NumPy Padding
-
Use the
NumPy.pad()
Function to Pad aNumPy
Array in Python -
Use the
shape()
Function to Pad aNumPy
Array in Python
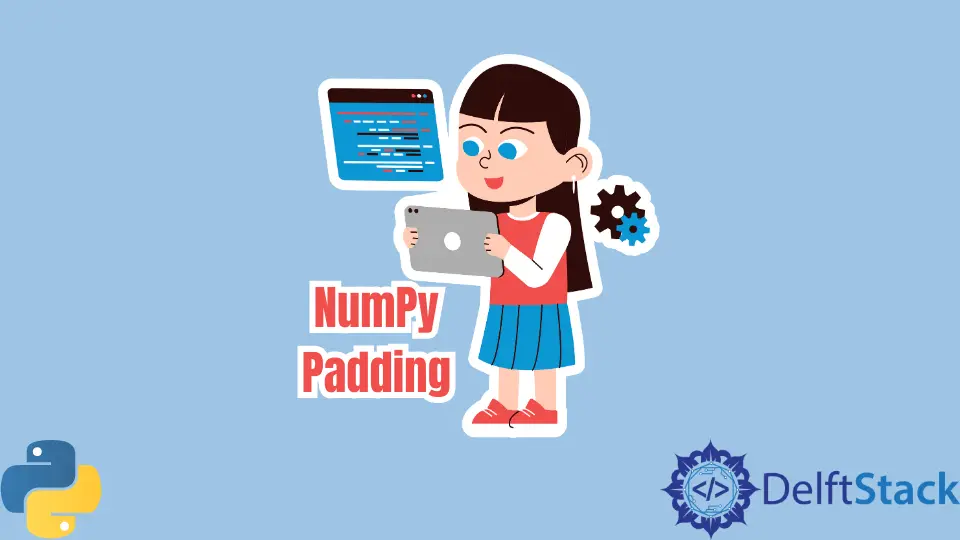
Python does not allow the direct use of arrays. This is where the NumPy
library comes in, making it possible to deal with and manipulate arrays in Python.
Arrays can be of any specified size and dimensions. Sometimes, there is a need to compensate for the dimensions of any particular array, and this is where padding comes in handy.
Padding, in simple terms, refers to adding uninformative values, usually zeros, to any rows or columns when talking about arrays. It is vastly utilized for compensating for the sheer number of rows or columns in a lacking array or a matrix.
This tutorial demonstrates how to pad a NumPy
array in Python. For example, we will pad the given NumPy
array with zeros in this tutorial.
Use the NumPy.pad()
Function to Pad a NumPy
Array in Python
As the name suggests, the NumPy.pad()
function is utilized to perform the padding operation on NumPy
arrays.
The syntax of the NumPy.pad()
function is as follows.
numpy.pad(array, pad_width, mode="constant", **kwargs)
All the parameters of the NumPy.pad()
function have been defined below for ease of understanding of the reader.
-
array
- The parameter specifies the array that must be padded. -
pad_width
- It specifies the number of values that would be added to the edges of all the axes. Tuples are used to specify the width of the multidimensional arrays. -
mode
- An optional parameter specifies the array’s mode. -
**kwargs
- Can pass variable keyword length of argument inside a function. It is optional to mention, and you can read more about it online.The examples in this article do not feature the use of this argument.
Here, we will take an example of a multidimensional array, but the same can be done for a one-dimensional array by tweaking the code a little bit.
The following code uses the NumPy.pad()
function to pad a NumPy
array in Python.
import numpy as np
x = np.array([[1.0, 1.0, 1.0, 1.0], [1.0, 1.0, 1.0, 1.0], [1.0, 1.0, 1.0, 1.0]])
y = np.pad(x, [(0, 1), (0, 1)], mode="constant")
print(y)
The above code provides the following output.
[[1. 1. 1. 1. 0.]
[1. 1. 1. 1. 0.]
[1. 1. 1. 1. 0.]
[0. 0. 0. 0. 0.]]
Here, we perform the padding operation on a multidimensional array to extend its dimensions to our specified size.
Use the shape()
Function to Pad a NumPy
Array in Python
This is an indirect method that is also capable of achieving the same results as the NumPy.pad()
function. The shape()
function is another one of the functions contained within the NumPy
library and can be accessed after importing this library to the code.
The shape
function is utilized to determine the dimensions of a given array or a matrix.
This method is a bit stretched, as it creates a fresh null matrix of the desired dimensions per the user’s needs and then inserts the original matrix into the newly created null matrix. It achieves the same results but takes a different and indirect path in the journey to get to the result.
Apart from the shape()
function, this approach uses the NumPy.zeros()
method to create a null matrix.
For this approach, we need a reference matrix whose dimensions satisfy the need of the user’s target dimensions post padding, as we take the reference of the final dimensions needed to create the null matrix first.
The following code uses the shape()
function to pad a NumPy
array in Python.
import numpy as np
x = np.array([[1.0, 1.0, 1.0, 1.0], [1.0, 1.0, 1.0, 1.0], [1.0, 1.0, 1.0, 1.0]])
y = np.array(
[
[1.0, 1.0, 1.0, 1.0, 1.0],
[1.0, 1.0, 1.0, 1.0, 1.0],
[1.0, 1.0, 1.0, 1.0, 1.0],
[1.0, 1.0, 1.0, 1.0, 1.0],
]
)
z = np.zeros(np.shape(y))
z[: x.shape[0], : x.shape[1]] = x
print(z)
The above code provides the following output.
[[1. 1. 1. 1. 0.]
[1. 1. 1. 1. 0.]
[1. 1. 1. 1. 0.]
[0. 0. 0. 0. 0.]]
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn