How to Calculate Fibonacci Sequence in MATLAB
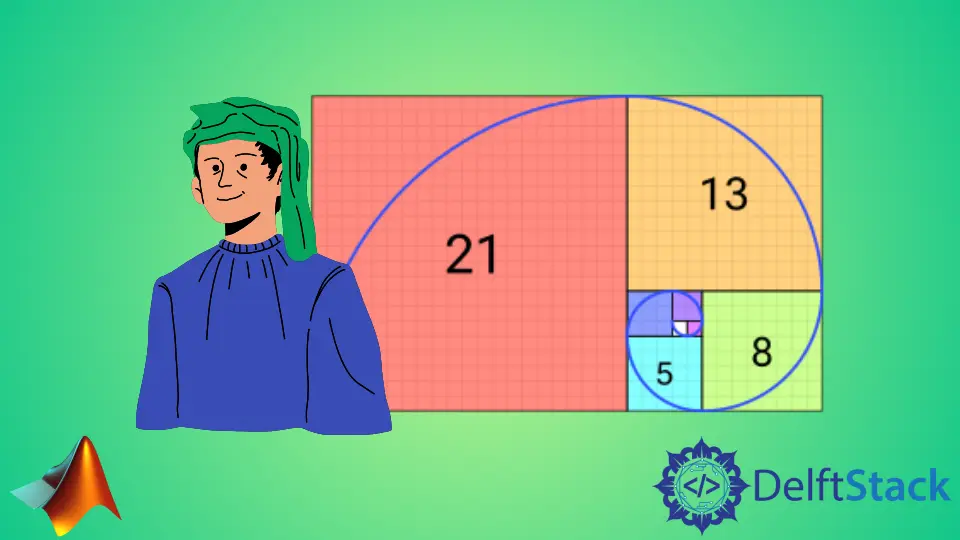
This tutorial will discuss generating the Fibonacci numbers using the fibonacci()
function in MATLAB.
MATLAB Fibonacci Sequence
Each number in the Fibonacci sequence is the sum of the two values before that number. The Fibonacci series is used in many applications like converting kilometers to miles, checking the effort needed to complete a task, and comparing two stories, data structures, and distribution systems.
For example, suppose we want to convert 34 miles to kilometers. In that case, we can use the Fibonacci series to find the value next to 34, which is 55 in the Fibonacci series, and it will be approximately equal to the real value of 34 miles in kilometers.
If we want to convert kilometers to miles, we can find the previous value in the Fibonacci series.
The formula used to generate the Fibonacci sequence is given below.
Fn = Fn-1 + Fn-2
In the above formula, n
represents the number of values, and its value should be greater than 1. For example, if we want to find the fifth value of the Fibonacci sequence, we can put 5 in the above formula, and the new formula is given below.
F5 = F4 + F3
We must add the third and fourth values from the Fibonacci sequence to find the fifth value. In Matlab, we can use the fibonacci()
function to find any value from the Fibonacci sequence.
For example, let’s use the fibonacci()
function to find the tenth value of the Fibonacci sequence. See the code below.
clc
clear
fn = fibonacci(10)
Output:
fn = 55
We used the clc
and clear
commands in the above code to clear the command and the workspace window. We can see in the output that the fibonacci()
function returned the tenth value of the Fibonacci sequence, which is 55
.
We can also generate the Fibonacci sequence up to a specific value using the fibonacci()
function in Matlab. We have to create a vector containing all the Fibonacci numbers we want to generate, and then we can pass the vector inside the fibonacci()
function to generate the sequence.
For example, let’s generate the first 10 Fibonacci values using the fibonacci()
function in Matlab. See the code below.
clc
clear
v = 1:10;
fn = fibonacci(v)
Output:
fn =
1 1 2 3 5 8 13 21 34 55
We can see in the above code that the fibonacci()
function returned the first 10 numbers of the Fibonacci series. In the case of a big Fibonacci number, the value will be converted to a double
data type, but we can use the sym()
function to get the value in symbolic form.
For example, let’s find the three hundredth value of the Fibonacci sequence with and without the sym()
function to check the difference in the output of the fibonacci()
function. See the code below.
clc
clear
v = sym(300);
v1 = 300;
fn = fibonacci(v)
fn1 = fibonacci(v1)
Output:
fn =
222232244629420445529739893461909967206666939096499764990979600
fn1 =
2.2223e+62
The first value in the output is in the symbolic form because we used the sym()
function to define the value, and the second value is in the double
data type, which is why it is converted to a short form because of the output value is too large. This link has more details about the fibonacci()
function.