Element-Wise Division in Python NumPy
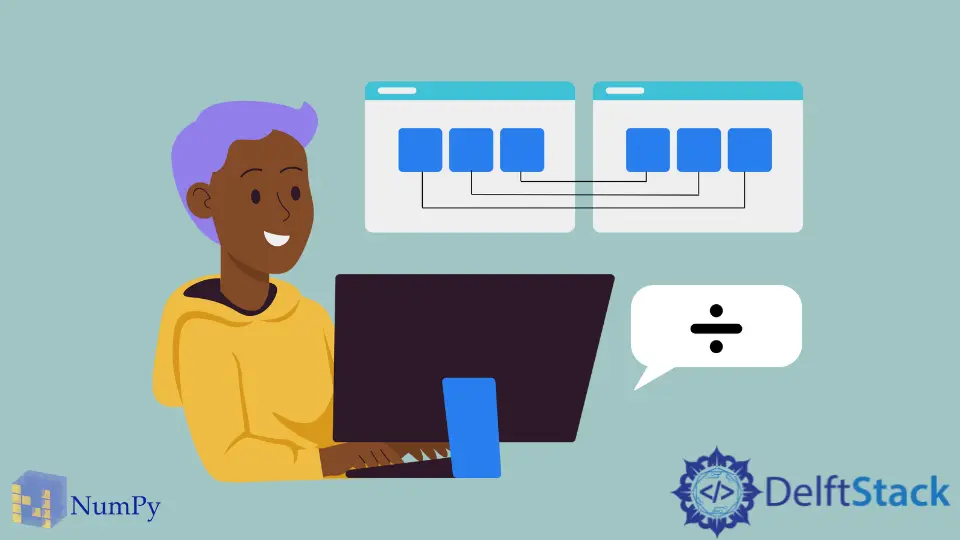
When working with numerical data in Python, especially in scientific computing and data analysis, element-wise operations are crucial. One common operation is element-wise division, which allows you to divide corresponding elements of two arrays. In Python, the NumPy library provides excellent tools for this purpose. There are two primary methods to perform element-wise division: using the numpy.divide()
function and the /
operator. Both methods are efficient and easy to use, making them popular among data scientists and analysts.
In this article, we will explore these methods in detail, providing clear examples and explanations to help you understand how to implement element-wise division in your own projects.
Using numpy.divide()
The numpy.divide()
function is a powerful way to carry out element-wise division between two NumPy arrays. This function takes two arrays as inputs and returns a new array containing the results of the division. It works seamlessly with arrays of the same shape, and it can also handle broadcasting, which allows for operations between arrays of different shapes under certain conditions.
Here’s a simple example to illustrate how to use numpy.divide()
:
import numpy as np
array1 = np.array([10, 20, 30])
array2 = np.array([2, 4, 5])
result = np.divide(array1, array2)
print(result)
Output:
[ 5. 5. 6.]
In this example, we first import the NumPy library. We then create two arrays, array1
and array2
. The numpy.divide()
function is called with these two arrays as arguments, and the result is stored in the variable result
. When we print result
, we see an array containing the results of dividing each corresponding element in array1
by the respective element in array2
. This method is particularly useful when you want more control over the division operation, such as handling errors or specifying a different output array.
Using the / Operator
Another way to perform element-wise division in NumPy is by using the /
operator. This method is often more intuitive and concise, allowing for quick calculations without the need for additional function calls. Just like numpy.divide()
, the /
operator can handle arrays of the same shape and supports broadcasting.
Here’s how you can use the /
operator for element-wise division:
import numpy as np
array1 = np.array([10, 20, 30])
array2 = np.array([2, 4, 5])
result = array1 / array2
print(result)
Output:
[ 5. 5. 6.]
In this example, we again import the NumPy library and create two arrays, array1
and array2
. Instead of using the numpy.divide()
function, we directly use the /
operator to divide array1
by array2
. The result is stored in the variable result
, which we then print. The output is the same as before, demonstrating that both methods yield identical results. The /
operator is often favored for its simplicity and readability, making it a popular choice for quick calculations in data analysis.
Conclusion
Element-wise division in Python using NumPy is a straightforward yet powerful operation that can greatly enhance your data analysis capabilities. Whether you choose to use the numpy.divide()
function or the /
operator, both methods provide efficient ways to manipulate arrays and perform calculations. By understanding these techniques, you can streamline your data processing tasks and improve your overall productivity in Python programming.
FAQ
-
What is element-wise division in NumPy?
Element-wise division in NumPy refers to the operation of dividing corresponding elements of two arrays, resulting in a new array of the same shape. -
Can I divide arrays of different shapes?
Yes, NumPy supports broadcasting, which allows you to perform element-wise division on arrays of different shapes under certain conditions. -
What is the difference between numpy.divide() and the / operator?
Both methods perform the same operation, butnumpy.divide()
offers more control and options, while the/
operator is more concise and easier to read. -
How does broadcasting work in NumPy?
Broadcasting allows NumPy to perform operations on arrays of different shapes by automatically expanding the smaller array to match the shape of the larger array. -
Are there any performance differences between the two methods?
In general, both methods are highly optimized in NumPy, and performance differences are negligible for most applications. Choose the method that best suits your coding style and needs.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn