Difference Between deleteMany() and Remove() in MongoDB
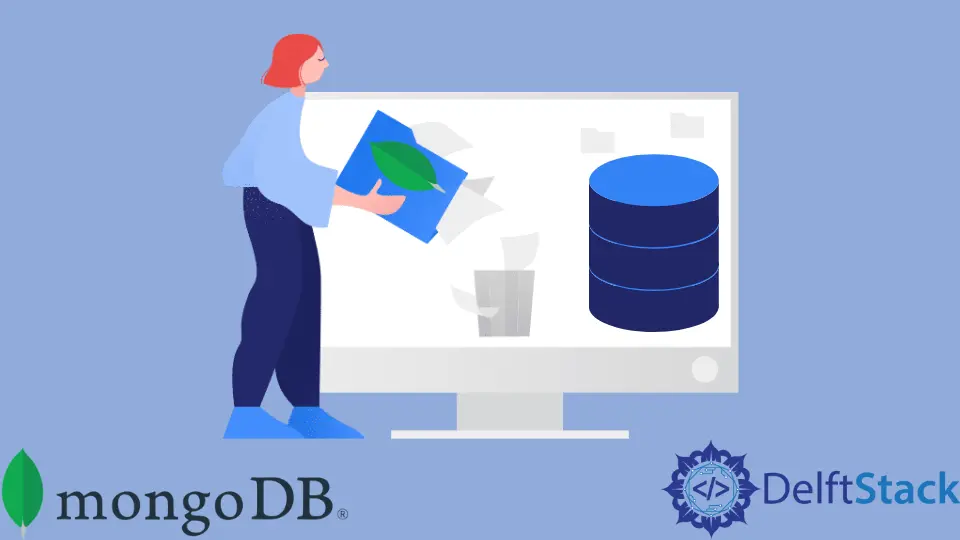
deleteMany()
and remove()
both are used for deleting entry from MongoDB. In the recent version, remove
is marked as deprecated.
Rather MongoDB suggests using deleteMany
and deleteOne
. The command deleteMany
can accept a parameter to match before deleting.
Parameter and Returns of deleteMany()
in MongoDB
This method is from the mongosh. Generally, it’s used in the collection to delete the documents under any specific collection.
Here’s how it’s used.
db.collection.deleteMany(
<FILTER>,{
writeConcern: <document>,
collation: <document>
}
)
The <FILTER>
represents that we can use our custom search field. Let’s say we want to delete all the products whose price equals 30
.
Then the code will be:
db.collection.deleteMany({
price:30
})
We can put the other parameter empty here. If you want to delete the full collection, insert {}
to the deleteMany
.
Here’s more about the writeConcern
and collation
.
Parameter | Type | Description |
---|---|---|
writeConcern |
Document | It’s an optional parameter. It puts acknowledgment for the document. To know more about writeConcern , visit this blog. |
collation |
Document | It allows the user to use language-specific rules for the document. The collation is an optional parameter. If you use it, you need to use the local field. Here’s more about the collation . |
deleteMany()
returns acknowledged
as true
if the function runs with the writeConcern
parameter; otherwise, false
. It also returns the deleteCount
, which refers to how many documents were deleted.
deleteMany
delete all the document that matches the filter.
A sample return can look like the following.
{ "acknowledged" : true, "deletedCount" : 9 }
Parameter and Returns of remove()
in MongoDB
remove()
method have two functionalities. It can also act as deleteMany
and deleteOne
.
The template code for the remove()
is here.
db.collection.remove(
<query>,
<justOne>
)
Generally, these two parameters are used. Also, you can extend this to the following.
db.collection.remove(
<query>,
{
justOne: <boolean>,
writeConcern: <document>,
collation: <document>
}
)
The writeConcern
and collation
are the same as we discussed above for the deleteMany()
. Here we can use the query
instead of a filter.
To know more about the query
in MongoDB, you can visit the following link.
If we pass an empty object in the remove
method, it will remove all the documents from the collection. For example, let’s say you have a product
collection and want to remove all the products with a price below 5.
Then the command will be:
db.products.remove(
{price:{ $lt: 5}}
)
If we put this command, the justOne
parameter will default be false
. Now, if you want to delete only one element for the same query, the command will be like the following.
db.products.remove(
{price:{ $lt: 5}}, true
)
This method returns an object called WriteResult
, which has the following parameters.
WriteResult({ "nRemoved" : 10 })
It also has writeError
and writeConcern
; these are related to writeConcern
above.
The remove()
is being deprecated in the later version of MongoDB. So, while developing the NodeJS app, you should only use the deleteOne
or deleteMany
.
To know more about remove()
, visit the following websites.