Python Numpy.pad Function
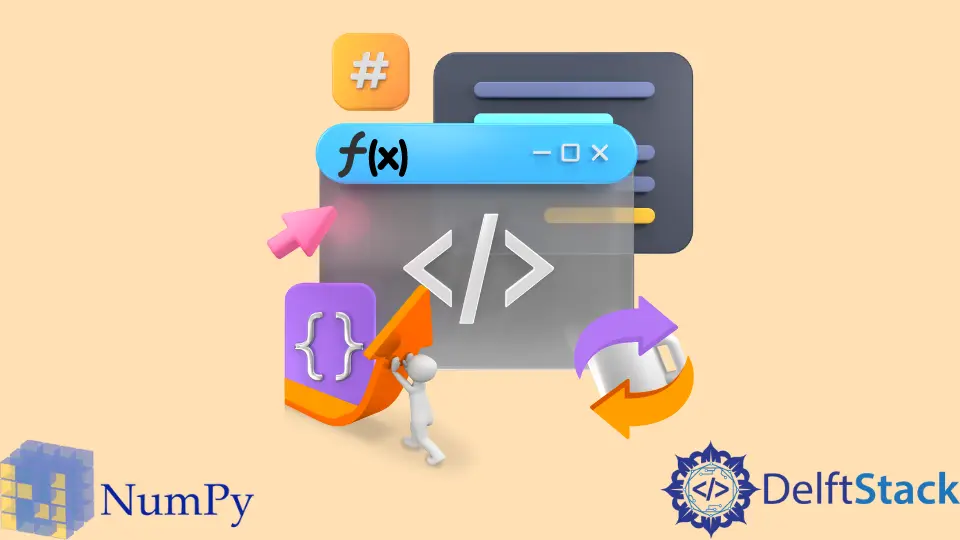
In Python, we have the NumPy
module to create and work with arrays. Arrays can be of different sizes and dimensions. Padding is a useful method available to compensate for the size of an array. We can alter the array and add some padded values to change its shape and size.
We can have other methods also to reshape an array. Still, this function is beneficial because it automatically resizes the array’s memory after using it.
The numpy.pad()
function is used to achieve this. The following code shows an example of this function.
import numpy as np
a = [1, 2, 3, 4]
b = np.pad(a, (3, 2), mode="constant", constant_values=(0, 5))
print(b)
Output:
[0 0 0 1 2 3 4 5 5]
In the above example, the first argument, a (3,2)
tuple, specifies that the 3 elements are added before the axis, and 2 elements are added at the end of the axis.
The mode
parameter specifies what type of value is to be used in padding the array. In our code, we use constant values 0 and 5 to pad the array, but we can alter this mode to different types like median
, mean
, empty
, wrap
, and more. Each mode provides different elements to pad the array with.
We can also use this function with multi-dimensional arrays. For example,
import numpy as np
a = np.array(
[[1.0, 1.0, 1.0, 1.0, 1.0], [1.0, 1.0, 1.0, 1.0, 1.0], [1.0, 1.0, 1.0, 1.0, 1.0]]
)
b = np.pad(a, [(0, 1), (0, 1)], mode="constant")
print(b)
Output:
[[1. 1. 1. 1. 1. 0.]
[1. 1. 1. 1. 1. 0.]
[1. 1. 1. 1. 1. 0.]
[0. 0. 0. 0. 0. 0.]]
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn