在 Matplotlib 中创建彩色三角形
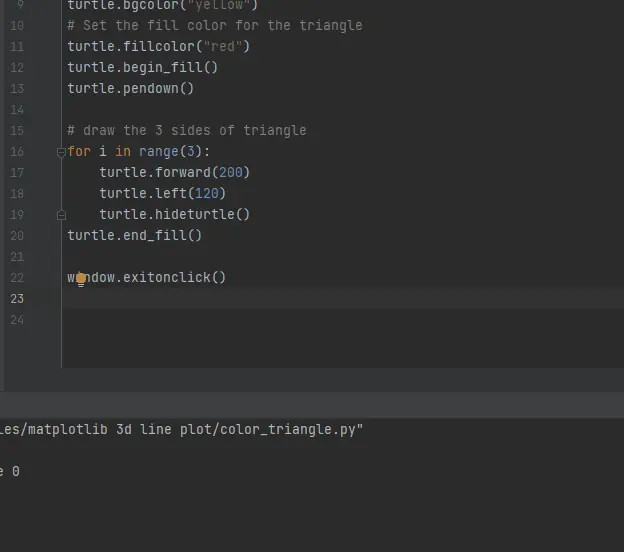
在本教程中,我们将使用 Python Matplotlib 代码为自己绘制一个简单的彩色三角形。我们将使用 Python 附带的 turtle
库。
在 Matplotlib 中使用 Turtle 库创建彩色三角形
为自己创建一个新的空白文件,开始使用我们的彩色三角形。一旦我们创建了一个新的空白文件,我们需要导入 turtle
库。
turtle
库是由另一个程序编写的代码,它使我们不必进行所有复杂的编码以在屏幕上绘图。导入 turtle
库后,我们将在屏幕上创建一个新的弹出窗口。
现在我们创建一个名为 window
的变量并访问 turtle
库以使用 Screen()
方法。
import turtle
# Set up the new blank window
window = turtle.Screen()
可以使用 turtle.bgcolor()
方法更改弹出屏幕的背景颜色。
turtle.bgcolor("yellow")
我们现在将使用 fillcolor()
方法为我们的三角形选择填充颜色。之后,我们需要编写 begin_fill()
方法来输入我们将要绘制的需要用 fillcolor()
方法填充的形状。
接下来,我们需要使用 pendown()
方法在屏幕上绘图。
turtle.fillcolor("red")
turtle.begin_fill()
turtle.pendown()
为了绘制这个三角形,我们将使用一个 for
循环。由于三角形有三个边,我们将做 3 次。
forward()
方法将我们的 turtle 向前移动一段距离,我们可以在此方法中将距离作为整数传递。所以这是向前移动 200 像素,然后使用 left()
方法将我们的 turtle 向左旋转 120 度。
hideturtle()
方法帮助我们隐藏 turtle 的头部。
# draw the 3 sides of triangle
for i in range(3):
turtle.forward(200)
turtle.left(120)
turtle.hideturtle()
接下来是我们跳出 for
循环,这样我们就不再缩进了。我们将编写 end_fill()
方法来停止用该颜色填充更多形状。
最后,我们得到了由 turtle
库绘制的三边三角形。我们将编写的最后一件事是当我们单击窗口时退出窗口的 exitonclick()
方法。
turtle.end_fill()
window.exitonclick()
完整代码示例:
import turtle
# Set up the new blank window
window = turtle.Screen()
# Set the fill back ground color
turtle.bgcolor("yellow")
# Set the fill color for the triangle
turtle.fillcolor("red")
turtle.begin_fill()
turtle.pendown()
# draw the 3 sides of triangle
for i in range(3):
turtle.forward(200)
turtle.left(120)
turtle.hideturtle()
turtle.end_fill()
window.exitonclick()
输出:
点击这里阅读更多关于 turtle
库的信息。
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn