How to Use while Loop in Bash
-
Syntax:
while
Loop in Bash -
Example:
while
Loop in Bash -
Example: Infinite
while
Loop in Bash -
Example:
while
Loop in Bash Withbreak
Statement -
Example:
while
Loop in Bash Withcontinue
Statement
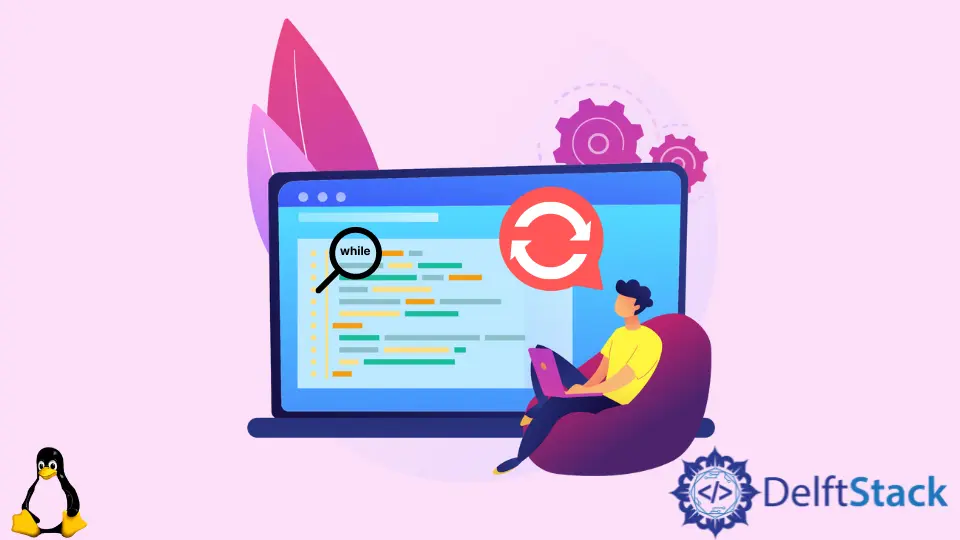
while
loop is one of the most widely used loop structures in almost every programming language. It is used when we don’t know the number of times we need to run a loop. We can specify a condition for the while
loop, and the statements in the loop are executed until the condition becomes false.
Syntax: while
Loop in Bash
while [condition]
do
command-1
command-2
...
...
command-n
done
Here, the condition
represents the condition that needs to be checked every time before executing commands in the loop. If the condition
is true, we execute the statements in the loop. If the condition
is false, we exit out of the loop. The statements from command-1
to command-n
are statements executed in a loop until the condition
becomes false.
Example: while
Loop in Bash
#!/bin/bash
num=5
while [ $num -ge 0 ]
do
echo $num
((num--))
done
Output:
5
4
3
2
1
0
Here, initially, num
is set to 5. We keep printing num
in the terminal and decrementing num
by 1 in a loop as long as the num
value is greater than or equal to 0.
Example: Infinite while
Loop in Bash
#!/bin/bash
while true
do
echo "This is an infinite while loop. Press CTRL + C to exit out of the loop."
sleep 0.5
done
Output:
This is an infinite while loop. Press CTRL + C to exit out of the loop.
This is an infinite while loop. Press CTRL + C to exit out of the loop.
This is an infinite while loop. Press CTRL + C to exit out of the loop.
^C
It is an infinite while
loop that prints This is an infinite
while loop. Press <kbd>Ctrl</kbd>+<kbd>C</kbd> to exit out of the loop.
in every 0.5 seconds. To exit out of the loop, we can Press Ctrl+C.
Example: while
Loop in Bash With break
Statement
#!/bin/bash
num=5
while [ $num -ge 0 ]
do
echo $num
((num--))
if [[ "$num" == '3' ]]; then
echo "Exit out of loop due to break"
break
fi
done
Output:
5
4
Exit out of loop due to break
In the above program, num
is initialized as 5. The loop is executed as long as num
is greater than or equal to 0. But as we have a break
statement in the loop when num
is 3. So, we exit out of the loop as the value of num
becomes 3.
Example: while
Loop in Bash With continue
Statement
#!/bin/bash
num=6
while [ $num -ge 1 ]
do
((num--))
if [[ "$num" == '3' ]]; then
echo "Ignore a step due to continue"
continue
fi
echo $num
done
Output:
5
4
Ignore a step due to continue
2
1
0
In the above program, num
is initialized as 6. In the loop, we first decrease num
by 1 and then print the num
latest value. The loop is executed as long as the num
value is greater than or equal to 1. When num
becomes 3, the script does not print the value of num
as we have the continue
statement when num
is 3.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn