How to Create Circle Arrow in Matplotlib
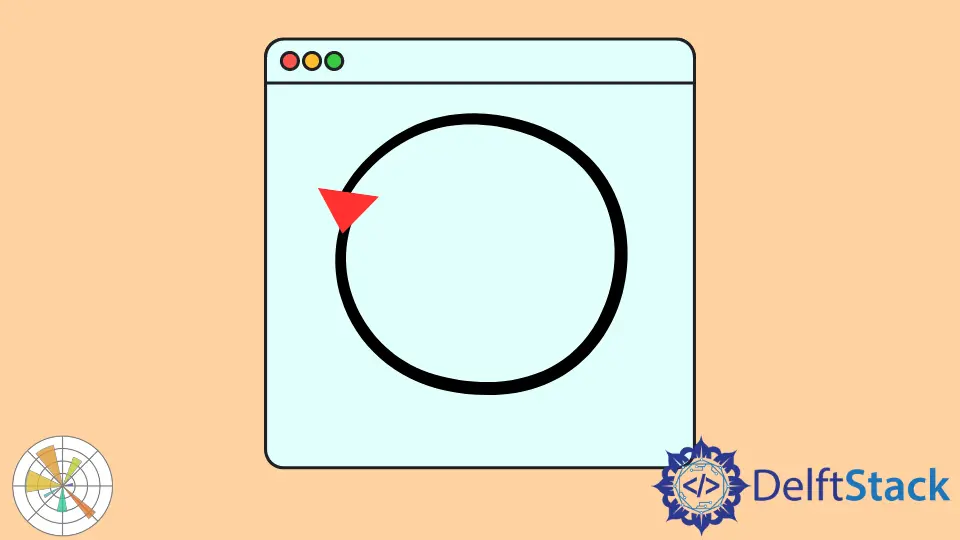
The turtle graphics is this nice and easy-to-use tool that allows you to get the hang of the coding syntax. We can create little animations using this module, moving objects around the screen and drawing the shapes.
In this tutorial, we’re going to draw a circle arrow with the help of the turtle
library in Python Matplotlib.
Create Circle Arrow With Turtle Module in Python Matplotlib
Let’s get started on the circle arrow by making a new file. Then we import the turtle
module into our program.
A little turtle appears on the screen and will draw stuff on the canvas or your window. So once you have imported the turtle
library, we need to create a pop-up window.
This is the window that is going to display our circle arrow. We make a window
variable and initialize the turtle.Screen()
method to access a new pop-up window on our screen.
The setup()
function set’s up to our screen with 600x500. The first parameter is the width, and the second parameter is the height.
import turtle
window = turtle.Screen() # set the pop up window
window.setup(600, 500)
The screen will be a canvas for us to draw on at the moment. We will use the bgcolor()
method to put color under this new window.
This method accepts whatever color you want your background to be as a string. We need to choose the color for our circle arrow by calling the color()
method.
In our case, we want magenta as the color of our arrow.
turtle.bgcolor("Yellow") # Change the background color of the window
turtle.color("magenta", "yellow")
We can change the size of the stroke or the border using the pensize()
method. The shape()
method helps us set our turtle’s head.
For now, we pass the "arrow"
as a string to create a circle arrow. Before creating a circle arrow, we need to use the begin_fill()
method to start filling in the shape’s color.
To draw a circle arrow, we use the circle()
method and pass it as an integer value which is the radius of our circle arrow. Once we have drawn our desired shape, we’ll use the end_fill()
method to stop the fill color from being used anywhere.
The exitonclick()
method exits the window when you click anywhere on the page.
turtle.pensize(5) # change the stroke on the shape
turtle.shape("arrow")
# Draw the circle arrow and fill it with the color
turtle.begin_fill()
turtle.circle(80) # Radius of 80px
turtle.end_fill()
window.exitonclick()
Full Code Example:
import turtle
window = turtle.Screen() # set the pop up window
window.setup(600, 500)
turtle.bgcolor("Yellow") # Change the background color of the window
turtle.color("magenta", "yellow")
turtle.pensize(5) # change the stroke on the shape
turtle.shape("arrow")
# Draw the circle arrow and fill it with the color
turtle.begin_fill()
turtle.circle(80) # Radius of 80px
turtle.end_fill()
window.exitonclick() # When the page is clicked,the app closes
Output:
Click here to read detailed documentation of the turtle
library.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn