How to Print JSON Without Whitespaces in MongoDB
- MongoDB Print JSON Without Whitespaces
-
Use the
find()
Method to Print JSON Without Whitespaces in MongoDB -
Use the
cursor
Object to PrintJSON
Without Whitespaces in MongoDB
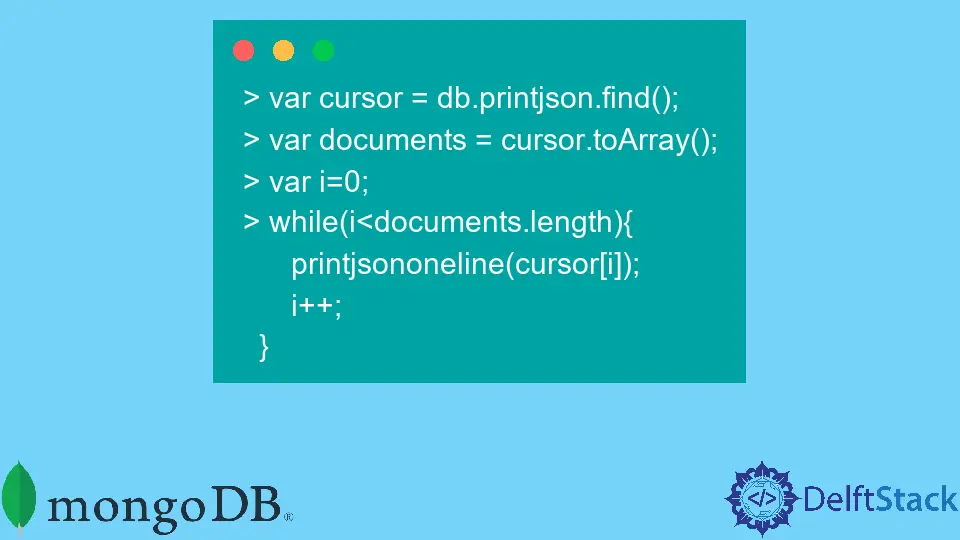
This article teaches how to print JSON documents without whitespaces in MongoDB with the help of different code snippets.
MongoDB Print JSON Without Whitespaces
Let’s create a sample collection and insert three documents to use in the code examples later in this tutorial to understand it better.
Example Code:
> db.createCollection('printjson');
> db.printjson.insertMany([
{"_id": "ab01", array: ['element1', 'element2'], results: [3,4]},
{"_id": "cd02", array: ['element1', 'element2'], results: [7,3]},
{"_id": "ef03", array: ['element1', 'element2'], results: [9,9]}
]);
> db.printjson.find().pretty();
OUTPUT:
{
"_id" : "ab01",
"array" : [
"element1",
"element2"
],
"results" : [
3,
4
]
}
{
"_id" : "cd02",
"array" : [
"element1",
"element2"
],
"results" : [
7,
3
]
}
{
"_id" : "ef03",
"array" : [
"element1",
"element2"
],
"results" : [
9,
9
]
}
As we can see, the output given above has a lot of whitespaces that we don’t want. We desire to print these resultant JSON documents without whitespaces, as given below.
{ "_id" : "ab01", "array" : [ "element1", "element2" ], "results" : [ 3, 4 ] }
{ "_id" : "cd02", "array" : [ "element1", "element2" ], "results" : [ 7, 3 ] }
{ "_id" : "ef03", "array" : [ "element1", "element2" ], "results" : [ 9, 9 ] }
Use the find()
Method to Print JSON Without Whitespaces in MongoDB
Example Code:
> db.printjson.find();
OUTPUT:
{ "_id" : "ab01", "array" : [ "element1", "element2" ], "results" : [ 3, 4 ] }
{ "_id" : "cd02", "array" : [ "element1", "element2" ], "results" : [ 7, 3 ] }
{ "_id" : "ef03", "array" : [ "element1", "element2" ], "results" : [ 9, 9 ] }
One of the simplest approach is to use the find()
method only instead of find().pretty()
.
Use the cursor
Object to Print JSON
Without Whitespaces in MongoDB
Before going to the code examples, it is necessary to know the cursor
object. Whenever we use the find()
method to retrieve documents from a particular collection, it returns a pointer that we call a cursor
.
We can say that the pointer returned by the find()
method is known as a cursor
object.
Example Code:
> var cursor = db.printjson.find();
> while(cursor.hasNext()){
printjsononeline(cursor.next());
}
OUTPUT:
{ "_id" : "ab01", "array" : [ "element1", "element2" ], "results" : [ 3, 4 ] }
{ "_id" : "cd02", "array" : [ "element1", "element2" ], "results" : [ 7, 3 ] }
{ "_id" : "ef03", "array" : [ "element1", "element2" ], "results" : [ 9, 9 ] }
In this code, we save the pointer returned by the find()
method in a variable named cursor
. Further, we iterate over the cursor
variable to print the JSON documents without whitespaces.
Here, the cursor.hasNext()
method returns true if the cursor returned by the db.printjson.find()
can iterate further to return more documents. The next()
method is being used to get access to the next document.
Instead of saving the pointer in a variable and then iterating over that variable, we can use the more optimized query given below.
Example Code:
> db.printjson.find().forEach(printjsononeline);
OUTPUT:
{ "_id" : "ab01", "array" : [ "element1", "element2" ], "results" : [ 3, 4 ] }
{ "_id" : "cd02", "array" : [ "element1", "element2" ], "results" : [ 7, 3 ] }
{ "_id" : "ef03", "array" : [ "element1", "element2" ], "results" : [ 9, 9 ] }
Next, we can iterate over the cursor
variable and print the resultant document in the array form using the toArray()
method, as shown below.
Example Code:
> var cursor = db.printjson.find();
> var documents = cursor.toArray();
> var i=0;
> while(i<documents.length){
printjsononeline(cursor[i]);
i++;
}
OUTPUT:
{ "_id" : "ab01", "array" : [ "element1", "element2" ], "results" : [ 3, 4 ] }
{ "_id" : "cd02", "array" : [ "element1", "element2" ], "results" : [ 7, 3 ] }
{ "_id" : "ef03", "array" : [ "element1", "element2" ], "results" : [ 9, 9 ] }
Alternatively, we can do the same as follows as well.
> var cursor = db.printjson.find();
> var i=0;
> while(i<cursor.count()){
printjsononeline(cursor[i]);
i++;
}
OUTPUT:
{ "_id" : "ab01", "array" : [ "element1", "element2" ], "results" : [ 3, 4 ] }
{ "_id" : "cd02", "array" : [ "element1", "element2" ], "results" : [ 7, 3 ] }
{ "_id" : "ef03", "array" : [ "element1", "element2" ], "results" : [ 9, 9 ] }