How to Save and Load NumPy Array in Python
-
Save and Load NumPy Array With the
numpy.savetxt()
andnumpy.loadtxt()
Functions -
Save and Load NumPy Array With the
numpy.tofile()
andnumpy.fromfile()
Functions -
Save and Load NumPy Array With the
numpy.save()
andnumpy.load()
Functions in Python
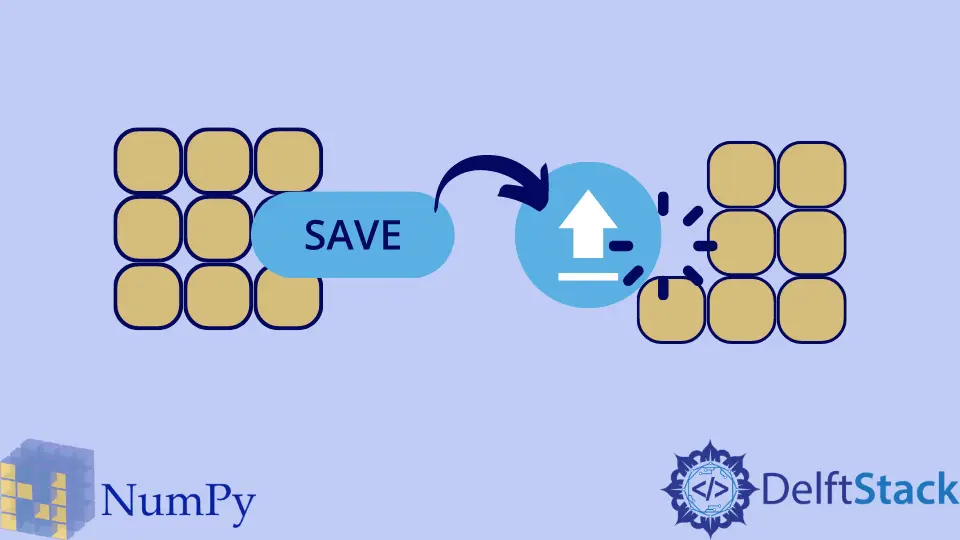
This tutorial will discuss the methods to save and load a NumPy array in Python.
Save and Load NumPy Array With the numpy.savetxt()
and numpy.loadtxt()
Functions
The numpy.savetxt()
function saves a NumPy array to a text file and the numpy.loadtxt()
function loads a NumPy array from a text file in Python. The numpy.save()
function takes the name of the text file, the array to be saved, and the desired format as input parameters and saves the array inside the text file. The numpy.loadtxt()
function takes the name of the text file and the data type of the array and returns the saved array. The following code example shows us how we can save and load a NumPy array with the numpy.savetxt()
and numpy.loadtxt()
functions in Python.
import numpy as np
a = np.array([1, 3, 5, 7])
np.savetxt("test1.txt", a, fmt="%d")
a2 = np.loadtxt("test1.txt", dtype=int)
print(a == a2)
Output:
[ True True True True]
In the above code, we saved the array a
inside the test1.txt
file with the numpy.savetxt()
function and loaded the array a2
from the test1.txt
file with the numpy.loadtxt()
function in Python. We first created the array a
with the np.array()
function. We then saved the array a
inside the test1.txt
file with the np.savetxt()
function and specified the format to be %d
, which is the integer format. We then loaded the saved array inside the array a2
with the np.loadtxt()
function and specified the dtype=int
. In the end, we compared both arrays and displayed the results.
This method is considerably slower than all the other methods discussed here.
Save and Load NumPy Array With the numpy.tofile()
and numpy.fromfile()
Functions
The numpy.tofile()
function saves a NumPy array in a binary file and the numpy.fromfile()
function loads a NumPy array from a binary file. The numpy.tofile()
function takes the name of the file as an input argument and saves the calling array inside the file in a binary format. The numpy.fromfile()
function takes the name of the file, and the data type of the array as input parameters and returns the array. The following code example shows us how to save and load a NumPy array with the numpy.tofile()
and numpy.fromfile()
functions in Python.
import numpy as np
a = np.array([1, 3, 5, 7])
a.tofile("test2.dat")
a2 = np.fromfile("test2.dat", dtype=int)
print(a == a2)
Output:
[ True True True True]
In the above code, we saved the array a
inside the test2.dat
file with the numpy.tofile()
function and loaded the array a2
from the test2.dat
file with the numpy.fromfile()
function in Python. We first created the array a
with the np.array()
function. We then saved the array a
inside the test2.dat
file with the np.tofile()
function. We then loaded the saved array inside the array a2
with the np.fromfile()
function and specified the dtype=int
. In the end, we compared both arrays and displayed the results.
This method is faster and more efficient than the previous method, but it is platform-dependent.
Save and Load NumPy Array With the numpy.save()
and numpy.load()
Functions in Python
This approach is a platform-independent way of saving and loading a NumPy array in Python. The numpy.save()
function saves a NumPy array to a file, and the numpy.load()
function loads a NumPy array from a file. We need to specify the .npy
extension for the files in this method. The numpy.save()
function takes the name of the file and the array to be saved as input parameters and saves the array inside the specified file. The numpy.load()
function takes the name of the file as an input parameter and returns the array. The following code example shows us how we can save and load a NumPy array with the numpy.save()
and numpy.load()
functions in Python.
import numpy as np
a = np.array([1, 3, 5, 7])
np.save("test3.npy", a)
a2 = np.load("test3.npy")
print(a == a2)
Output:
[ True True True True]
In the above code, we saved the array a
inside the test3.npy
file with the numpy.save()
function and loaded the array a2
from the test3.npy
file with the numpy.load()
function in Python. We first created the array a
with the np.array()
function. We then saved the array a
inside the test3.npy
file with the np.save()
function. We then loaded the saved array inside the array a2
with the np.load()
function. In the end, we compared both arrays and displayed the results.
This method is the best one so far because it is very efficient and platform-independent.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn