How to Get the Last N Records in MongoDB
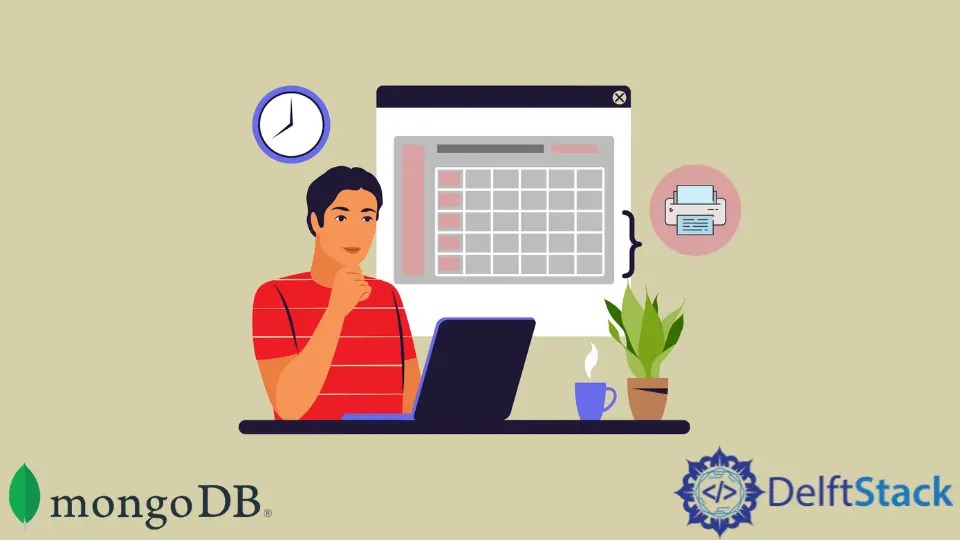
This tutorial will explore the number of ways to get the last N records in MongoDB, where N is a positive number and greater than zero. We will see how to retrieve the number of documents with and without sorting.
Get the Last N Records in MongoDB
We must have a collection containing documents to continue with the practical examples. So, let’s move ahead and use the following queries to create a collection and insert a few documents in it.
Example Code:
> use get_n_records;
> db.createCollection('stock');
> db.stock.insertMany([
{"item": "abc", "quantity": 12},
{"item": "def", "quantity": 234},
{"item": "ghi", "quantity": 45},
{"item": "jkl", "quantity": 345},
{"item": "mno", "quantity": 243},
{"item": "pqr", "quantity": 345},
{"item": "stu", "quantity": 56},
{"item": "vwx", "quantity": 575},
{"item": "yzz", "quantity": 398}
]);
> db.stock.find();
OUTPUT:
{ "_id" : ObjectId("629ac651b7e637e64d8e75fb"), "item" : "abc", "quantity" : 12 }
{ "_id" : ObjectId("629ac651b7e637e64d8e75fc"), "item" : "def", "quantity" : 234 }
{ "_id" : ObjectId("629ac651b7e637e64d8e75fd"), "item" : "ghi", "quantity" : 45 }
{ "_id" : ObjectId("629ac651b7e637e64d8e75fe"), "item" : "jkl", "quantity" : 345 }
{ "_id" : ObjectId("629ac651b7e637e64d8e75ff"), "item" : "mno", "quantity" : 243 }
{ "_id" : ObjectId("629ac651b7e637e64d8e7600"), "item" : "pqr", "quantity" : 345 }
{ "_id" : ObjectId("629ac651b7e637e64d8e7601"), "item" : "stu", "quantity" : 56 }
{ "_id" : ObjectId("629ac651b7e637e64d8e7602"), "item" : "vwx", "quantity" : 575 }
{ "_id" : ObjectId("629ac651b7e637e64d8e7603"), "item" : "yzz", "quantity" : 398 }
Now, we have our collection ready and populated. The point is to consider whether we want to get the last N records from the most recent to less recent inserted documents or vice versa.
Let’s learn each of the scenarios given below.
Get the Last N Records From Most Recent to Less Recent Inserted Documents or Vice Versa
Before continuing, take a look at the following two terms to understand them easily:
-
Most Recent to Less Recent Sorting
It means Last In First Out (descending order). The documents inserted in the last will be displayed first.
-
Less Recent to Most Recent Sorting
It means First In First Out (ascending order). The documents inserted first will be displayed first.
Use the cursor.sort()
Method to Get the Last N Records From Most Recent to Less Recent Inserted Documents or Vice Versa
Example Code (Last In First Out):
> db.stock.find().sort({_id:-1}).limit(3);
OUTPUT:
{ "_id" : ObjectId("629ac651b7e637e64d8e7603"), "item" : "yzz", "quantity" : 398 }
{ "_id" : ObjectId("629ac651b7e637e64d8e7602"), "item" : "vwx", "quantity" : 575 }
{ "_id" : ObjectId("629ac651b7e637e64d8e7601"), "item" : "stu", "quantity" : 56 }
Example Code (First In First Out):
> db.stock.find().sort({_id:1}).limit(3);
OUTPUT:
{ "_id" : ObjectId("629ac651b7e637e64d8e75fb"), "item" : "abc", "quantity" : 12 }
{ "_id" : ObjectId("629ac651b7e637e64d8e75fc"), "item" : "def", "quantity" : 234 }
{ "_id" : ObjectId("629ac651b7e637e64d8e75fd"), "item" : "ghi", "quantity" : 45 }
Here, we use the sort()
method to sort the documents from the most recent to the less recent insertion order (descending order). So, we are applying the sort()
method to the cursor before getting any documents (records) from the database.
The sort()
method has the field-value pair as {field: value}
, and we can perform a sort on a maximum of 32 keys. The documents are not stored in a specified order in a collection while using MongoDB.
That is why when we perform a sort on a particular field with duplicate values, the documents with those values may be returned in any order. To get a consistent sort, we must include at least one field containing the unique values.
The easiest way to ensure this is to have the _id
field in the sort()
method.
The limit(N)
is used to get the specified number of documents. We can omit this function if we are interested in getting all the sorted documents.
Alternatively, we can also use the query as follows without having a particular field.
Example Code:
> db.stock.find().sort({$natural:1}).limit(3);
OUTPUT:
{ "_id" : ObjectId("629ac651b7e637e64d8e75fb"), "item" : "abc", "quantity" : 12 }
{ "_id" : ObjectId("629ac651b7e637e64d8e75fc"), "item" : "def", "quantity" : 234 }
{ "_id" : ObjectId("629ac651b7e637e64d8e75fd"), "item" : "ghi", "quantity" : 45 }
The $natural
works like First In First Out and Last In First Out if their value is 1
and -1
, respectively. We can use it if we have the MongoDB version 4.4 or above.
Use the $sort
and $limit
(Aggregation) Stages to Get the Last N Records From Most Recent to Less Recent Inserted Documents or Vice Versa
Example Code (Last In First Out):
> db.stock.aggregate([
{ $sort : { _id : -1 } },
{ $limit : 4 }
]);
OUTPUT:
{ "_id" : ObjectId("629ac651b7e637e64d8e7603"), "item" : "yzz", "quantity" : 398 }
{ "_id" : ObjectId("629ac651b7e637e64d8e7602"), "item" : "vwx", "quantity" : 575 }
{ "_id" : ObjectId("629ac651b7e637e64d8e7601"), "item" : "stu", "quantity" : 56 }
{ "_id" : ObjectId("629ac651b7e637e64d8e7600"), "item" : "pqr", "quantity" : 345 }
Example Code (First In First Out):
> db.stock.aggregate([
{ $sort : { _id : 1 } },
{ $limit : 4 }
]);
OUTPUT:
{ "_id" : ObjectId("629ac651b7e637e64d8e75fb"), "item" : "abc", "quantity" : 12 }
{ "_id" : ObjectId("629ac651b7e637e64d8e75fc"), "item" : "def", "quantity" : 234 }
{ "_id" : ObjectId("629ac651b7e637e64d8e75fd"), "item" : "ghi", "quantity" : 45 }
{ "_id" : ObjectId("629ac651b7e637e64d8e75fe"), "item" : "jkl", "quantity" : 345 }
The $sort
aggregation stage is similar to the cursor.sort()
method. It is used to sort all the documents and returns them in sorted order to a pipeline.
It takes a document having the field name and respective sort order. Remember that 1
and -1
are used for ascending and descending order.
Like the cursor.sort()
method, we can use the $sort
aggregation stage to sort on a maximum of 32 keys and have at least one field (containing unique values) for consistent sort order.
The sort order is assessed from left to right if we are required to sort on multiple fields. While the $limit
takes a number that shows how many documents need to be printed on the screen; also, it has a 64-bit integer limit.
Use the skip()
and count()
Methods Together to Get the Last N Records in MongoDB
This approach is captivating because we are not sorting the data but getting a section of documents from the beginning or end, considering the project requirements.
Example Code (Get the First 3 Records Without Sorting the Documents):
> db.stock.find().limit(3);
OUTPUT:
{ "_id" : ObjectId("629ac651b7e637e64d8e75fb"), "item" : "abc", "quantity" : 12 }
{ "_id" : ObjectId("629ac651b7e637e64d8e75fc"), "item" : "def", "quantity" : 234 }
{ "_id" : ObjectId("629ac651b7e637e64d8e75fd"), "item" : "ghi", "quantity" : 45 }
Example Code (Get the Last 3 Records Without Sorting the Documents):
> db.stock.find().skip(db.stock.count() - 3)
OUTPUT:
{ "_id" : ObjectId("629ac651b7e637e64d8e7601"), "item" : "stu", "quantity" : 56 }
{ "_id" : ObjectId("629ac651b7e637e64d8e7602"), "item" : "vwx", "quantity" : 575 }
{ "_id" : ObjectId("629ac651b7e637e64d8e7603"), "item" : "yzz", "quantity" : 398 }
In this example, we use db.stock.count()
to count the total number of documents, subtract 3 from them and skip all using the skip()
method.
Remember, the number of documents that we subtracted is not skipped (in this case, it is 3). These 3 documents we will get as a result.