How to Create a Surface Plot in Matplotlib
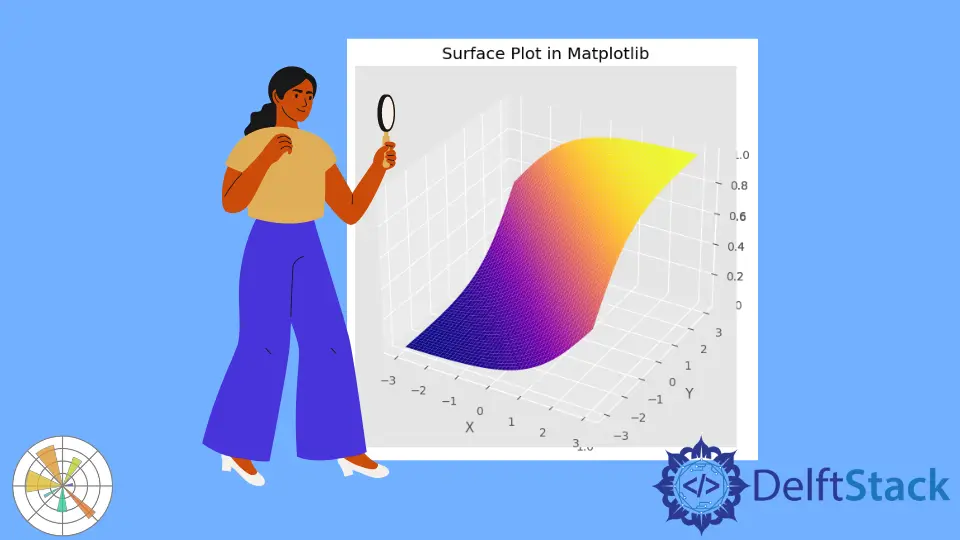
In Matplotlib, we use the mplot3d
toolkit for 3-D analysis and visualization which contains 3-D plotting methods built on top of Matplotlib’s 2-D functions. We can create 3-D axes by passing projection='3d'
argument to any of the axes’ creation functions in Matplotlib. Once 3-D axes are initialized, we can use the plot_surface()
method to generate surface plots.
Axes3D.plot_surface()
Method
We can create a surface plot using Axes3D.plot_surface(X, Y, Z, *args, **kwargs)
method where X, Y, and Z all are 2-D arrays.
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits import mplot3d
fig = plt.figure(figsize=(8, 6))
ax3d = plt.axes(projection="3d")
xdata = np.linspace(-3, 3, 100)
ydata = np.linspace(-3, 3, 100)
X, Y = np.meshgrid(xdata, ydata)
Z = 1 / (1 + np.exp(-X - Y))
ax3d = plt.axes(projection="3d")
ax3d.plot_surface(X, Y, Z, cmap="plasma")
ax3d.set_title("Surface Plot in Matplotlib")
ax3d.set_xlabel("X")
ax3d.set_ylabel("Y")
ax3d.set_zlabel("Z")
plt.show()
This generates a surface plot in the 3D space using Matplotlib. Here cmap
parameter is used to make a good representation of our data in 3D colorspace. The color of the plot is varied with variation in the value of the dependent variable.
We can customize the plot varying following parameters:
rstride
: Row step size whose default value is 10cstride
: Column step size whose default value is 10color
: Color of the surfacecmap
: Colormap of surfacefacecolors
: Face colors for each patch in the surfacenorm
: An instance of Normalize to map values to colorsvmin
: Minimum value to mapvmax
: Maximum value to mapshade
: Whether to shade the facecolors
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits import mplot3d
fig = plt.figure(figsize=(8, 6))
ax3d = plt.axes(projection="3d")
xdata = np.linspace(-3, 3, 100)
ydata = np.linspace(-3, 3, 100)
X, Y = np.meshgrid(xdata, ydata)
Z = 1 / (1 + np.exp(-X - Y))
ax3d = plt.axes(projection="3d")
surf = ax3d.plot_surface(X, Y, Z, rstride=7, cstride=7, cmap="viridis")
fig.colorbar(surf, ax=ax3d)
ax3d.set_title("Surface Plot in Matplotlib")
ax3d.set_xlabel("X")
ax3d.set_ylabel("Y")
ax3d.set_zlabel("Z")
plt.savefig("Customized Surface Plot.png")
plt.show()
In this example, we add a color bar in the figure by using the colorbar()
method and passing surface plot object to the method which makes the figure more informative.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn