreach en PHP -
Usando la variable
index
para encontrar el índice de foreach en PHP -
Usando tanto la variable
key
como laindex
para encontrar el índice foreach en PHP
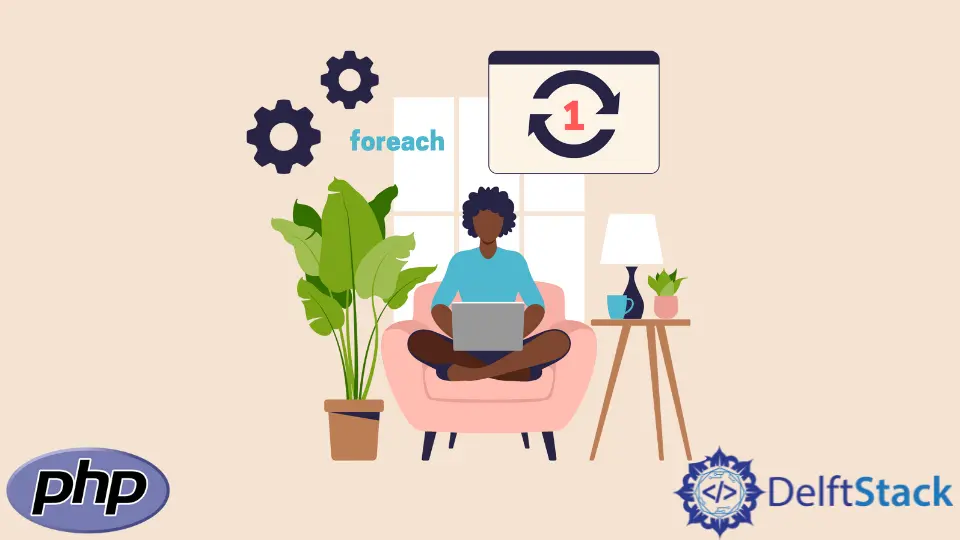
En este artículo, introduciremos métodos para encontrar el índice foreach
.
- Usando la variable
key
- Usando la variable
index
- Usando tanto la
key
como elindex
variable
Usando la variable key
para encontrar el índice foreach en PHP
La clave variable almacena el índice de cada valor en el bucle foreach
. El bucle foreach
en PHP se usa de la siguiente manera.
foreach($arrayName as $value){
//code
}
El valor de la variable almacena el valor de cada elemento del array.
<?php
$array = array(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
foreach ($array as $key => $value) {
echo "The index is = " . $key . ", and value is = ". $value;
echo "\n";
}
?>
La variable clave aquí contiene el índice del bucle foreach
. El valor de la variable muestra el valor de cada elemento de la array.
Producción :
The index is = 0, and the value is = 1
The index is = 1, and the value is = 2
The index is = 2, and the value is = 3
The index is = 3, and the value is = 4
The index is = 4, and the value is = 5
The index is = 5, and the value is = 6
The index is = 6, and the value is = 7
The index is = 7, and the value is = 8
The index is = 8, and the value is = 9
The index is = 9, and the value is = 10
Usando la variable index
para encontrar el índice de foreach en PHP
El índice de la variable se utiliza como una variable adicional para mostrar el índice de foreach
en cada iteración.
<?php
// Declare an array
$arr = array(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
$index = 0;
foreach($arr as $key=>$val) {
echo "The index is $index";
$index++;
echo "\n";
}
?>
Producción :
The index is 0
The index is 1
The index is 2
The index is 3
The index is 4
The index is 5
The index is 6
The index is 7
The index is 8
The index is 9
Usando tanto la variable key
como la index
para encontrar el índice foreach en PHP
Ahora, usaremos tanto la variable clave como una variable adicional para encontrar el índice de foreach
.
<?php
// Declare an array
$arr = array(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
$index = 0;
foreach ($arr as $key=>$value) {
echo "The index is $index";
$index = $key+1;
echo "\n";
}
?>
Hemos almacenado el valor de la variable clave con un incremento en su valor en la variable índice. De esta manera, podemos encontrar el índice de foreach
usando tanto la variable clave como la variable índice.
Producción :
The index is 0
The index is 1
The index is 2
The index is 3
The index is 4
The index is 5
The index is 6
The index is 7
The index is 8
The index is 9