Python Numpy.square() - Square
-
Syntax of
numpy.square()
-
Example Codes:
numpy.square()
-
Example Codes:
numpy.square()
With theout
Parameter -
Example Codes:
numpy.square()
With Negative Numbers -
Example Codes:
numpy.square()
With Complex Numbers
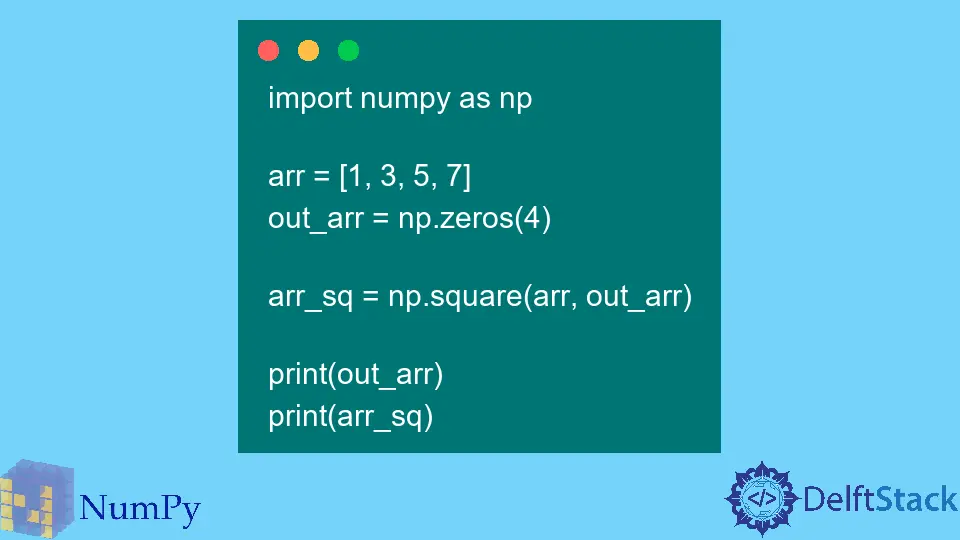
Numpy.square()
function calculates the square of every element in the given array.
It is the inverse operation of Numpy.sqrt()
method.
Syntax of numpy.square()
numpy.square(arr, out=None)
Parameters
arr |
input array |
out |
If out is given, the result will be stored in out . out should have the same shape as arr . |
Return
It returns an array of the square of each element in the input array, even if out
is given.
Example Codes: numpy.square()
import numpy as np
arr = [1, 3, 5, 7]
arr_sq = np.square(arr)
print(arr_sq)
Output:
[ 1 9 25 49]
Example Codes: numpy.square()
With the out
Parameter
import numpy as np
arr = [1, 3, 5, 7]
out_arr = np.zeros(4)
arr_sq = np.square(arr, out_arr)
print(out_arr)
print(arr_sq)
Output:
[ 1 9 25 49]
[ 1 9 25 49]
out_arr
has the same shape as arr
, and the square of arr
is saved in it.
And the numpy.square()
method also returns the square array, as shown above.
If out
doesn’t have the same shape as arr
, it raises a ValueError
.
import numpy as np
arr = [1, 3, 5, 7]
out_arr = np.zeros(3)
arr_sq = np.square(arr, out_arr)
print(out_arr)
print(arr_sq)
Output:
Traceback (most recent call last):
File "C:\Test\test.py", line 6, in <module>
arr_sq = np.square(arr, out_arr)
ValueError: operands could not be broadcast together with shapes (4,) (3,)
Example Codes: numpy.square()
With Negative Numbers
import numpy as np
arr = [-1, -3, -5, -7]
arr_sq = np.square(arr)
print(arr_sq)
Output:
[ 1 9 25 49]
Example Codes: numpy.square()
With Complex Numbers
import numpy as np
arr = [1 + 2j, -2 - 1j, 2 - 3j, -3 + 4j]
arr_sq = np.square(arr)
print(arr_sq)
Output:
[-3. +4.j 3. +4.j -5.-12.j -7.-24.j]
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook