How to Set Matplotlib Grid Interval
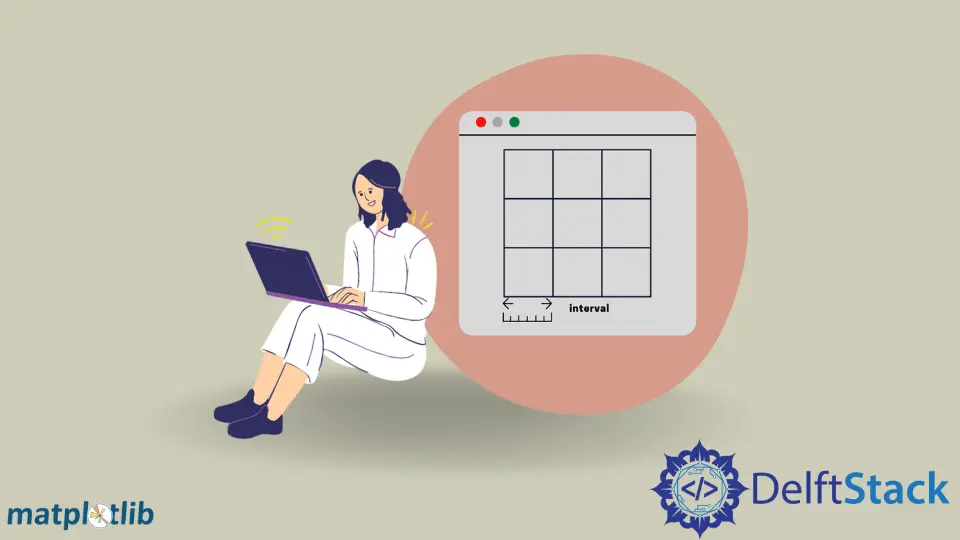
This tutorial will introduce how we can set grid spacing and apply different styles to major and minor grids in the Matplotlib plot.
We must use the matplotlib.pyplot.grid()
function to show the grids.
import matplotlib.pyplot as plt
plt.figure(figsize=(8, 6))
plt.xlim(0, 10)
plt.ylim(0, 10)
plt.grid()
plt.title("Plot with Grids")
plt.show()
Output:
It displays a plot with grids in which grids are spaced as per the spacing of the ticks. We can change the grid interval by changing the spacing of ticks
.
Change Matplotlib Plot Grid Spacing by Changing the Spacing of the Ticks
import matplotlib.pyplot as plt
fig, axes = plt.subplots(nrows=2, ncols=1, figsize=(8, 6))
axes[0].set_xlim(0, 10)
axes[0].set_ylim(0, 10)
axes[0].set_title("Subplot 1")
axes[0].grid()
axes[1].set_xlim(0, 8)
axes[1].set_ylim(0, 8)
axes[1].set_title("Subplot 2")
axes[1].grid()
plt.show()
Output:
It creates a figure with two subplots. We have set the different spacing of ticks for both subplots. From the figure, it is clear that grids’ spacing is maintained as per ticks are spaced.
import numpy as np
import matplotlib.pyplot as plt
fig, axes = plt.subplots(nrows=2, ncols=1, figsize=(8, 6))
major_ticks_top = np.linspace(0, 20, 6)
minor_ticks_top = np.linspace(0, 20, 21)
major_ticks_bottom = np.linspace(0, 15, 6)
axes[0].set_xticks(major_ticks_top)
axes[0].set_yticks(major_ticks_top)
axes[0].set_xticks(minor_ticks_top, minor=True)
axes[0].set_yticks(minor_ticks_top, minor=True)
axes[0].set_title("Subplot 1")
axes[0].grid(which="major", alpha=0.6)
axes[0].grid(which="minor", alpha=0.3)
axes[1].set_xticks(major_ticks_bottom)
axes[1].set_yticks(major_ticks_bottom)
axes[1].set_title("Subplot 2")
axes[1].grid()
plt.tight_layout()
plt.show()
Output:
It displays a Matplotlib figure with two subplots in it. Subplot 1
has both minor and major grids. The minor grids are located with a spacing of 1 unit and represented with a darker line. Similarly, the major grids are placed with the spacing of 5 units and represented by a lighter line.
We use minor=True
to denote minor ticks in set_yticks()
or set_xticks()
functions. We use which="major"
to select grid corresponding to major
ticks, and which="major"
to select grid corresponding to minor
ticks.
The alpha
parameter in the grid()
method is used to set the grid lines’ intensity. The higher the value of alpha
, the darker the grid lines are.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn