How to Calculate Euclidean Distance in Python
- Use the NumPy Module to Find the Euclidean Distance Between Two Points
-
Use the
distance.euclidean()
Function to Find the Euclidean Distance Between Two Points -
Use the
math.dist()
Function to Find the Euclidean Distance Between Two Points
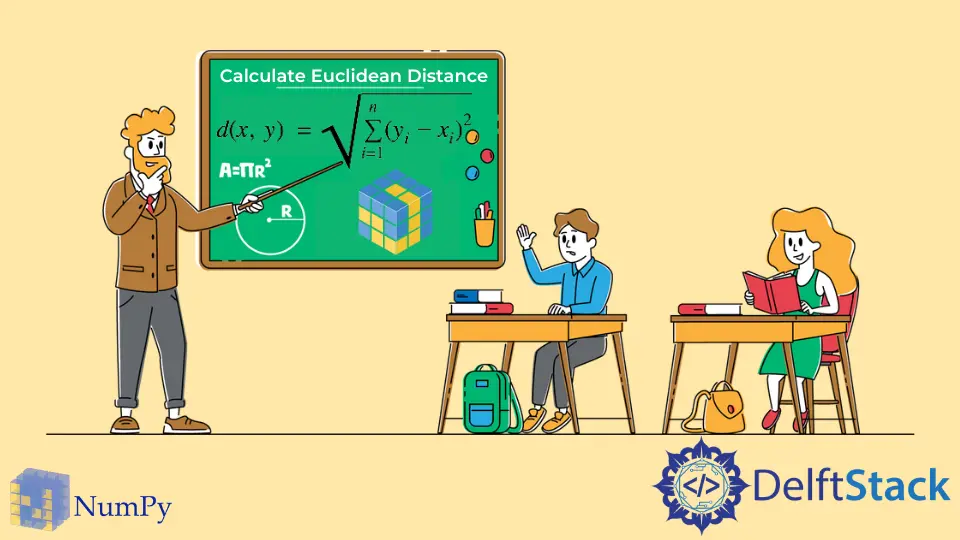
In the world of mathematics, the shortest distance between two points in any dimension is termed the Euclidean distance. It is the square root of the sum of squares of the difference between two points.
In Python, the numpy, scipy modules are very well equipped with functions to perform mathematical operations and calculate this line segment between two points.
In this tutorial, we will discuss different methods to calculate the Euclidean distance between coordinates.
Use the NumPy Module to Find the Euclidean Distance Between Two Points
The numpy module can be used to find the required distance when the coordinates are in the form of an array. It has the norm()
function, which can return the vector norm of an array. It can help in calculating the Euclidean Distance between two coordinates, as shown below.
import numpy as np
a = np.array((1, 2, 3))
b = np.array((4, 5, 6))
dist = np.linalg.norm(a - b)
print(dist)
Output:
5.196152422706632
We can also directly implement the mathematical formula using the numpy module. For this method, we will use the numpy.sum()
function, which returns the sum of elements, and the numpy.square()
function will return the square of the elements.
import numpy as np
a = np.array((1, 2, 3))
b = np.array((4, 5, 6))
dist = np.sqrt(np.sum(np.square(a - b)))
print(dist)
Output:
5.196152422706632
The numpy.sqrt()
function provides the square root of the value.
Another way of implementing the Euclidean Distance formula is using the dot()
function. We can find the dot product of the difference of points and its transpose, returning the sum of squares.
For example,
import numpy as np
a = np.array((1, 2, 3))
b = np.array((4, 5, 6))
temp = a - b
dist = np.sqrt(np.dot(temp.T, temp))
print(dist)
Output:
5.196152422706632
Use the distance.euclidean()
Function to Find the Euclidean Distance Between Two Points
We discussed different methods to calculate the Euclidean Distance using the numpy module. However, these methods can be a little slow so we have a faster alternative available.
The scipy library has many functions for mathematical and scientific calculation. The distance.euclidean()
function returns the Euclidean Distance between two points.
For example,
from scipy.spatial import distance
a = (1, 2, 3)
b = (4, 5, 6)
print(distance.euclidean(a, b))
Output:
5.196152422706632
Use the math.dist()
Function to Find the Euclidean Distance Between Two Points
The math
module also can be used as an alternative. The dist()
function from this module can return the line segment between two points.
For example,
from math import dist
a = (1, 2, 3)
b = (4, 5, 6)
print(dist(a, b))
Output:
5.196152422706632
The scipy
and math
module methods are a faster alternative to the numpy methods and work when the coordinates are in the form of a tuple or a list.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn