How to Return Query Based on Date in MongoDB
- Query with Date Range in MongoDB
- Find Documents Between Two Dates in MongoDB
- Find Documents After a Specific Date in MongoDB
- Find Documents Before a Specific Date in MongoDB
- Return the Query Based on the Date in MongoDB
- Conclusion
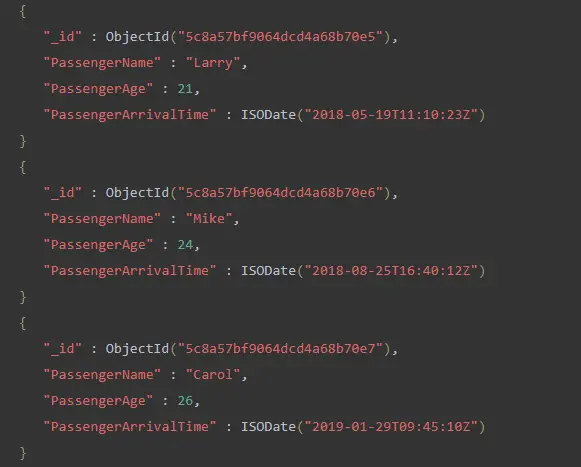
In this MongoDB tutorial article, the problem of returning the query based on date is pointed out. Moreover, how to query with a Date Range in MongoDB is explained in detail.
Query with Date Range in MongoDB
This section will show how to write a MongoDB date range query to get records based on a timestamp. Examples for MongoDB date query include MongoDB date greater than or less than time or date, etc.
Syntax:
db.collection.find({
day: {
$gt: ISODate("2020-01-21"),
$lt: ISODate("2020-01-24")
}
})
This query returns all documents in the collection where the day
field is greater than 2020-01-21
and less than 2020-01-24
.
The instructions shown above are straightforward to comprehend. However, there are minor differences between these instructions.
You can use $gte
for greater than or equal to
.
gte = greater than or equal to i.e >=
$gt
indicates greater than
.
gt = greater than i.e >
$lte
is used for less than or equal
.
lte = less than or equal to i.e <=
$lt
indicates less than
.
lt = less than i.e <
The information below shows how you can use this syntax in practice with a collection data with the following documents:
db.data.insertOne({day: new Date("2022-01-20"), amount: 40})
db.data.insertOne({day: new Date("2022-01-21"), amount: 32})
db.data.insertOne({day: new Date("2022-01-22"), amount: 19})
db.data.insertOne({day: new Date("2022-01-23"), amount: 29})
db.data.insertOne({day: new Date("2022-01-24"), amount: 35})
Find Documents Between Two Dates in MongoDB
You can utilize the query given below to find all documents where the day
field is between two specific dates:
db.data.find({
day: {
$gt: ISODate("2020-01-21"),
$lt: ISODate("2020-01-24")
}
})
Output:
{ _id: ObjectId("618548bc7529c93ea0b41490"),
day: 2020-01-22T00:00:00.000Z,
amount: 19 }
{ _id: ObjectId("618548bc7529c93ea0b41491"),
day: 2020-01-23T00:00:00.000Z,
amount: 29 }
Find Documents After a Specific Date in MongoDB
You can use the following query given below to find all documents where the day
field is after a specific date:
db.data.find({
day: {
$gt: ISODate("2020-01-22")
}
})
Output:
{ _id: ObjectId("618548bc7529c93ea0b41491"),
day: 2020-01-23T00:00:00.000Z,
amount: 29 }
{ _id: ObjectId("618548bc7529c93ea0b41492"),
day: 2020-01-24T00:00:00.000Z,
amount: 35 }
Find Documents Before a Specific Date in MongoDB
You can use the following query given below to find all documents where the day
field is before a specific date:
db.data.find({
day: {
$lt: ISODate("2020-01-22")
}
})
Output:
{ _id: ObjectId("618548bc7529c93ea0b4148e"),
day: 2020-01-20T00:00:00.000Z,
amount: 40 }
{ _id: ObjectId("618548bc7529c93ea0b4148f"),
day: 2020-01-21T00:00:00.000Z,
amount: 32 }
Return the Query Based on the Date in MongoDB
Let’s watch an example of how to return a query in MongoDB depending on the date.
Let’s make a collection called data
using the document to better grasp the notion. The following is the query to build a collection containing a record:
db.data.insertOne({"PassengerName":"John","PassengerAge":23,"PassengerArrivalTime":new ISODate("2018-03-10 14:45:56")});
{
"acknowledged" : true,
"insertedId" : ObjectId("5c8a57be9064dcd4a68b70e4")
}
db.data.insertOne({"PassengerName":"Larry","PassengerAge":21,"PassengerArrivalTime":new ISODate("2018-05-19 11:10:23")});
{
"acknowledged" : true,
"insertedId" : ObjectId("5c8a57bf9064dcd4a68b70e5")
}
db.data.insertOne({"PassengerName":"Mike","PassengerAge":24,"PassengerArrivalTime":new ISODate("2018-08-25 16:40:12")});
{
"acknowledged" : true,
"insertedId" : ObjectId("5c8a57bf9064dcd4a68b70e6")
}
db.data.insertOne({"PassengerName":"Carol","PassengerAge":26,"PassengerArrivalTime":new ISODate("2019-01-29 09:45:10")});
{
"acknowledged" : true,
"insertedId" : ObjectId("5c8a57bf9064dcd4a68b70e7")
}
This will display all the documents from a collection with the help of the find()
method. The query for this is given below:
db.data queryFromDate.find().pretty();
Output:
Here is the return query based on the date. Records having a date after 2018-05-19T11:10:23Z
will be shown as,
> db.data queryFromDate.find({"PassengerArrivalTime" : { $gte : new ISODate("2018-05-19T11:10:23Z") }}).pretty();
Output:
Conclusion
Through the help of this article, you have gained information about using the Date()
method. Moreover, the $gte
and $lte
commands are explained with examples.
Returning a query based on the date is also illustrated with code snippets.