How to Compare Dates in MongoDB
- Using the $gt and $lt Operators
- Using the $gte and $lte Operators
- Using the Aggregation Framework
- Conclusion
- FAQ
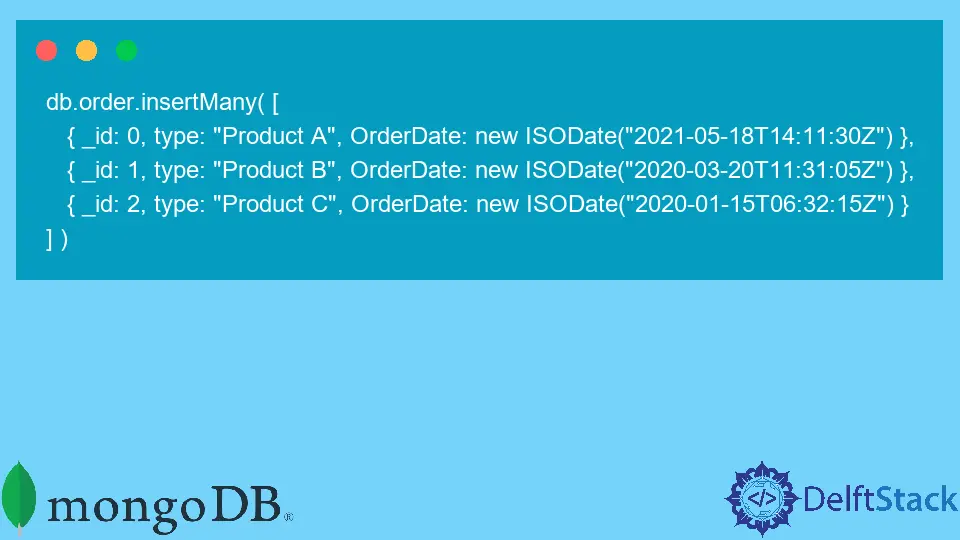
When working with databases, managing and comparing dates is a crucial skill that can significantly enhance your data querying capabilities. In MongoDB, the flexibility of date handling allows for a variety of comparisons, enabling you to filter data effectively based on time. Whether you are analyzing user activity, generating reports, or simply retrieving records within a specific timeframe, knowing how to compare dates is essential.
In this tutorial, we’ll dive into the various methods to compare dates in MongoDB, providing clear examples and explanations to help you master this task. Let’s get started!
Using the $gt and $lt Operators
One of the most straightforward ways to compare dates in MongoDB is by using the $gt
(greater than) and $lt
(less than) operators. These operators allow you to filter documents based on whether a date field falls before or after a specified date.
Here’s a quick example of how to use these operators to find documents with a date greater than a specific value.
from pymongo import MongoClient
from datetime import datetime
client = MongoClient('mongodb://localhost:27017/')
db = client['your_database']
collection = db['your_collection']
date_to_compare = datetime(2023, 1, 1)
results = collection.find({"date_field": {"$gt": date_to_compare}})
for document in results:
print(document)
In this code, we first connect to the MongoDB database and specify the collection we want to query. The date_to_compare
variable is set to January 1, 2023. The find
method retrieves all documents where the date_field
is greater than this date. The results are then printed out in a loop.
Output:
{ "_id": 1, "date_field": "2023-01-15", "data": "Sample Data 1" }
{ "_id": 2, "date_field": "2023-02-10", "data": "Sample Data 2" }
This method allows you to easily filter records based on whether their dates are greater than a specified date. You can also use $lt
to find records that are less than a certain date, following the same structure.
Using the $gte and $lte Operators
If you need to include the boundary dates in your comparison, the $gte
(greater than or equal to) and $lte
(less than or equal to) operators come in handy. These operators are particularly useful when you want to retrieve documents within a specific date range.
Here’s an example of how to use $gte
and $lte
to find documents between two dates:
from pymongo import MongoClient
from datetime import datetime
client = MongoClient('mongodb://localhost:27017/')
db = client['your_database']
collection = db['your_collection']
start_date = datetime(2023, 1, 1)
end_date = datetime(2023, 12, 31)
results = collection.find({"date_field": {"$gte": start_date, "$lte": end_date}})
for document in results:
print(document)
In this code snippet, we define a start_date
and an end_date
. The find
method retrieves all documents where the date_field
falls within this range, inclusive of both dates.
Output:
{ "_id": 1, "date_field": "2023-01-15", "data": "Sample Data 1" }
{ "_id": 2, "date_field": "2023-12-10", "data": "Sample Data 2" }
Using $gte
and $lte
is a powerful way to ensure that your queries are inclusive of the specified boundaries, making it an effective method for date range filtering.
Using the Aggregation Framework
For more complex date comparisons, MongoDB’s Aggregation Framework offers advanced capabilities. This allows you to perform operations such as grouping, filtering, and sorting based on date fields.
Here’s how you can use the aggregation pipeline to compare dates:
from pymongo import MongoClient
from datetime import datetime
client = MongoClient('mongodb://localhost:27017/')
db = client['your_database']
collection = db['your_collection']
pipeline = [
{
"$match": {
"date_field": {
"$gte": datetime(2023, 1, 1),
"$lt": datetime(2023, 12, 31)
}
}
},
{
"$group": {
"_id": "$date_field",
"total": {"$sum": 1}
}
}
]
results = collection.aggregate(pipeline)
for document in results:
print(document)
In this code, we define an aggregation pipeline that first matches documents with a date_field
between January 1, 2023, and December 31, 2023. The $group
stage then aggregates these results by the date_field
and counts the total occurrences for each date.
Output:
{ "_id": "2023-01-15", "total": 5 }
{ "_id": "2023-12-10", "total": 3 }
The Aggregation Framework is ideal for more complex queries, allowing you to analyze and manipulate your date data with precision.
Conclusion
In this tutorial, we explored how to compare dates in MongoDB using various methods, including the $gt
, $lt
, $gte
, $lte
operators, and the Aggregation Framework. Each method has its unique advantages, depending on your specific needs for querying and analyzing date data. By mastering these techniques, you can significantly enhance your data management skills in MongoDB. Whether you are filtering records, generating reports, or conducting analyses, knowing how to effectively compare dates will empower you to make the most of your data.
FAQ
-
How do I compare two dates in MongoDB?
You can use the$gt
,$lt
,$gte
, and$lte
operators to compare two dates in MongoDB queries. -
Can I compare dates with time in MongoDB?
Yes, MongoDB stores dates with time information, allowing you to perform comparisons that include both date and time. -
What is the Aggregation Framework in MongoDB?
The Aggregation Framework is a powerful tool in MongoDB that allows you to process data records and return computed results, making it suitable for complex queries. -
Can I use date comparison in indexes?
Yes, you can create indexes on date fields, which can optimize queries that involve date comparisons. -
How do I format dates when querying in MongoDB?
You can use Python’sdatetime
module to format dates before querying in MongoDB.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn