How to Read a File Line by Line Using Bash
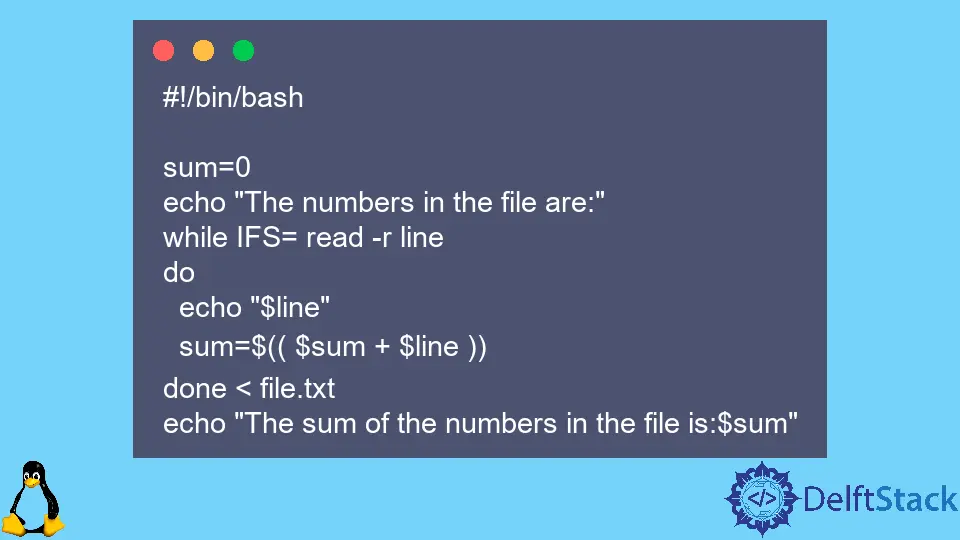
We may face several situations in Bash, where we need to process the data stored in a file line by line. In such cases, we need to read the contents of the file. We use the read
command in Bash to read a file line by line.
Read the File Line by Line in Bash
Syntax
while IFS= read -r line
do
echo "$line"
done < file_name
It reads the content of the file file_name
one line at a time and prints the lines one by one in the terminal. The loop is executed until we reach the end of the file. The IFS
is set to the null string, which helps to retain leading and trailing whitespaces.
Alternatively, the above command can also be replaced by the following command within a single line:
while IFS= read -r line; do echo $line; done < file_name
Example: Read the File Line by Line in Bash
In the example, we will read the file file.txt
, which contains numbers in each line and then find the sum of all numbers in the file.
Content of file.txt
1
5
6
7
8
10
#!/bin/bash
sum=0
echo "The numbers in the file are:"
while IFS= read -r line
do
echo "$line"
sum=$(( $sum + $line ))
done < file.txt
echo "The sum of the numbers in the file is:$sum"
Output:
The numbers in the file are:
1
5
6
7
8
The sum of the numbers in the file is:27
It reads the numbers line by line from a file named file.txt
and then sums up all those numbers and finally echoes the sum.
Example: Set Fields in Files to Variables
We can set fields in the file to variables by passing multiple variables to the read
command, which will separate fields within a line based on the value of IFS
.
Content of file.txt
Rohit-10
Harish-30
Manish-50
Kapil-10
Anish-20
#!/bin/bash
while IFS=- read -r name earnings
do
echo "$name" has made earnings of "$earnings" pounds today!
done < file.txt
Output:
Rohit has made earnings of 10 pounds today!
Harish has made earnings of 30 pounds today!
Manish has made earnings of 50 pounds today!
Kapil has made earnings of 10 pounds today!
Here, each line in the file is divided into two segments as we have passed two variables to the read
command. The first segment will be assigned to the name
variable, which extends from the beginning of the line until the first -
, and the remaining portion will be assigned to the earnings
variable.
Alternative Methods to Read Files in Bash
#!/bin/bash
while IFS=- read -r name earnings
do
echo "$name" has made earnings of "$earnings" pounds today!
done < <(cat file.txt )
Output:
Rohit has made earnings of 10 pounds today!
Harish has made earnings of 30 pounds today!
Manish has made earnings of 50 pounds today!
Kapil has made earnings of 10 pounds today!
Here, the filename file.txt
is passed to the program as an output of the cat
command.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn