How to Plot Horizontal Line in Python
- Horizontal Line in Python
-
Use the
plot()
Function in Python -
Use the
hlines()
Function in Python -
Use the
axhline()
Function in Python - Dotted Horizontal Line in Python
- Horizontal Line With Label in Python
- Multiple Horizontal Line Matplotlib
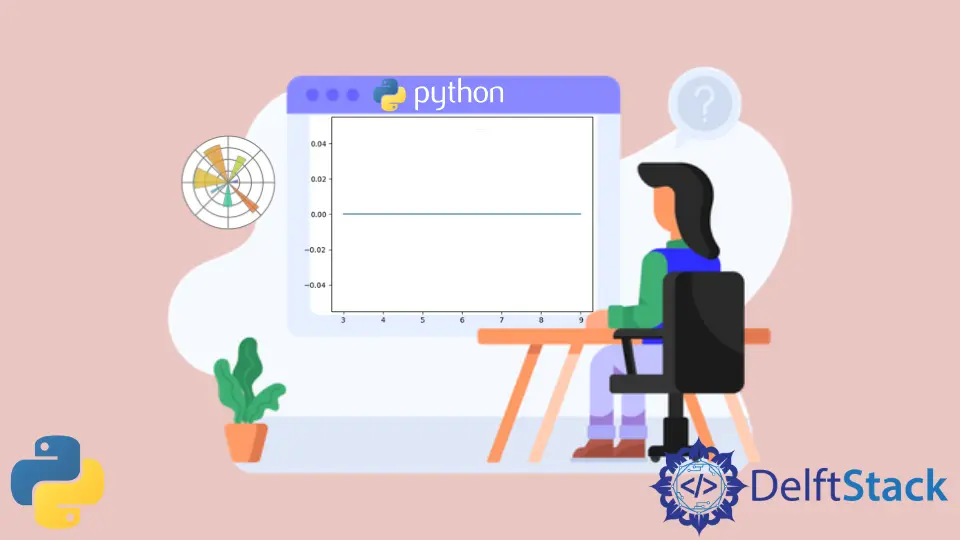
We will introduce how to create a horizontal line in Python. We will also introduce the Matplotlib
library in Python.
Horizontal Line in Python
A horizontal line is any straight line that drives from left to right or right to left. When we see it in a coordinate plane, it is a line parallel to the x-axis.
In Python, Matplotlib
is popularly used for plotting. There are many methods available to plot a horizontal line which is as follows.
- Plotting horizontal line by the
plot()
function. - Plotting horizontal lines by the
hlines()
function. - Plotting horizontal line by the
axhline()
function.
Use the plot()
Function in Python
When our goal is to produce 2D plots, we can use the Plot()
function. X points are x-axis points towards the plot, and Y points are y-axis points.
Code:
# python
import matplotlib.pyplot as plotLine
xAxis = [3, 5, 7, 9]
yAxis = [0, 0, 0, 0]
plotLine.plot(xAxis, yAxis)
plotLine.show()
Output:
First, we imported the matplotlib.pyplot
library, then outlined the data points we wanted to plot. In this example, we stated the y-axis points to be 0 because our goal is to draw a horizontal line.
We apply the plotLine.plot()
function to draw a line, and for visual purposes, we have used plotLine.show()
.
Use the hlines()
Function in Python
When we want to draw a horizontal line across the axes, we use the hlines()
function. This function will simplify our task.
Syntax:
# python
hlines(Yaxis, XaxisStart, XaxisEnd, lineColor, lineStyle)
Here, four parameters are used, Yaxis
will denote the position on the y-axis when we need to plot a line. XaxisStart
and XaxisEnd
indicate where the line starts and where it will end.
The lineColor
will add the required colors to the line, and the lineStyle
will add the style or type of the line we specified.
Code:
# python
import matplotlib.pyplot as plotLine
plotLine.hlines(3, 5, 10, color="blue")
plotLine.show()
Output:
We used the matplotlib.pyplot
library to create a horizontal line with the help of the hlines()
function. As an argument, we have passed the values and got the result as shown above.
Use the axhline()
Function in Python
The axhline()
function is designed to draw horizontal lines to the plot. The axhline()
function has similar parameters as in hlines()
function.
Code:
# python
import matplotlib.pyplot as plotLine
plotLine.axhline(y=1.3, xmin=0.2, xmax=0.7)
plotLine.show()
Output:
We have drawn a horizontal line and authorized the y
, xmin
, and xmax
as parameters and fixed them as 1.3, 0.2, and 0.7.
Dotted Horizontal Line in Python
Matplotlib
library also allows us to have a dotted line. When we need a dotted horizontal line, we have to change the line style to a dotted line, which will do the trick for us.
The Matplotlib.pyplot
library offers the linestyle
parameter to set the line type.
Code:
# python
import matplotlib.pyplot as plotLine
plotLine.axhline(y=1.3, xmin=0.2, xmax=0.7, linestyle="dotted")
plotLine.show()
Output:
The axhline()
function have four parameters y
, xmin
, xmax
, and linestyle
. Our goal is to achieve a horizontal line dotted in style, so we fixed linestyle
to dotted.
Horizontal Line With Label in Python
We can also achieve a horizontal line with a label with the help of the axhline()
function. We have to set the label
as a parameter.
Code:
# python
import matplotlib.pyplot as plotLine
plotLine.axhline(y=1.3, xmin=0.2, xmax=0.7, label="Line Label")
plotLine.legend(loc="upper left")
plotLine.show()
Output:
We can easily create a label for the horizontal line by using the label
parameter. We can define the label’s location using another function, legend()
.
Multiple Horizontal Line Matplotlib
We can also achieve multiple horizontal lines in matplotlib
in Python. There are two methods available by which we can achieve our goal, using the axhline()
method or by using the hlines()
method.
Axhline()
method allows us to obtain multiple horizontal lines in the plot.
Code:
# python
import matplotlib.pyplot as plotLine
plotLine.axhline(y=1.3, xmin=0.2, xmax=0.7, label="Blue Line Label")
plotLine.legend(loc="upper left")
plotLine.axhline(y=1.8, xmin=0.6, xmax=0.9, label="Red Line Label", color="red")
plotLine.legend(loc="upper left")
plotLine.axhline(y=1.5, xmin=0.5, xmax=0.9, label="Yellow Line Label", color="yellow")
plotLine.legend(loc="upper left")
plotLine.show()
Output:
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn