Multiple if Conditions in Bash Script
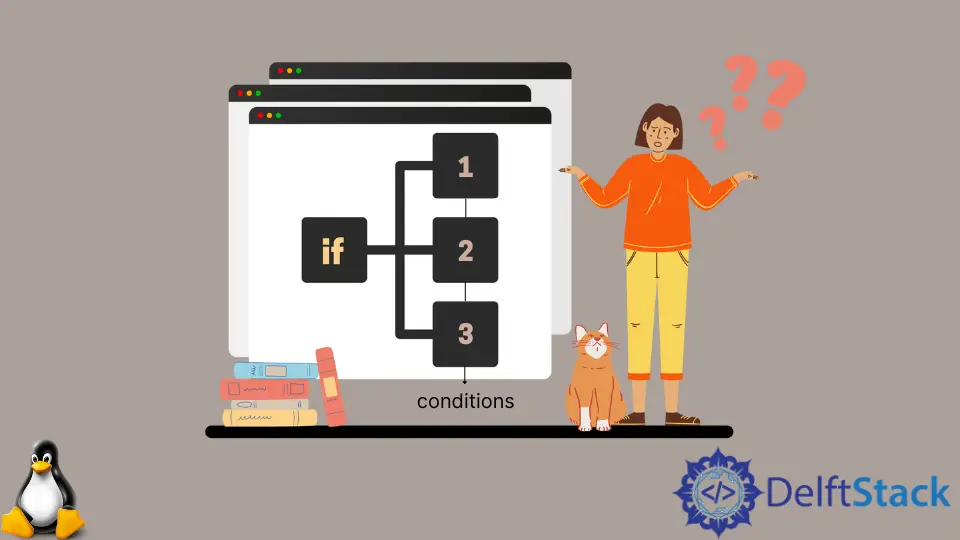
This programming tutorial will discuss conditional structures in bash, especially the if
condition with single and multiple conditions.
Introduction to Bash Programming
Bash is a simple command-line interpreter in UNIX and Linux operating systems. This interpreter allows us to run some commands using the command line, and these commands can be collectively run by typing them in a file known as script.
The shell script is nothing but a collection of bash commands that can run individually on the bash or be written in a script file, and then later, that script file can be executed by bash. The results in both cases will remain the same.
Bash is a critical tool for developers and is usually used to automate repetitive tasks that are required to be executed frequently. Bash programming is easy to learn and requires only fundamental knowledge of bash commands.
Write a Bash Script
Bash scripts are written in a file with the extension .script
. Although Linux is an extension-free operating system, it is a good programming convention to have this extension to your bash scripts.
The following command’s function is to create a new file.
vim myscript.sh
After this command executes, a file with the name myscript.sh
will be created and opened in the vim
editor. Below is the first line of every bash script.
#!/bin/bash
This line is known as shebang
, written to tell the operating system the location of the bash interpreter. After this line, the actual code of the bash script starts.
Conditional Statements in Bash Script
In a Bash script, we can have multiple types of conditional statements like:
if
statementsif .. then.. else
statementif .. elif
statements- Nested
if
statements case
statements
We’ll discuss the if
statements with single and multiple conditions. Before moving towards the if
statement, let’s see some commonly used conditional operators in if
statements.
Operator Symbol | Description |
---|---|
-eq |
It returns true if the two numbers are equal. |
-lt |
It returns true if a number is less than another number. |
-gt |
It returns true if a number is larger than another number. |
== |
It returns true if the two strings are equal. |
!= |
It returns true if the two strings are not equal. |
! |
It negates the expression with which it is used. |
Use the if
Statement With One Condition
Syntax:
if [ condition-statement ];
then
Commands..
fi
Let us look at an example bash script that uses if
conditions.
Script:
#!/bin/bash
echo "Enter your marks out of 100: "
read marks
if [ $marks -gt 100 ]; then
printf "You have entered incorrect marks: $marks\n "
fi
Output:
Use the if
Statement With Multiple Conditions
In the previous example, we used a single condition. We can also apply multiple conditions and separate them using logical operators AND
or OR
operators.
Let us look at the example below.
Script:
#!/bin/bash
echo "Enter your marks out of 100: "
read marks
if [[ $marks -gt 100 && $marks -lt 0 ]]; then
printf "You have entered incorrect marks: $marks\n "
fi
Output:
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn