How to Print Document Values Using MongoDB Shell
- Print Document Values Using MongoDB Shell
- Print the Document Values for All the Documents in a Collection
- Print the Document Values for the First Document Only
- Print the Document Values for All the Documents with Custom Property Names
- Print the Document Values Based on the Specified Conditions for All the Documents
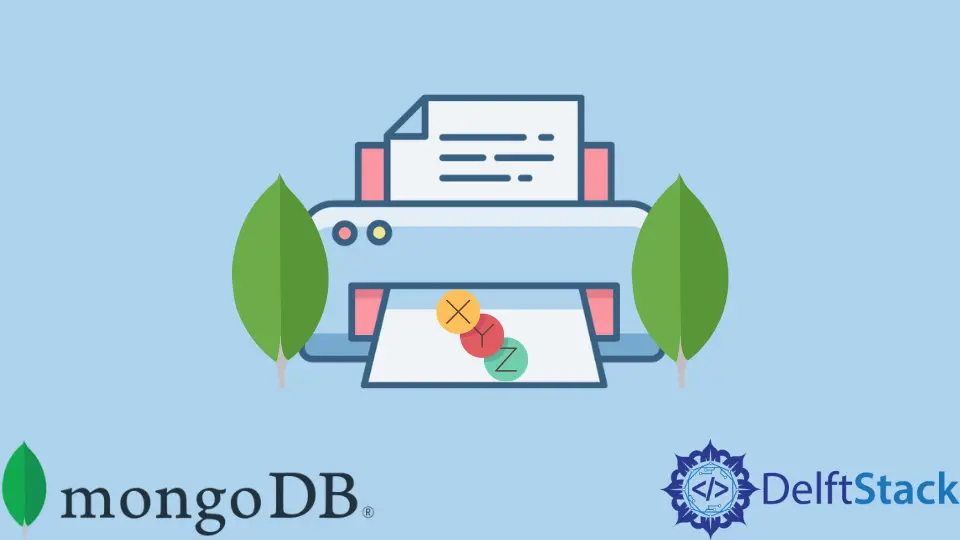
Printing the document’s value is also an incredible skill that we can benefit from depending on the project’s needs.
This tutorial prints the document values instead of printing the complete document using the MongoDB shell.
Print Document Values Using MongoDB Shell
Printing the documents using the Mongo shell is very easy, but how to print the values and properties instead of getting the whole document? Before digging into the details, let’s create a collection named teachers
containing the JSON documents as given below.
Create Collection:
> db.createCollection('teachers')
Insert the JSON Documents into the teachers
Collection:
> db.teachers.insertMany(
[
{
"first_name": "Mehvish",
"last_name": "Ashiq",
"gender": "Female",
"grade": 18
},
{
"first_name": "Tahir",
"last_name": "Raza",
"gender": "Male",
"grade": 18
},
{
"first_name": "Saira",
"last_name": "Daniel",
"gender": "Female",
"grade": 20
}
]
)
Display All Documents from teachers
Collection:
> db.teachers.find().pretty()
OUTPUT:
{
"_id" : ObjectId("6290440e7c524c650b7a51c0"),
"first_name" : "Mehvish",
"last_name" : "Ashiq",
"gender" : "Female",
"grade" : 18
}
{
"_id" : ObjectId("6290440e7c524c650b7a51c1"),
"first_name" : "Tahir",
"last_name" : "Raza",
"gender" : "Male",
"grade" : 18
}
{
"_id" : ObjectId("6290440e7c524c650b7a51c2"),
"first_name" : "Saira",
"last_name" : "Daniel",
"gender" : "Female",
"grade" : 20
}
Once we are done with creating the collection and populating it with documents, we can print the document values depending on the project requirements. Some of the scenarios are listed below:
- Print the document values for all the documents in a collection
- Print the document values for the first document only
- Print the document values for all the documents with custom property names
- Print the document values based on the specified conditions for all the documents
Print the Document Values for All the Documents in a Collection
Example Code:
> db.teachers.find().forEach(
function (data) {
Object.keys(data).forEach(
function(key) {
print(key + ': ' + data[key])
}
)
print('\n')
}
)
OUTPUT:
_id: 6290440e7c524c650b7a51c0
first_name: Mehvish
last_name: Ashiq
gender: Female
grade: 18
_id: 6290440e7c524c650b7a51c1
first_name: Tahir
last_name: Raza
gender: Male
grade: 18
_id: 6290440e7c524c650b7a51c2
first_name: Saira
last_name: Daniel
gender: Female
grade: 20
This example code gets all the JSON documents using the find()
method. Then, we iterate over each document using JavaScript’s forEach()
loop and pass every document to an anonymous function as data
.
In this function, we use Object.keys(data)
to retrieve all the keys (also called properties or field names) for that particular document contained by the data
variable.
Further, another forEach()
method is used to iterate over the keys of the document (stored in the data
variable) and call another anonymous function to print all the keys with the respective values.
Print the Document Values for the First Document Only
Example Code:
> let result = db.getCollection('teachers').findOne()
> for (var key in result) {
if (result.hasOwnProperty(key)) {
print(key + ': ' + result[key]);
}
}
OUTPUT:
_id: 6290440e7c524c650b7a51c0
first_name: Mehvish
last_name: Ashiq
gender: Female
grade: 18
For this example snippet, we use the findOne()
method to retrieve the first document only from the specified collection and save it into the result
object.
Next, we use JavaScript’s for-in
loop to iterate over the result
object and return a key
to access the value as result[key]
.
Before accessing the value for the key
, we use the hasOwnProperty()
method to check if the object (which is result
in this case) has the given property as its own.
Print the Document Values for All the Documents with Custom Property Names
Example Code:
> db.teachers.find().forEach(
function (document) {
print("FirstName: " + document.first_name +"\n"+
"LastName: " + document.last_name + "\n"+
"Grade: " + document.grade)
print("\n\n")
}
)
OUTPUT:
FirstName: Mehvish
LastName: Ashiq
Grade: 18
FirstName: Tahir
LastName: Raza
Grade: 18
FirstName: Saira
LastName: Daniel
Grade: 20
Sometimes, we do not want to get the property names as they are specified in the program, but we want to use the custom property names. If so, this solution is for you.
We get all the documents in the teachers
collection using the find()
method. Then, iterate over each document to retrieve the values using keys (also called field or property names).
You may have noticed that we are not getting the primary key named _id
and its value for this example. It is because we are not specifying it in the print()
statement, but it is still there in the document
variable.
Use the following code to check.
Example Code:
db.teachers.find().forEach(
function (document) {
print(document._id)
print("\n\n")
}
)
OUTPUT:
ObjectId("6290440e7c524c650b7a51c0")
ObjectId("6290440e7c524c650b7a51c1")
ObjectId("6290440e7c524c650b7a51c2")
Let’s see the following section to avoid it on the Mongo shell.
Print the Document Values Based on the Specified Conditions for All the Documents
Example Code:
> db.teachers.find({}, {"grade": 1, "_id":0}).forEach(
function(property) {
print(property.grade)
})
OUTPUT:
18
18
20
Here, we need to understand first the find()
function to understand the example code. We can use the find()
method in different ways to meet the requirements; some formats are listed below:
- Use
find()
orfind({})
to get all the documents from the specified collection - Use
find({}, {"city": 1})
to retrieve thecity
property with its value for all the documents. - Use
find({}, {"city": 1, "_id:0"})
to get thecity
property and its value for all documents but don’t display theObjectId
. Here, setting the value of_id
to0
(by default it is1
) does not allow to print theObjectId
.
Similarly, we use the find()
method to retrieve the grade
property and its value without displaying the _id
field.
However, we use the forEach()
loop for every record to only print the property value passed to the anonymous function. We can optimize the code using JavaScript’s arrow function.
Example Code:
db.teachers.find({}, {"grade": 1, "_id":0}).forEach( property => print(property.grade))
OUTPUT:
18
18
20