The if not Condition in Bash
-
the
if not
Condition in the Case of Integers in Bash -
the
if not
Condition in Case of Strings in Bash -
Bash
if not
Condition in the Expression
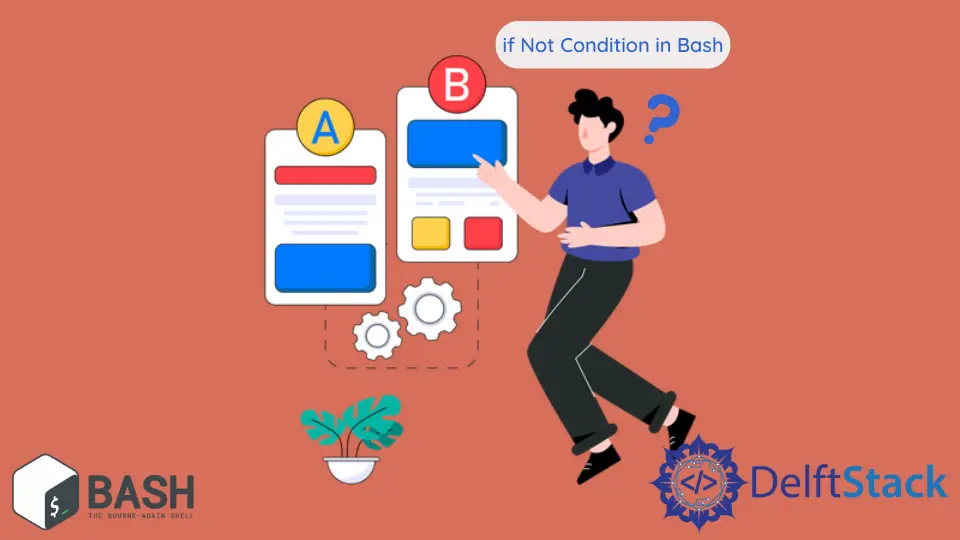
In bash, if the code of the command list is true, then if | then
statements execute one or more commands after the word then
. If the check returns false, it executes else
, if the condition requires it. We will learn to make the if not
condition here.
the if not
Condition in the Case of Integers in Bash
At the heart of the if not
condition for integer comparisons lies the -ne
operator. This operator stands for not equal
and is used to assess the inequality between two integer variables.
When the -ne
operator is employed, it evaluates whether the values of the two variables are indeed not equal.
If the comparison holds true, the subsequent code block under the then
clause is executed; otherwise, the execution proceeds to the next set of instructions.
We can use -ne
to check the inequality between two integer variables. Let’s look at an example.
#!/bin/bash
x=5
y=4
if [[ $x -ne $y ]];
then
echo "Not equal"
fi
Output:
Not equal
In this example, we have two integer variables, x
and y
, assigned the values 5 and 4, respectively.
The if
statement employs the -ne
operator to ascertain whether x
is not equal to y
.
Since 5 is indeed not equal to 4, the condition evaluates to true, leading to the execution of the echo
statement within the then
clause.
the if not
Condition in Case of Strings in Bash
We can use the !=
operator to compare two strings.
#!/bin/bash
sone=Bin
stwo=Bash
if [[ $sone != $stwo ]];
then
echo "Not equal"
fi
In this example, we have two string variables, sone
and stwo
, assigned the values Bin
and Bash
, respectively.
The if
statement employs the !=
operator to determine whether sone
is not equal to stwo
.
Since the two strings are indeed not equal, the condition evaluates to true, resulting in the execution of the echo
statement within the then
clause.
Consequently, the output of the script is:
Not equal
Codes Explanation
- Script Initialization: The script begins with the shebang (
#!/bin/bash
), indicating that the script should be interpreted using the Bash shell. - Variable Declaration: We define two string variables,
sone
andstwo
, and assign them values ofBin
andBash
, respectively. - Conditional Evaluation: The
if
statement is used to evaluate the condition$sone != $stwo
. The!=
operator checks whether the values ofsone
andstwo
are not equal. - Conditional Block: As the condition holds true (
"Bin"
is not equal to"Bash"
), the code block within thethen
clause is executed. - Output Generation: The
echo
statement within the conditional block outputs the messageNot equal
.
Bash if not
Condition in the Expression
Central to the if not
condition for expressions is the !
operator. When used outside of double square brackets [[ ]]
, this operator inverts the logical outcome of an expression.
By doing so, it provides a means to check for the absence or negation of a particular condition. This feature enables you to craft scripts that take distinct paths based on whether the condition is not satisfied.
#!/bin/bash
n=4
if ! [[ $n -eq 0 ]];
then
echo "Not equal to 0"
fi
Output:
Not equal to 0
In this example, the script begins by declaring a variable n
and assigning it the value 4
.
The if
statement follows, utilizing the !
operator outside double square brackets to evaluate whether $n
is not equal to 0
.
As 4
is indeed not equal to 0
, the condition evaluates to true, and the echo
statement within the then
clause is executed.
Output:
Not equal to 0
Code Exploration
- Script Initialization: The script commences with the shebang (
#!/bin/bash
), indicating that the script should be interpreted using the Bash shell. - Variable Declaration: We declare a variable
n
and set its value to4
. - Conditional Evaluation: The
if
statement is used to evaluate the condition! [[ $n -eq 0 ]]
. The!
operator negates the logical outcome of the expression within the double square brackets. - Conditional Block: Since the condition holds true (
4
is not equal to0
), the code block within thethen
clause is executed. - Output Generation: The
echo
statement within the conditional block outputs the messageNot equal to 0
.