How to Echo to Stderr in Bash
-
Use the
>&2
Operator to Echo tostderr
in Bash -
Use
echo
With File Descriptor Redirection to Echo tostderr
in Bash -
Use
printf
With File Descriptor Redirection to Echo tostderr
in Bash -
Use
echo
With Process Substitution to Echo tostderr
in Bash -
Use the
logger -s
Command to Echo tostderr
in Bash -
Use the
/dev/stderr
File to Echo tostderr
in Bash -
Use the
/proc/self/fd/2
File Descriptor to Echo tostderr
in Bash - Conclusion
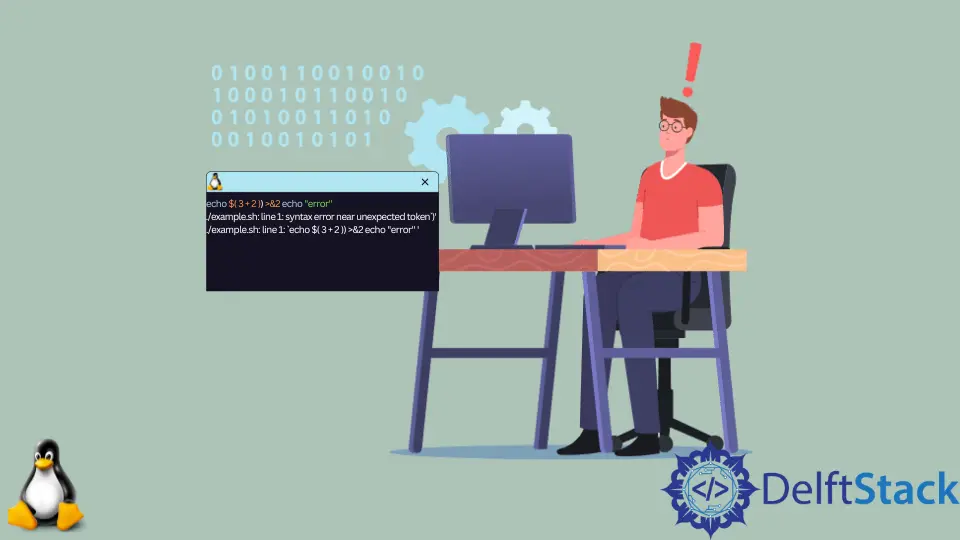
In Bash scripting, the standard output (stdout
) is typically used to display regular program output, while the standard error (stderr
) is reserved for displaying error messages. However, there are scenarios where you may want to print messages directly to stderr
.
The Linux environment identifies every file object with its description, known as FD
. It is a positive integer value that identifies the open file session. For stderr
, the value of the file descriptor is 2
.
This article will show different methods to redirect the echo
output to stderr
in Bash.
Use the >&2
Operator to Echo to stderr
in Bash
The command stderr
is mainly used to keep a record of errors during the execution of any command.
Syntax:
Your command here 2>error.log
In the syntax above, you can find a symbol 2>
and a file named error.log
. Now the 2>
indicates the value of FILE DESCRIPTOR
, representing the identity of stderr
.
In our case, the FILE DESCRIPTOR
value is 2
. We provided the file name for the log file generated during the command execution.
You can also echo
the stderr
message by following the below syntax.
Your command here >&2 echo "error"
Let’s see an example to make the topic easier. Suppose we have created an error command and want to echo
the output error message.
echo $( 3 + 2 )) >&2 echo "error"
We intentionally made an error in the code above to understand how it works. We removed one of the parentheses here, which can be an error.
After that, we show that error with the echo
.
Output:
main.bash: line 6: syntax error near unexpected token `)'
main.bash: line 6: `echo $( 3 + 2 )) >&2 echo "error"'
Now we correct the code like below and run the command again.
echo $(( 3 + 2 )) >&2 "No error has been found."
Output:
5 No error has been found.
Use echo
With File Descriptor Redirection to Echo to stderr
in Bash
This method involves temporarily redirecting stdout
to stderr
.
echo "This is regular output"
exec 1>&2
echo "This is an error message"
echo "This is regular output"
: This line prints"This is regular output"
tostdout
.exec 1>&2
: This line redirectsstdout
(file descriptor1
) tostderr
(file descriptor2
).echo "This is an error message"
: Sincestdout
is now redirected tostderr
, this line prints"This is an error message"
tostderr
.
Use printf
With File Descriptor Redirection to Echo to stderr
in Bash
This method utilizes the printf
command along with file descriptor redirection.
echo "This is regular output"
printf "This is an error message\n" 1>&2
echo "This is regular output"
: This line prints"This is regular output"
tostdout
.printf "This is an error message\n" 1>&2
: Theprintf
command is used to format and print the error message. The1>&2
redirectsstdout
tostderr
.
Use echo
With Process Substitution to Echo to stderr
in Bash
Process substitution allows you to use a command’s output as a file.
echo "This is regular output"
echo "This is an error message" > >(cat 1>&2)
echo "This is regular output"
: This line prints"This is regular output"
tostdout
.echo "This is an error message" > >(cat 1>&2)
: The> >(cat 1>&2)
construct sends the output of theecho
command to thecat
command, which in turn redirects it tostderr
(1>&2
).
Use the logger -s
Command to Echo to stderr
in Bash
The logger -s
command is a convenient way to send output to the system log, which typically goes to stderr
.
Here’s an example code:
echo "This is regular output"
logger -s "This is an error message"
echo "This is regular output"
: This line prints"This is regular output"
tostdout
.logger -s "This is an error message"
: Thelogger -s
command logs the message"This is an error message"
. The-s
flag ensures that the message is sent tostderr
.
When you run this script, the error message will be logged to the system log, typically directed to stderr
.
Use the /dev/stderr
File to Echo to stderr
in Bash
You can use the /dev/stderr
special file to redirect output to stderr
. Here’s an example code:
echo "This is regular output"
echo "This is an error message" > /dev/stderr
echo "This is regular output"
: This line prints"This is regular output"
tostdout
.echo "This is an error message" > /dev/stderr
: Here, the> /dev/stderr
part redirects the output of theecho
command to the/dev/stderr
special file, which representsstderr
.
When you run this script, the error message will be sent directly to stderr
.
Use the /proc/self/fd/2
File Descriptor to Echo to stderr
in Bash
You can use the /proc/self/fd/2
file descriptor to redirect output to stderr
. Here’s an example code:
echo "This is regular output"
echo "This is an error message" > /proc/self/fd/2
echo "This is regular output"
: This line prints"This is regular output"
tostdout
.echo "This is an error message" > /proc/self/fd/2
: Here, the> /proc/self/fd/2
part redirects the output of theecho
command to the file descriptor2
, which representsstderr
.
When you run this script, the error message will be sent directly to stderr
.
Conclusion
This article explored seven methods to echo
to stderr
in Bash. Each method has its strengths and use cases, so choose the one that best suits your application.
Understanding these methods will give you greater flexibility in handling error messages in your Bash scripts.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn