How to Aggregate Match in an Array in MongoDB
- MongoDB Aggregate Match in an Array
-
Use
$match
With$in
to Find Matching Documents in an Array in MongoDB -
Use
$match
With$eq
to Find Matching Documents in an Array in MongoDB -
Use
$match
With$all
to Find Matching Documents in an Array in MongoDB
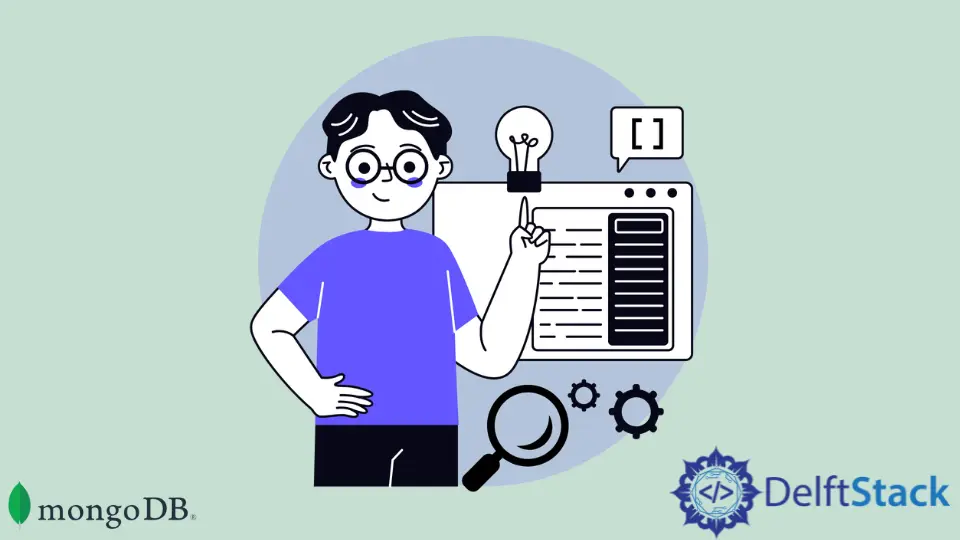
This article educates about using the MongoDB aggregate match in an array with the help of code examples. We will explore different examples using string type array and numeric type array.
MongoDB Aggregate Match in an Array
We have already learned to use the match aggregation stage with comparison operators, $and
/$or
operators and $group
/$project
stages.
Here, we focus on learning the MongoDB aggregate match in an array to find matching documents. To create a collection where at least one field contains an array.
You can take advantage of the commands below to follow along with us.
Example Code:
db.createCollection('student_courses');
db.student_courses.insertMany([
{"_id": "ma01", courses: ['Java', 'Python', 'Databases'], results: [3,5]},
{"_id": "sd02", courses: ['Java', 'Python'], results: [6,3]},
{"_id": "gh03", courses: ['JavaScript', 'Angular'], results: [8,9]},
{"_id": "rt04", courses: ['Data Science', 'Python'], results: [2,5]}
]);
db.student_courses.find();
Output:
{ "_id" : "ma01", "courses" : [ "Java", "Python", "Databases" ], "results" : [ 3, 5 ] }
{ "_id" : "sd02", "courses" : [ "Java", "Python" ], "results" : [ 6, 3 ] }
{ "_id" : "gh03", "courses" : [ "JavaScript", "Angular" ], "results" : [ 8, 9 ] }
{ "_id" : "rt04", "courses" : [ "Data Science", "Python" ], "results" : [ 2, 5 ] }
Use $match
With $in
to Find Matching Documents in an Array in MongoDB
Example Code:
db.student_courses.aggregate({
$match:{
$expr:{ $in: ['Java', "$courses"]}
}
});
Output:
{ "_id" : "ma01", "courses" : [ "Java", "Python", "Databases" ], "results" : [ 3, 5 ] }
{ "_id" : "sd02", "courses" : [ "Java", "Python" ], "results" : [ 6, 3 ] }
This code snippet uses the $match
with $expr
to let us use aggregation expressions within a query language. Here, the $expr
builds the query expression that compares the fields from the exact documents in the $match
stage.
Further, the $in
operator selects those documents where the courses
array contains Java
as an array element. To check multiple values in the specified array, use the query in the following manner.
Example Code:
db.student_courses.aggregate({
$match:{
courses:{ $in: ['Java', 'Python']}
}
});
Output:
{ "_id" : "ma01", "courses" : [ "Java", "Python", "Databases" ], "results" : [ 3, 5 ] }
{ "_id" : "sd02", "courses" : [ "Java", "Python" ], "results" : [ 6, 3 ] }
{ "_id" : "rt04", "courses" : [ "Data Science", "Python" ], "results" : [ 2, 5 ] }
This example selects only those documents where the courses
array has either Java
or Python
(or both). Similarly, we can work with a numeric array.
Example Code:
db.student_courses.aggregate({
$match:{
results:{ $in: [3,6]}
}
});
Output:
{ "_id" : "ma01", "courses" : [ "Java", "Python", "Databases" ], "results" : [ 3, 5 ] }
{ "_id" : "sd02", "courses" : [ "Java", "Python" ], "results" : [ 6, 3 ] }
This query retrieves only those documents where the results
array contains either 3
or 6
(or both).
Example Code:
db.student_courses.aggregate({
$match:{
$expr:{ $gt: [{$sum: "$results"}, 10]}
}
});
Output:
{ "_id" : "gh03", "courses" : [ "JavaScript", "Angular" ], "results" : [ 8, 9 ] }
Here, we select only those documents where the sum of all the elements of the results
array is greater than 10
.
Use $match
With $eq
to Find Matching Documents in an Array in MongoDB
Example Code:
db.student_courses.aggregate({
$match:{
courses:{ $eq: ['Java', 'Python']}
}
});
Output:
{ "_id" : "sd02", "courses" : [ "Java", "Python" ], "results" : [ 6, 3 ] }
Here, we use the $eq
operator to get only those elements where the courses
array contains the exact array as we specify with the $eq
operator.
Use $match
With $all
to Find Matching Documents in an Array in MongoDB
Example Code:
db.student_courses.aggregate({
$match:{
courses:{ $all: ['Java', 'Python']}
}
});
Output:
{ "_id" : "ma01", "courses" : [ "Java", "Python", "Databases" ], "results" : [ 3, 5 ] }
{ "_id" : "sd02", "courses" : [ "Java", "Python" ], "results" : [ 6, 3 ] }
The $all
is equivalent to the $and
operator. This code retrieves all those documents where the courses
array contains all the specified elements for the $all
operator.
The resulting documents must contain Java
and Python
elements.