How to Create Colored Triangle in Matplotlib
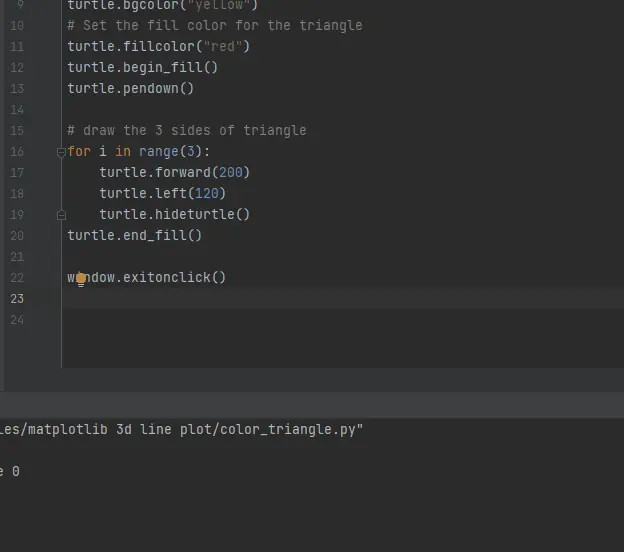
In this tutorial, we’ll use Python Matplotlib code to draw ourselves a simple colored triangle. We’ll be using the turtle
library that comes with Python.
Create a Colored Triangle With Turtle Library in Matplotlib
Make yourself a new blank file to get started on our colored triangle. Once we have created a new blank file, we need to import the turtle
library.
The turtle
library is a code written by another program to save us from having to do all the complex coding to get drawing on the screen. Once you have imported the turtle
library, we will make a new pop-up window on the screen.
Now we create a variable called window
and access the turtle
library to use the Screen()
method.
import turtle
# Set up the new blank window
window = turtle.Screen()
The background color of our pop-up screen can be changed using the turtle.bgcolor()
method.
turtle.bgcolor("yellow")
We will now choose a fill color for our triangle using the fillcolor()
method. After that, we need to write the begin_fill()
method to input the shape that we will draw that needs to be filled in with the fillcolor()
method.
Next is, we need to use the pendown()
method to draw on the screen.
turtle.fillcolor("red")
turtle.begin_fill()
turtle.pendown()
To draw this triangle, we will use a for
loop. Since a triangle has three sides, we will do it three times.
The forward()
method moves our turtle forward for a certain distance, and we can pass the distance as an integer in this method. So this is moving forward 200 pixels and then turning our turtle to the left 120 degrees using the left()
method.
The hideturtle()
method helps us hide the turtle’s head.
# draw the 3 sides of triangle
for i in range(3):
turtle.forward(200)
turtle.left(120)
turtle.hideturtle()
The next is we jump out of the for
loop so that we are no longer indented. We will write the end_fill()
method that stops filling in more shapes with that color.
Finally, we have our three-sided triangle drawn by the turtle
library. The last thing we will write is the exitonclick()
method to exit the window when we click on our window.
turtle.end_fill()
window.exitonclick()
Full Code Example:
import turtle
# Set up the new blank window
window = turtle.Screen()
# Set the fill back ground color
turtle.bgcolor("yellow")
# Set the fill color for the triangle
turtle.fillcolor("red")
turtle.begin_fill()
turtle.pendown()
# draw the 3 sides of triangle
for i in range(3):
turtle.forward(200)
turtle.left(120)
turtle.hideturtle()
turtle.end_fill()
window.exitonclick()
Output:
Click here to read more about the turtle
library.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn