Python에서 가로선 그리기
- Python의 수평선
-
Python에서
plot()
함수 사용 -
Python에서
hlines()
함수 사용 -
Python에서
axhline()
함수 사용 - Python의 점선 수평선
- Python의 레이블이 있는 수평선
- 다중 수평 라인 Matplotlib
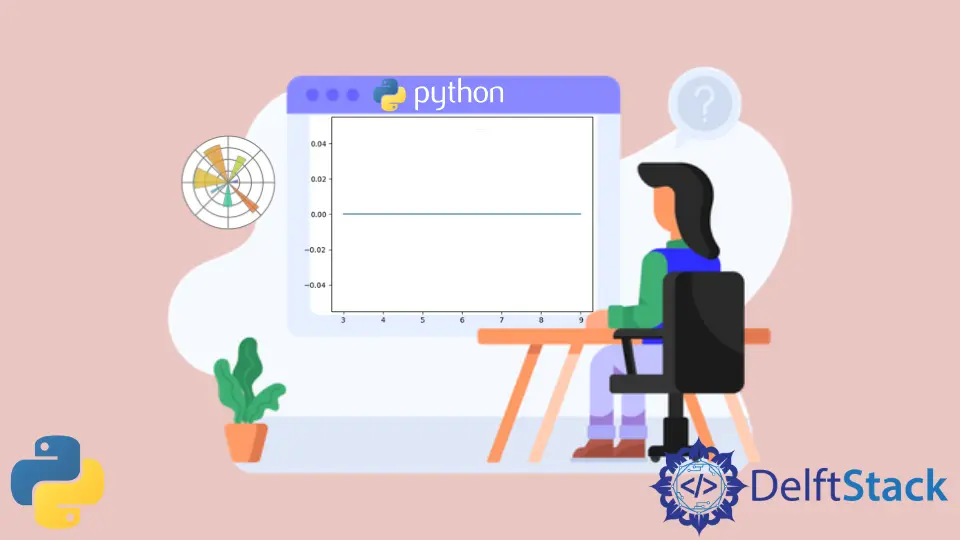
Python에서 수평선을 만드는 방법을 소개합니다. Python의 Matplotlib
라이브러리도 소개합니다.
Python의 수평선
수평선은 왼쪽에서 오른쪽으로 또는 오른쪽에서 왼쪽으로 움직이는 모든 직선입니다. 좌표평면에서 보면 x축에 평행한 선이다.
Python에서 Matplotlib
은 플로팅에 널리 사용됩니다. 다음과 같이 수평선을 그리는 데 사용할 수 있는 많은 방법이 있습니다.
plot()
함수로 수평선을 그립니다.hlines()
함수로 수평선 그리기.axhline()
함수로 수평선을 그립니다.
Python에서 plot()
함수 사용
목표가 2D 플롯을 생성하는 것이라면 Plot()
함수를 사용할 수 있습니다. X 포인트는 플롯을 향한 x축 포인트이고 Y 포인트는 y축 포인트입니다.
암호:
# python
import matplotlib.pyplot as plotLine
xAxis = [3, 5, 7, 9]
yAxis = [0, 0, 0, 0]
plotLine.plot(xAxis, yAxis)
plotLine.show()
출력:
먼저 matplotlib.pyplot
라이브러리를 가져온 다음 플롯하려는 데이터 포인트의 윤곽을 그렸습니다. 이 예에서는 목표가 수평선을 그리는 것이므로 y축 점을 0으로 지정했습니다.
plotLine.plot()
함수를 적용하여 선을 그리고 시각적 목적으로 plotLine.show()
를 사용했습니다.
Python에서 hlines()
함수 사용
축을 가로지르는 수평선을 그리려면 hlines()
함수를 사용합니다. 이 기능은 작업을 단순화합니다.
통사론:
# python
hlines(Yaxis, XaxisStart, XaxisEnd, lineColor, lineStyle)
여기에서 4개의 매개변수가 사용됩니다. Y축
은 선을 그려야 할 때 y축의 위치를 나타냅니다. XaxisStart
및 XaxisEnd
는 라인이 시작되는 위치와 종료되는 위치를 나타냅니다.
lineColor
는 선에 필요한 색상을 추가하고 lineStyle
은 지정한 선의 스타일 또는 유형을 추가합니다.
암호:
# python
import matplotlib.pyplot as plotLine
plotLine.hlines(3, 5, 10, color="blue")
plotLine.show()
출력:
matplotlib.pyplot
라이브러리를 사용하여 hlines()
함수의 도움으로 수평선을 만들었습니다. 인수로 값을 전달하고 위와 같이 결과를 얻었습니다.
Python에서 axhline()
함수 사용
axhline()
함수는 플롯에 수평선을 그리도록 설계되었습니다. axhline()
함수에는 hlines()
함수와 유사한 매개변수가 있습니다.
암호:
# python
import matplotlib.pyplot as plotLine
plotLine.axhline(y=1.3, xmin=0.2, xmax=0.7)
plotLine.show()
출력:
수평선을 그리고 y
, xmin
및 xmax
를 매개변수로 승인하고 1.3, 0.2 및 0.7로 고정했습니다.
Python의 점선 수평선
Matplotlib
라이브러리를 사용하면 점선을 사용할 수도 있습니다. 점선으로 된 수평선이 필요할 때 선 스타일을 점선으로 변경해야 합니다.
Matplotlib.pyplot
라이브러리는 선 유형을 설정하는 linestyle
매개변수를 제공합니다.
암호:
# python
import matplotlib.pyplot as plotLine
plotLine.axhline(y=1.3, xmin=0.2, xmax=0.7, linestyle="dotted")
plotLine.show()
출력:
axhline()
함수에는 y
, xmin
, xmax
및 linestyle
의 네 가지 매개변수가 있습니다. 우리의 목표는 수평선 스타일의 점선을 만드는 것이므로 linestyle
을 점선으로 고정했습니다.
Python의 레이블이 있는 수평선
axhline()
함수를 사용하여 레이블이 있는 수평선을 얻을 수도 있습니다. 레이블
을 매개변수로 설정해야 합니다.
암호:
# python
import matplotlib.pyplot as plotLine
plotLine.axhline(y=1.3, xmin=0.2, xmax=0.7, label="Line Label")
plotLine.legend(loc="upper left")
plotLine.show()
출력:
label
매개변수를 사용하여 수평선에 대한 레이블을 쉽게 생성할 수 있습니다. 다른 함수인 legend()
를 사용하여 라벨의 위치를 정의할 수 있습니다.
다중 수평 라인 Matplotlib
Python의 matplotlib
에서 여러 개의 수평선을 얻을 수도 있습니다. axhline()
메서드 또는 hlines()
메서드를 사용하여 목표를 달성할 수 있는 두 가지 방법이 있습니다.
Axhline()
메서드를 사용하면 플롯에서 여러 수평선을 얻을 수 있습니다.
암호:
# python
import matplotlib.pyplot as plotLine
plotLine.axhline(y=1.3, xmin=0.2, xmax=0.7, label="Blue Line Label")
plotLine.legend(loc="upper left")
plotLine.axhline(y=1.8, xmin=0.6, xmax=0.9, label="Red Line Label", color="red")
plotLine.legend(loc="upper left")
plotLine.axhline(y=1.5, xmin=0.5, xmax=0.9, label="Yellow Line Label", color="yellow")
plotLine.legend(loc="upper left")
plotLine.show()
출력:
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn