How to Delete All Records of a Collection in MongoDB Shell
- Delete the Records From a Collection in MongoDB
- Delete the First Record From a Collection in MongoDB
- Delete a Single Record From a Collection in MongoDB
- Delete Multiple Records From a Collection in MongoDB
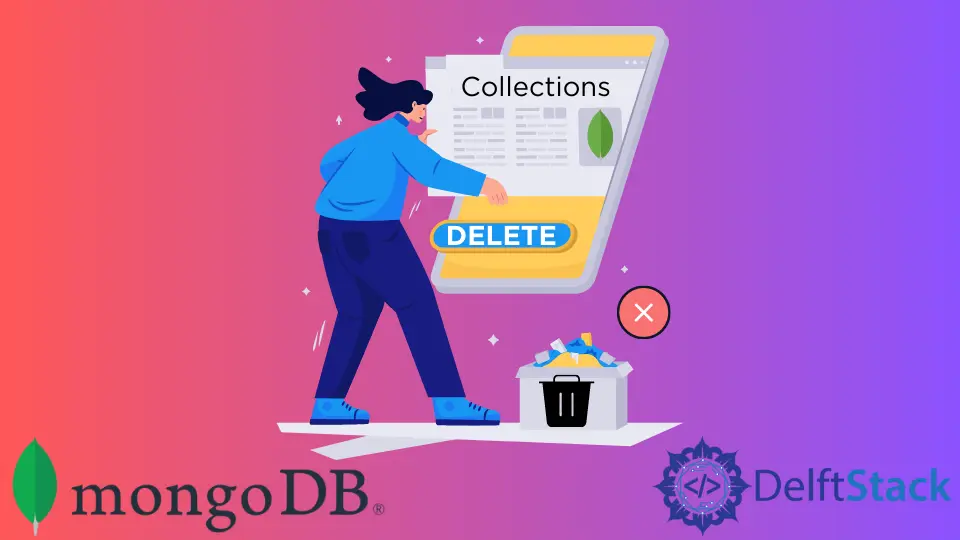
In this MongoDB article, you will learn how to delete the records in a collection using MongoDB. This will be explained using the help of a few examples of how to implement various operations.
Below is the list of the following topics covered in this tutorial.
- How to delete the records in MongoDB?
- How to delete the first record from a collection in MongoDB?
- How to delete a single record from a collection in MongoDB?
- How to delete multiple records from the collection in MongoDB?
Delete the Records From a Collection in MongoDB
To delete the records from a collection, you can use the MongoDB shell’s delete
method.
-
You can explore the following method to delete a single record.
db.collection.deleteOne()
-
You can explore the following method to delete multiple records.
db.collection.deleteMany()
Using the help of different examples, you will learn how to delete records from a collection in MongoDB through the support of this topic. So, let’s take a look at an example and then delete the collected records.
A collection named teams
was created, and the following records were inserted inside the teams
collection. This collection will be used for all the examples in this tutorial.
db={
"teams": [
{
team: "Manchester City ",
position: "1st",
points: 70
},
{
team: "Liverpool",
position: "2nd",
points: 69
},
{
team: "Chelsea",
position: "3rd",
points: 59
},
{
team: "Arsenal",
position: "4th",
points: 54
},
{
team: "Manchester United",
position: "6th",
points: 50
}
]
}
Delete the First Record From a Collection in MongoDB
An example of this method is shown below. Firstly, you will apply the query below to delete a single document using the deleteOne()
method.
db.teams.deleteOne({})
The code used above will delete the first record of the teams
collection. The image below shows the above query results once it’s been run.
The first document of the collection has been successfully deleted where the team
is Manchester City
. Thus this example teaches you how to delete a single record from the collection using the deleteone()
method.
Delete a Single Record From a Collection in MongoDB
This part of the article will teach you to delete only one document that matches a given filter. For example, the deleteOne()
method will delete only one record that matches a criterion, even if numerous records fit the supplied filter.
An example of this method is shown below. You will apply the query below to delete a single document using the deleteOne()
method.
db.teams.deleteOne( { "team": "Arsenal" } )
You have used the deleteOne()
method to delete the one document where the team
is Arsenal
. The image below shows the above query results once it’s been run.
You should be able to successfully delete all of the collected records as per the set requirement.
Delete Multiple Records From a Collection in MongoDB
You will learn how to delete all records from the collection through this topic. First, you can create criteria or filters to help you find the records you want to remove.
Then, pass a filter parameter to the deleteMany()
function to remove all records that fit the deletion criterion.
Let’s use an example to grasp this better and delete all papers that match a criterion. An example of this method is shown below.
The following records were inserted into the teams
collection.
db={
"teams": [
{
team: "Manchester",
position: "1st",
points: 70
},
{
team: "Liverpool",
position: "2nd",
points: 69
},
{
team: "Chelsea",
position: "3rd",
points: 59
},
{
team: "Arsenal",
position: "4th",
points: 54
},
{
team: "Manchester",
position: "6th",
points: 50
}
]
}
After that, you will apply the query below to delete all records that match a condition.
db.teams.deleteMany( { "team": "Manchester" } )
You can use the deleteMany()
method to delete all the records where the team
is Manchester
.
The image below shows the above query results once it’s been run.
The image above shows that the user has successfully deleted both collected records from the collection as per the set requirement where the team
is Manchester
.
So using this MongoDB article, you have learned how to delete the first record from a collection in MongoDB, delete a single record from a collection in MongoDB, and delete multiple records from the collection in MongoDB.
The article also gives you various examples for these methods to grasp this topic with a better understanding.