How to Check if a Command Exists in Bash
-
Use the
command -v
Command to Check if a Command Exists in Bash -
Use the
type
Command to Check if a Command Exists in Bash -
Use the
hash
Command to Check if a Command Exists in Bash -
Use the
test
Command to Check if a Command Exists in Bash
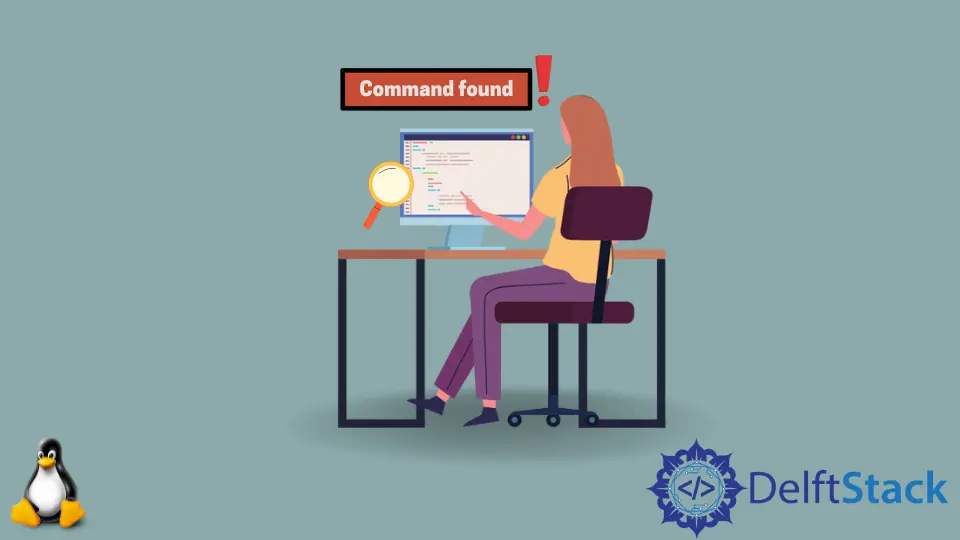
There may be a need to validate whether a command, program, or file exists using Bash Scripting or programming.
This article will enlist multiple methods to fulfill that need.
We can use different built-in commands in Bash that allow us to check if a command exists or not. The use of these commands is demonstrated below.
Use the command -v
Command to Check if a Command Exists in Bash
The command -v
is a built-in function in all POSIX systems and Bash. This function checks if a command exists as it returns the valid path for that command if it does exist and returns NULL
if it does not.
For example:
command -v ls
Output:
/bin/ls
The command -v
can also be used safely in Bash scripts to check the existence of a command with if
conditions, which is demonstrated below.
if ! [ -x "$(command -v npm)" ]; then
echo 'Error: npm is not installed.' >&2
exit 1
fi
The above code will check if npm
is installed, i.e., exists within the user directory and if it is executable. If npm
is not found on Path
, the above code will raise an exception and terminate.
The template above can be used to check for the existence of any program/command/utility by using the name of that program/command/utility.
Use the type
Command to Check if a Command Exists in Bash
The type
command is a very useful built-in command that gives information about the entity it uses. It can be used with commands, files, keywords, shell built-ins, etc.
The use of the type
command is shown below.
type command
Output:
command is a shell builtin
More specifically, for our use case, we can use the type
command with the -p
option to get the path of a file or executable. The use of this is demonstrated below.
type -p npm
Output:
/usr/local/bin/npm
Since npm
was installed on our system, type -p
has returned its valid path. It is important to note that if the type
command (without any flags) is used with an entity that does not exist, it will raise an error; however, type -p
in the same case will return NULL
instead.
This behavior is demonstrated below.
type yarn
Output:
bash: type: yarn: not found
An error is returned as yarn
is not installed on our system. However, type -p yarn
returns no output; depending on how and why the existence of a program needs to be checked, the -p
flag can be used or omitted.
Use the hash
Command to Check if a Command Exists in Bash
The hash
command works similarly to the type
command. However, it exits successfully if the command is not found.
Furthermore, it has the added benefit of hashing the queried command resulting in a faster lookup.
The syntax for the command is as follows.
hash -t ls
In this case, the queries command is ls
. Depending on your system, the output will be something like the below.
/usr/bin/ls
This is the file’s location that runs when the command is called. If the command is not found, e.g., when the query is something like: hash -t nothing
, then the output will be like the below.
bash: hash: nothing: not found
The output is descriptive when the command is found and gives the added benefit of hashing the command for a faster search next time.
Use the test
Command to Check if a Command Exists in Bash
test
is a built-in shell command that is mainly used for comparisons and conditional statements; it can also be used to check for the existence of files and directories; it is important to note that test
works only if the complete, valid path is provided of the file or directory you want to check.
There are a lot of option flags with the test
command that relates to files and directories, and a list of these option flags is shown below.
It should be noted that these flags only return true
if the file exists and the special condition is met. Below, you can find the special conditions listed.
-b FILE
-True
if the file is a special block file.-c FILE
-True
if the file is a special character file.-d FILE
-True
if it is a directory.-e FILE
-True
if it is a file, regardless of type.-f FILE
-True
only if it is a regular file (e.g., not a directory or device).-G FILE
-True
if the file has the same group as the user executing the command.-h FILE
-True
if it is a symbolic link.-g FILE
-True
if it has theset-group-id
(sgid
) flag set.-k FILE
-True
if it has a sticky bit flag set.-L FILE
-True
if it is a symbolic link.-O FILE
-True
if it is owned by the user running the command.-p FILE
-True
if it is a pipe.-r FILE
-True
if it is readable.-S FILE
-True
if it is a socket.-s FILE
-True
if it has some nonzero size.-u FILE
-True
if theset-user-id
(suid
) flag is set.-w FILE
-True
if it is writable.-x FILE
-True
if it is executable.
In this example, FILE
denotes the complete, valid path of a file that the user wants to check.
The use of a test
to check for the existence of a file is shown below.
test -e etc/random.txt && echo "FILE exists."
The above code statement will check for the existence of the file etc/random.txt
and output FILE exists
if the test statement returns true
.