How to Echo Tab Characters in Bash Script
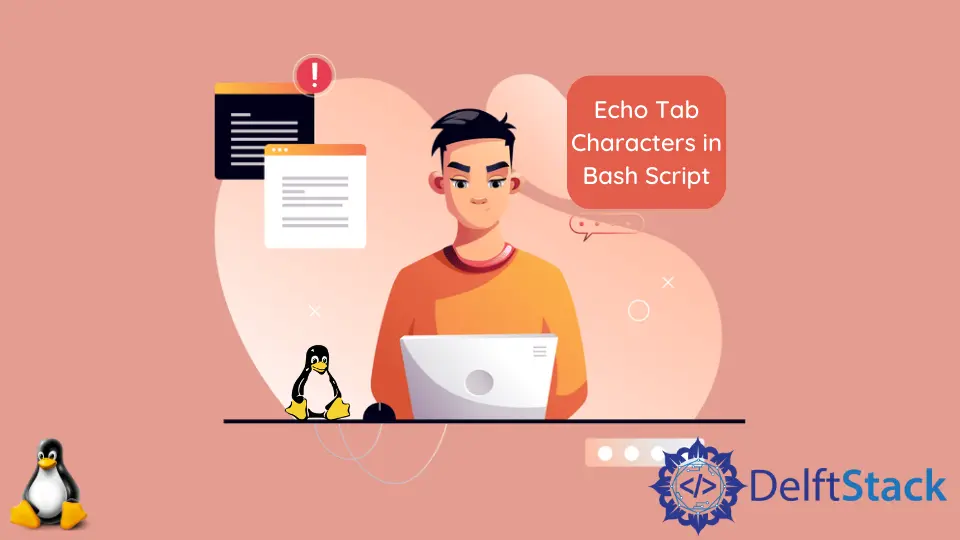
This write-up explains how to echo
one or multiple tab
characters when using a Bash script.
Echo Tab Characters in Bash Script
The echo
command is simple: it prints whatever is passed to the terminal. Normally, if you save a variable as something like:
example=' 'testing
If you try to print out this variable with the command echo '['$example']'
, you will get the following output.
[ testing]
This is not what we have input or saved to the variable. In the above example, we used the tab
character twice, yet all we see is a single space.
It should be noted that this behavior is not consistent across all shells. For example, running the same script in the zsh
shell will result in the following output, which we expect to get.
[ testing]
Coming back to Bash scripts, we have a few ways around this.
Use the -e
Flag
The -e
flag means enable interpretation of backslash escapes
. If you were writing the same example above, you would write it as follows.
example='\t\t'testing
echo -e '['$example']'
Which gives the output:
[ testing]
The \t
part represents a tab. Hence, as we wanted to introduce two tabs, we wrote it as \t\t
.
Once the -e
flag is passed, the \t
symbol is interpreted as a tab and displayed as required.
If the -e
flag is not used, as in the following case, then the \t
will be read as literal strings and will not be processed as tabs.
example='\t\t'testing
echo '['$example']'
Which gives the output:
[\t\ttesting]
It should be noted that passing the flag is not POSIX; hence, this cannot be used portably, such as in make
, in which the flag is not implemented. Hence, we will discuss a few alternatives, including the almost universal printf
.
Of course, this part slightly deviates from what we started with, as we will abandon echo
entirely.
Use Double Quotes
This is arguably the simplest solution and does not require any explanation other than its syntax. You would write the command as follows.
example=' 'testing
echo "[$example]"
Which would give the result:
[ testing]
Use printf
Instead
This is the most portable of the three solutions and the most recommended. echo
has many implementations and versions, but printf
is mostly the same.
Even if it is implemented separately anywhere, it is implemented very similarly. We can see this as any variation of the above methods would result in the same output when called using zsh
but different when called using bash
.
The syntax for using printf
is defined below.
example=' 'x
printf '%s\n' "[$example]"
Which gives the output:
[ x]
As a general recommendation, echo
works fine as long as the output needed is a simple text like hello
, but for anything complex, using echo
can get tricky because of how different its behavior can be across shells. In all such cases (special characters, etc.), it is recommended to use printf
instead.
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn