如何使用 Bash 逐行读取文件
Suraj Joshi
2020年10月15日
Linux
Bash
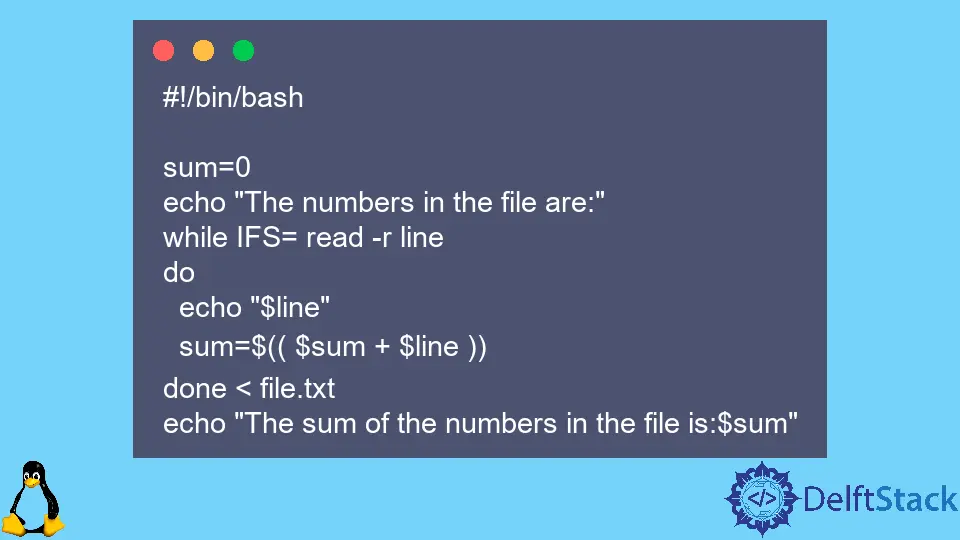
在 Bash 中,我们可能会遇到几种情况,需要逐行处理存储在文件中的数据。在这种情况下,我们需要读取文件的内容。我们使用 Bash 中的 read
命令来逐行读取文件。
在 Bash 中逐行读取文件
语法
while IFS= read -r line
do
echo "$line"
done < file_name
它一行一行地读取文件 file_name
的内容,并在终端上逐行打印。循环执行,直到我们到达文件的终点。IFS
被设置为空字符串,这有助于保留前导和尾部的空白。
另外,上面的命令也可以用下面的命令代替。
while IFS= read -r line; do echo $line; done < file_name
示例:在 Bash 中逐行读取文件
在本例中,我们将读取文件 file.txt
,其中每行都包含数字,然后找出文件中所有数字的总和。
file.txt
的内容
1
5
6
7
8
10
#!/bin/bash
sum=0
echo "The numbers in the file are:"
while IFS= read -r line
do
echo "$line"
sum=$(( $sum + $line ))
done < file.txt
echo "The sum of the numbers in the file is:$sum"
输出:
The numbers in the file are:
1
5
6
7
8
The sum of the numbers in the file is:27
它从名为 file.txt
的文件中逐行读取数字,然后将所有这些数字相加,最后回声输出。
示例:将文件中的字段设置为变量
我们可以将文件中的字段设置为变量,将多个变量传给 read
命令,命令会根据 IFS
的值来分隔一行中的字段。
file.txt
的内容
Rohit-10
Harish-30
Manish-50
Kapil-10
Anish-20
#!/bin/bash
while IFS=- read -r name earnings
do
echo "$name" has made earnings of "$earnings" pounds today!
done < file.txt
输出:
Rohit has made earnings of 10 pounds today!
Harish has made earnings of 30 pounds today!
Manish has made earnings of 50 pounds today!
Kapil has made earnings of 10 pounds today!
这里,文件中的每一行都被分成两段,因为我们已经向 read
命令传递了两个变量。第一段将分配给 name
变量,它从行的开始一直延伸到第一个 -
,剩下的部分将分配给 earnings
变量。
在 Bash 中读取文件的其他方法
#!/bin/bash
while IFS=- read -r name earnings
do
echo "$name" has made earnings of "$earnings" pounds today!
done < <(cat file.txt )
输出:
Rohit has made earnings of 10 pounds today!
Harish has made earnings of 30 pounds today!
Manish has made earnings of 50 pounds today!
Kapil has made earnings of 10 pounds today!
这里,文件名 file.txt
作为 cat
命令的输出传给程序。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn