如何在 Bash 中使用 While 迴圈
Suraj Joshi
2023年1月30日
-
Bash 中的
while
迴圈語法 -
例子:Bash 中的
while
迴圈 -
示例:Bash 中的無限
while
迴圈 -
示例:帶有
break
語句的 Bash 中的 while 迴圈 -
示例:帶有
continue
語句的 Bash 中的while
迴圈
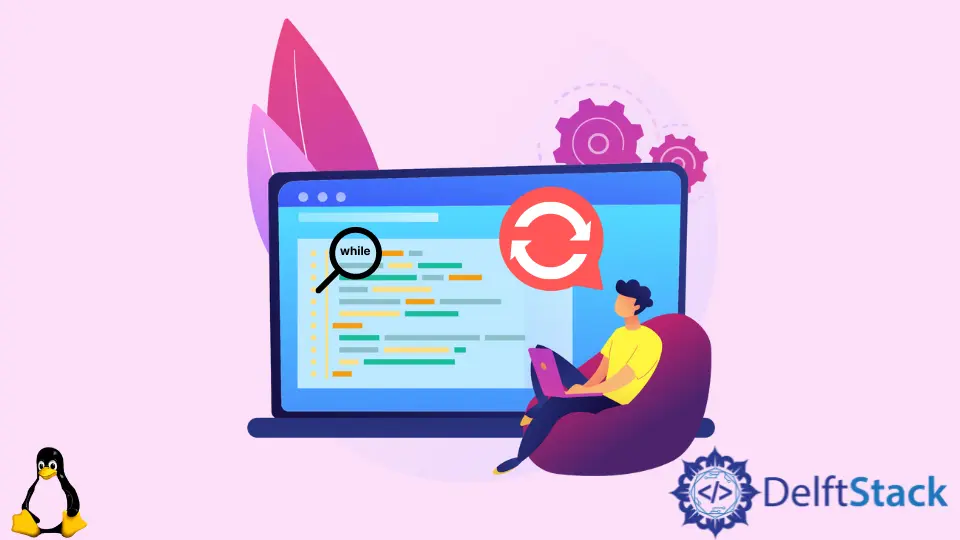
while
迴圈幾乎是所有程式語言中使用最廣泛的迴圈結構之一。當我們不知道需要執行一個迴圈的次數時,就會用到它。我們可以為 while
迴圈指定一個條件,迴圈中的語句會被執行,直到條件變為假。
Bash 中的 while
迴圈語法
while [condition]
do
command-1
command-2
...
...
command-n
done
這裡,condition
代表每次在迴圈中執行命令前需要檢查的條件。如果 condition
為真,我們就執行迴圈中的語句。如果 condition
為假,我們退出迴圈。從 command-1
到 command-n
的語句是在迴圈中執行的語句,直到 condition
變為 false
。
例子:Bash 中的 while
迴圈
#!/bin/bash
num=5
while [ $num -ge 0 ]
do
echo $num
((num--))
done
輸出:
5
4
3
2
1
0
這裡,最初,num
被設定為 5。只要 num
的值大於或等於 0,我們就在終端列印 num
,並在迴圈中把 num
遞減 1。
示例:Bash 中的無限 while
迴圈
#!/bin/bash
while true
do
echo "This is an infinite while loop. Press CTRL + C to exit out of the loop."
sleep 0.5
done
輸出:
This is an infinite while loop. Press CTRL + C to exit out of the loop.
This is an infinite while loop. Press CTRL + C to exit out of the loop.
This is an infinite while loop. Press CTRL + C to exit out of the loop.
^C
這是一個無限迴圈,每 0.5 秒列印出 This is an infinite while loop. Press <kbd>Ctrl</kbd>+<kbd>C</kbd> to exit out of the loop.
。要退出迴圈,我們可以按 CTRL+C。
示例:帶有 break
語句的 Bash 中的 while 迴圈
#!/bin/bash
num=5
while [ $num -ge 0 ]
do
echo $num
((num--))
if [[ "$num" == '3' ]]; then
echo "Exit out of loop due to break"
break
fi
done
輸出:
5
4
Exit out of loop due to break
在上面的程式中,num
初始化為 5,只要 num
大於或等於 0,迴圈就會被執行,但由於迴圈中的 break
語句在 num
為 3 時,所以當 num
的值變成 3 時,我們就退出迴圈。
示例:帶有 continue
語句的 Bash 中的 while
迴圈
#!/bin/bash
num=6
while [ $num -ge 1 ]
do
((num--))
if [[ "$num" == '3' ]]; then
echo "Ignore a step due to continue"
continue
fi
echo $num
done
輸出:
5
4
Ignore a step due to continue
2
1
0
在上面的程式中,num
初始化為 6,在迴圈中,我們首先將 num
減少 1,然後列印 num
的最新值。只要 num
值大於或等於 1,迴圈就會執行。當 num
變成 3 時,指令碼不列印 num
的值,因為當 num
為 3 時,我們有 continue
語句。
作者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn