How to Use the shift Command in Bash Scripts
-
What is the
shift
Command? - Basic Usage of Shift
- Shifting with a Specified Number
- Using Shift in a Loop
- Conclusion
- FAQ
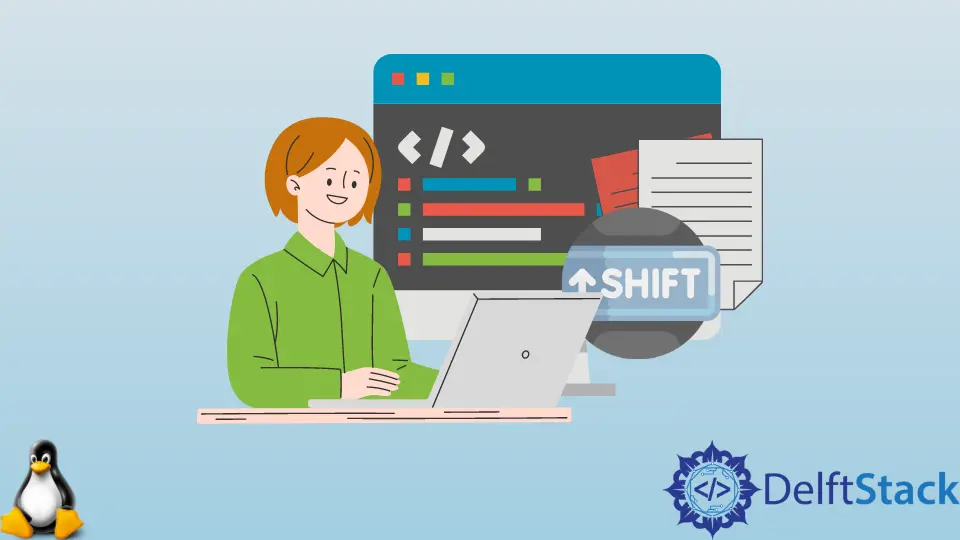
In the world of Bash scripting, understanding how to manipulate command-line arguments is crucial for creating flexible and dynamic scripts. One of the key tools at your disposal is the shift
command. This command allows you to shift the positional parameters to the left, effectively discarding the first argument and bringing the subsequent arguments into the spotlight. Whether you’re parsing user input or managing a series of commands, mastering the shift
command can streamline your scripts significantly.
In this tutorial, we will explore how to use the shift
command in Bash scripts, providing you with practical examples and insights along the way.
What is the shift
Command?
The shift
command in Bash is a built-in utility that modifies the positional parameters of a script. When you run shift
, the first positional parameter ($1) is removed, and all other parameters are shifted down by one position. If you have multiple parameters, you can specify how many positions to shift by providing an optional numeric argument. For example, shift 2
would remove the first two parameters.
Understanding the shift
command is essential for handling scripts that require variable-length arguments. This command is particularly useful in loops, where you may want to process each argument one by one.
Basic Usage of Shift
Let’s start with a basic example of how to use the shift
command in a Bash script. In this scenario, we will create a simple script that accepts multiple arguments and prints them one by one.
#!/bin/bash
while [ "$#" -gt 0 ]; do
echo "Argument: $1"
shift
done
Output:
Argument: arg1
Argument: arg2
Argument: arg3
In this script, we first check if there are any arguments left by evaluating "$#"
(the number of positional parameters). If there are, we print the first argument using $1
and then call shift
to remove it. This process continues until all arguments have been processed. This method is straightforward and allows you to handle any number of input arguments seamlessly.
Shifting with a Specified Number
Sometimes, you may want to shift multiple arguments at once. The shift
command allows you to do just that. By providing a numeric argument, you can specify how many positions to shift. Here’s an example that demonstrates this feature.
#!/bin/bash
echo "Original arguments: $@"
shift 2
echo "After shifting: $@"
Output:
Original arguments: arg1 arg2 arg3 arg4
After shifting: arg3 arg4
In this script, we first print all the original arguments using $@
, which represents all positional parameters. After calling shift 2
, we remove the first two arguments, allowing us to see the remaining ones. This capability is especially useful when you know exactly how many arguments you want to ignore or process at once.
Using Shift in a Loop
The shift
command becomes even more powerful when used within loops. It allows for a more controlled and dynamic processing of arguments. This next example will demonstrate how to implement shift
in a loop to handle a series of commands or options.
#!/bin/bash
while [ "$#" -gt 0 ]; do
case "$1" in
-h|--help)
echo "Help: This script processes arguments."
shift
;;
-v|--version)
echo "Version: 1.0"
shift
;;
*)
echo "Processing argument: $1"
shift
;;
esac
done
Output:
Processing argument: arg1
Processing argument: arg2
Help: This script processes arguments.
In this example, we use a case
statement to handle different flags, such as -h
for help and -v
for version. Each time we process an argument, we call shift
to move to the next one. This structure allows for flexible command-line options, making your script more user-friendly and efficient.
Conclusion
The shift
command is an essential tool in Bash scripting that enables you to manipulate positional parameters effectively. By mastering its usage, you can create scripts that are more dynamic and capable of handling a variety of input scenarios. Whether you are processing user input, managing command-line options, or iterating through arguments, the shift
command can significantly enhance your scripting capabilities. With the examples provided, you should now have a solid understanding of how to implement the shift
command in your own Bash scripts.
FAQ
-
What does the shift command do in Bash?
The shift command shifts the positional parameters to the left, removing the first argument and bringing the others forward. -
Can I shift multiple arguments at once?
Yes, you can specify a number with the shift command to remove multiple arguments at once, likeshift 2
. -
How do I check the number of arguments in a Bash script?
You can check the number of arguments using the special variable$#
, which returns the count of positional parameters. -
Is the shift command useful in loops?
Absolutely! The shift command is particularly effective in loops, allowing you to process each argument sequentially. -
Can I use shift with functions in Bash?
Yes, you can use the shift command within functions to manipulate the positional parameters passed to that function.