How to Handle Exceptions in Kotlin
- Concept of Exception in Kotlin
- Handle Unchecked Exceptions in Kotlin
- Create a Custom Exception in Kotlin
-
Handle Exceptions Using the
@Throws
Annotation in Kotlin
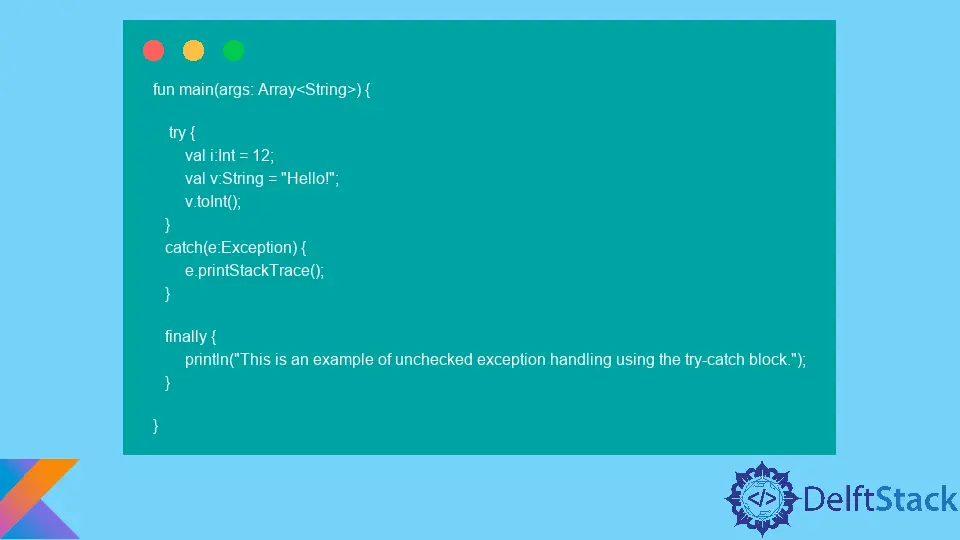
Exception handling is a fundamental concept of most programming languages. It helps handle exceptions so that the code executes without any problem.
Even Kotlin allows exception handling with @Throws
annotation. This article will look at how we can use the @Throws
annotation to handle exceptions in Kotlin.
But before getting into that, let’s look at the basic concept of exceptions in Kotlin.
Concept of Exception in Kotlin
Kotlin exceptions are similar to Java exceptions. They are all the descendants of the Throwable
class in Kotlin; however, there are a few differences, too.
Unlike Java, Kotlin does not have any checked exceptions. So, there are only unchecked or runtime exceptions in Kotlin.
Also, Kotlin allows creating custom exceptions. Hence, we can make our own exception handling code to prevent any runtime error.
Handle Unchecked Exceptions in Kotlin
As previously stated, unchecked exceptions are those that occur during the runtime. All the Java unchecked exceptions, including ArithmeticException
, NullPointerException
, NumberFormatException
, etc., are examples of unchecked exceptions in Kotlin.
We can handle an unchecked exception in Kotlin using the try-catch
block and the finally
keyword.
Here’s an example code to demonstrate unchecked exceptions.
fun main(args: Array<String>) {
try {
val i:Int = 12;
val v:String = "Hello!";
v.toInt();
}
catch(e:Exception) {
e.printStackTrace();
}
finally {
println("This is an example of unchecked exception handling using the try-catch block.");
}
}
Output:
Click here to check the demo of the example code.
Create a Custom Exception in Kotlin
We can also create custom exceptions in Kotlin using the throw
keyword. Let’s create a code where we declare an integer variable.
We will then check if the number is equal to or above 18. If it is, we will print a message saying, "You are eligible to vote."
; otherwise, we will throw a custom error message.
fun main(args: Array<String>) {
val v:Int;
v = 16;
if(v >= 18)
{
println("Welcome!! You are eligible to vote.")
}
else
{
//throwing custom exception using the throw keyword
throw customExceptionExample("Sorry! You have to wait to cast a vote.")
}
}
//custom exception class
class customExceptionExample(message: String) : Exception(message)
Output:
Click here to check the demo of the example code.
Handle Exceptions Using the @Throws
Annotation in Kotlin
Although there are no checked exceptions, we can still handle them in Kotlin. We can do that by using the Kotlin @Throws
exception annotation.
The @Throws
annotation is helpful for Java interoperability. Hence, if there are any checked exception codes that we need to convert to Java, we can use the @Throws
annotation for the JVM machine.
Here’s an example code to demonstrate the same.
import java.io.*
import kotlin.jvm.Throws
fun main(args: Array<String>) {
val va=0
var res=0
try {
res=va/0 // Since nothing is divisible by 0, it will throw an exception
} catch (excep: Exception) {
// While this is an Airthmetic exception,
// we will throw a NullPointerException using the function call
excep().throwJavaChecked()
}
}
class excep{
@Throws(NullPointerException::class)
fun throwJavaChecked() {
throw NullPointerException()
}
}
Output:
Click here to check the demo of the example code.
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn