How to Query for Documents With Array Size Greater Than 1 in MongoDB
-
Query in MongoDB Array Size Greater Than 1 Using
$where
Operator -
Use
$expr
and$gt
Operators to Query for Documents With Array Size Greater Than 1 in MongoDB -
Use
$exists
and$ne
Operators to Query for Documents With Array Size Greater Than 1 in MongoDB - Use Aggregation Framework to Query for Documents With Array Size Greater Than 1 in MongoDB
- Use Dot Notation to Query for Documents With Array Size Greater Than 1 in MongoDB
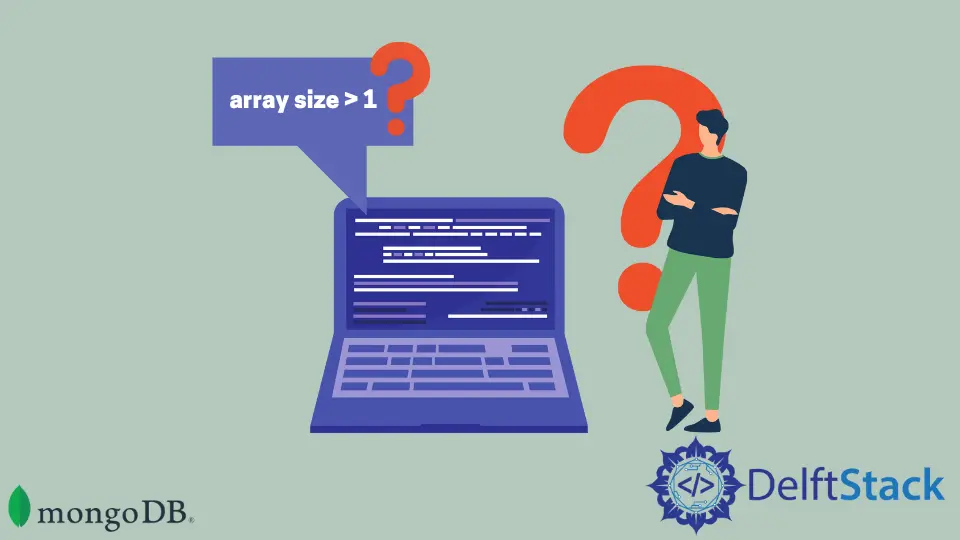
MongoDB, a NoSQL
database, is known for its flexibility in handling complex data structures, including arrays. Arrays are a versatile data type in MongoDB, often used to store lists of values within a document.
When working with arrays in MongoDB, you might encounter scenarios where you need to query for documents based on the size of an array. In this article, we will explore different methods to achieve this by using MongoDB queries.
All the examples in this article will use the below sample data to query for documents with a particular array size in MongoDB.
db.collection.insertMany([
{
_id: 1,
title: "The Art of Programming",
authors: ["John Doe", "Jane Smith"]
},
{
_id: 2,
title: "The Art of Moving On",
authors: ["Juan dela Cruz"]
}
]);
Query in MongoDB Array Size Greater Than 1 Using $where
Operator
The $where
operator in MongoDB allows you to write JavaScript expressions as part of your query. This flexibility opens up possibilities for complex queries, enabling you to incorporate custom logic that goes beyond the standard query operators.
You can leverage this to check the length of the array within the expression.
db.collection.find({
$where: "this.arrayField.length > 1"
})
In this syntax, collection
is the name of your MongoDB collection, and arrayField
is the field containing the array.
The $where
operator evaluates the provided JavaScript expression for each document in the collection. The expression checks if the length of the arrayField
is greater than 1.
The following query retrieves documents where the authors
array field has a size greater than 1:
db.collection.find({
$where: "this.authors.length > 1"
})
Output:
Use $expr
and $gt
Operators to Query for Documents With Array Size Greater Than 1 in MongoDB
The $expr
operator in MongoDB is part of the aggregation framework and allows you to use aggregation expressions within your queries. It’s particularly useful when you need to compare fields within the same document, making it well-suited for array size comparisons.
Combining it with the $gt
(greater than) operator, you can compare the size of the array directly.
Let’s start by examining the basic structure of a query using $expr
and $gt
to filter documents based on the array size.
db.collection.find({
$expr: { $gt: [{ $size: "$arrayField" }, 1] }
})
The $expr
operator allows you to use aggregation expressions within the query. $size
returns the number of elements in the specified array (arrayField
), and $gt
checks if the size is greater than 1.
This query retrieves documents where the length of the authors
is greater than 1.
db.collection.find({
$expr: { $gt: [{ $size: "$authors" }, 1] }
})
Output (based on the sample data):
Use $exists
and $ne
Operators to Query for Documents With Array Size Greater Than 1 in MongoDB
The $exists
operator in MongoDB checks for the existence of a field, while the $ne
operator compares a field to a specified value, returning documents where the field is not equal to that value. Combining these operators allows us to filter documents based on the presence of an array and its size.
You can use the $exists
operator along with the $ne
(not equal) operator to find documents where the array exists and its size is not equal to 1.
Let’s delve into the structure of a MongoDB query using $exists
and $ne
to filter documents based on array size.
db.collection.find({
arrayField: { $exists: true, $not: { $size: 1 } }
})
In this query, we check if arrayField
exists ($exists: true
) and its size is not equal to 1 ($not: { $size: 1 }
), effectively filtering documents with an array size greater than 1.
This query retrieves documents where authors
exists and its size is greater than 1.
db.collection.find({
authors: { $exists: true, $not: { $size: 1 } }
})
Output (based on the sample data):
Use Aggregation Framework to Query for Documents With Array Size Greater Than 1 in MongoDB
The aggregation framework in MongoDB is a pipeline-based system that allows for the processing of documents through a series of stages.
Each stage performs a specific operation, and the result of one stage becomes the input for the next. This framework is particularly powerful for tasks such as filtering, grouping, sorting, and projecting data.
You can use the $project
stage to create a new field representing the array size and then filter based on that field.
db.collection.aggregate([
{
$project: {
documentSize: { $size: "$arrayField" },
otherFields: 1 // Include other fields as needed
}
},
{
$match: {
documentSize: { $gt: 1 }
}
}
])
In this example, the $project
stage creates a new field called documentSize
representing the size of the arrayField
. The $match
stage then filters documents where documentSize
is greater than 1.
This query retrieves documents where the length of the authors
field is greater than 1.
db.collection.aggregate([
{
$project: {
documentSize: { $size: "$authors" },
otherFields: 1 // Include other fields as needed
}
},
{
$match: {
documentSize: { $gt: 1 }
}
}
])
Output (based on the sample data):
Use Dot Notation to Query for Documents With Array Size Greater Than 1 in MongoDB
Dot notation is a MongoDB feature that allows you to access array elements and fields within nested documents using a dot (.
) to separate the levels. It simplifies the process of querying and updating nested structures, providing a clean and intuitive syntax.
Let’s examine the structure of a MongoDB query using dot notation to select documents based on array size.
db.collection.find({
"arrayField.1": { $exists: true }
})
In this syntax, the collection
is the name of your MongoDB collection. The "arrayField.1"
uses dot notation to check if the second element (index 1) of the arrayField
exists. The $exists: true
ensures that the specified array element exists.
This query retrieves documents where the size of the authors
is greater than 1, as it checks for the existence of the second element (index 1).
db.collection.find({
"authors.1": { $exists: true }
})
Output (based on the sample data):