How to Convert a String to Date in MongoDB
- Create String or Date Data Types
- Convert a String to Date in MongoDB
-
Use
toDate
Operator -
Use the
convert
Operator -
Use the
dateFromString
Operator -
Use the
set
Operator -
Use
ClockTime
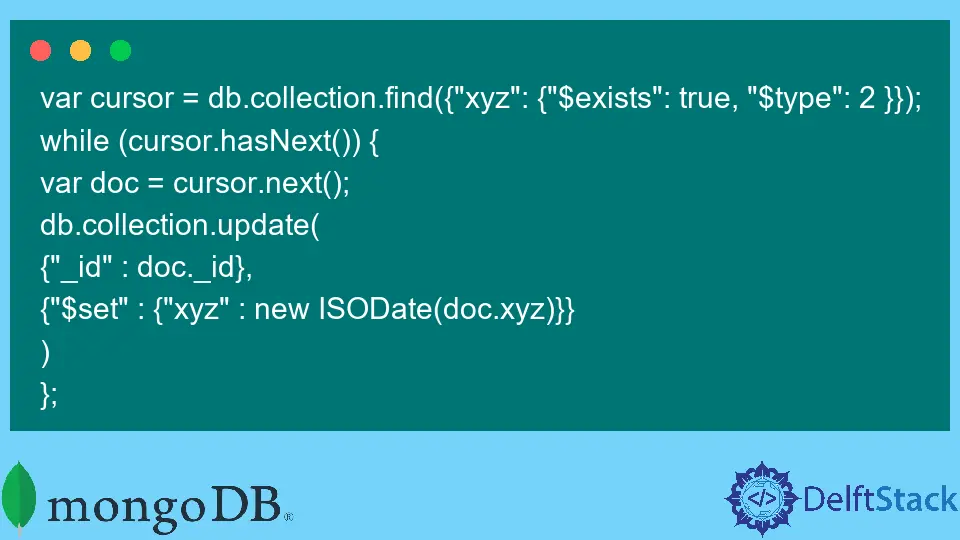
MongoDB is a great platform that has become increasingly popular. Amongst the various features that it offers, MongoDB also allows you to convert your data from one type to another.
This might seem like a complex feature, but it is pretty simple to execute.
If you’re looking for a way to convert your data from string to date, you’re in the right place.
Create String or Date Data Types
Before delving into how the conversion works, it is necessary to explain how strings and dates are created, to begin with. Ideally, a user picks his desired data type initially.
However, there may be an instance where you would like to change it later on. Regardless, here are some ways you can initially create a field with the date format.
{
birth: new Date('Jun 23, 1912'),
}
Therefore, the general structure would be the name of the field followed by a colon and then the data type.
Convert a String to Date in MongoDB
MongoDB does allow you to switch your data type from string to date. It is much simpler than you would expect.
All you need to do is add a few additional lines of code, and you’re good to go.
Use toDate
Operator
Amongst the multiple methods out there, the toDate
operator is probably the one that is used the most frequently. This is because it is fairly easy to remember and simple to execute.
This operator will convert the value to date if possible.
However, it returns errors if it cannot be changed to this format. In the same way, if the value that is to be converted is null or missing, the operator will return null.
It is important to note that you might not be able to use this method if your MongoDB version is older than MongoDB 4.0. Here is a simple way to use MongoDB’s toDate
operator.
db.collection.aggregate([
{ "$addFields": {
"created_at": {
"$toDate": "$created_at"
}
} }
])
This code will alter the fields stored in the required date format. As specified above, the operator returns errors or null values in case of any errors or missing data.
Note: This code has been designed to work within an aggregated pipeline. However, it may be modified to be used without one.
Use the convert
Operator
Another method with which you can convert strings to dates is the convert
operator. This works similarly to the toDate
operator as long as you convert the value into a date format.
The convert
operator is different because it is used for many conversions.
It isn’t limited to conversions from string to date. Therefore, while using the convert
operator, defining the data type, you wish to convert the value into is necessary.
This is often done using the to
operator after using the convert
operator. You may refer to the code below for a better understanding of its use.
db.collection.aggregate([
{ "$addFields": {
"created_at": {
"$convert": {
"input": "$created_at",
"to": "date"
}
}
} }
])
This code performs identically to the one above for the toDate
operator. As you can see, after specifying the convert
operator, the to
operator is used to specify that the value is to be converted into the date format.
Remember that this operator is only available for MongoDB 4.0 and newer versions.
Use the dateFromString
Operator
If you have an older version of MongoDB, you can always use the dateFromString
operator to get your job done. This will work for all versions of MongoDB 3.6 or newer, so you might need an update to work with this operator.
The dateFromString
operator allows you to convert a string to a date object. Moreover, it has a few additional features, such as options for specifying the date format and the timezone.
Here is a simple way you can use this operator.
db.collection.aggregate([
{ "$addFields": {
"created_at": {
"$dateFromString": {
"dateString": "$created_at",
"format": "%m-%d-%Y"/* this option is only available in MongoDB 4.0. or newer versions*/
}
}
} }
])
This code will allow you to make the desired conversion. Moreover, you can even alter the date format using the format
operator if you have the right version of MongoDB.
Use the set
Operator
If you prefer using an older version of MongoDB, you can still get the desired result by using the set
operator. This is available in all the versions of MongoDB between MongoDB 2.6 and MongoDB 3.1, including both.
It is important to note that this operator is not solely used for converting strings to dates. Therefore, you must include some manual processes to get the job done.
You will have to iterate the cursor returned by the find()
methods by using the forEach()
method or the cursor
method next()
to access what is required. You must convert the desired field into an ISODATE
object within this loop.
Finally, you will update the set
operator so that the string format can be updated. In the code below, xyz
is the field that contains the string, which has to be converted into a date format.
var cursor = db.collection.find({"xyz": {"$exists": true, "$type": 2 }});
while (cursor.hasNext()) {
var doc = cursor.next();
db.collection.update(
{"_id" : doc._id},
{"$set" : {"xyz" : new ISODate(doc.xyz)}}
)
};
Use ClockTime
Another method that works in most cases uses ClockTime
. This essentially uses the ClockTime
collection coupled with the find()
method to convert a string into the date format.
An example of the use is displayed in the code below.
db.ClockTime.find().forEach(function(doc) {
doc.ClockInTime=new Date(doc.ClockInTime);
db.ClockTime.save(doc);
})
We hope you can now find a method to convert strings to dates on MongoDB that works for you.
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHub