在 Kotlin 中處理異常
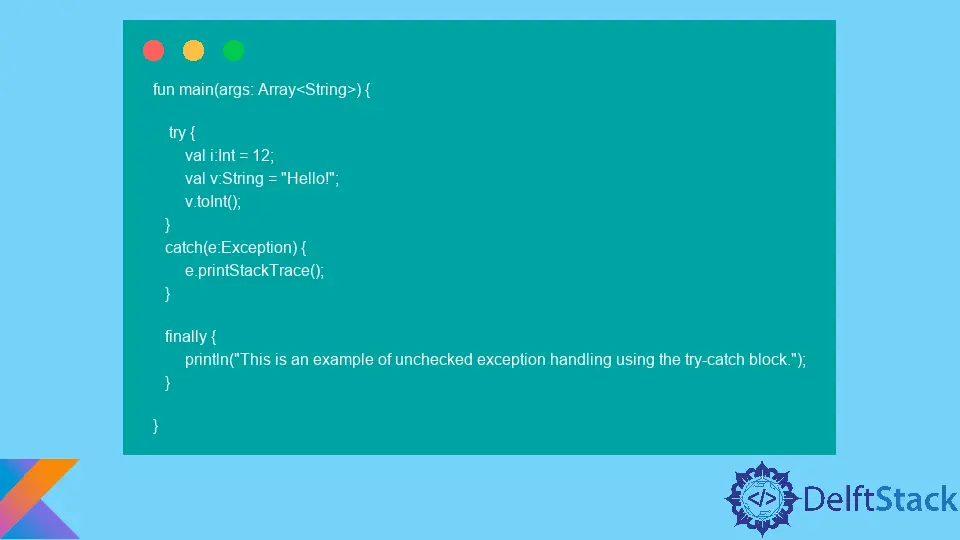
異常處理是大多數程式語言的基本概念。它有助於處理異常,以便程式碼執行沒有任何問題。
甚至 Kotlin 也允許使用 @Throws
註釋進行異常處理。本文將介紹如何使用 @Throws
註釋來處理 Kotlin 中的異常。
但在進入之前,讓我們先看看 Kotlin 中異常的基本概念。
Kotlin 中的異常概念
Kotlin 異常類似於 Java 異常。他們都是 Kotlin 中 Throwable
類的後代;但是,也有一些差異。
與 Java 不同,Kotlin 沒有任何檢查異常。因此,Kotlin 中只有未經檢查的或執行時異常。
此外,Kotlin 允許建立自定義異常。因此,我們可以編寫自己的異常處理程式碼來防止任何執行時錯誤。
在 Kotlin 中處理未經檢查的異常
如前所述,未經檢查的異常是在執行時發生的異常。所有 Java 未檢查異常,包括 ArithmeticException
、NullPointerException
、NumberFormatException
等,都是 Kotlin 中未檢查異常的示例。
我們可以使用 try-catch
塊和 finally
關鍵字在 Kotlin 中處理未經檢查的異常。
這是演示未經檢查的異常的示例程式碼。
fun main(args: Array<String>) {
try {
val i:Int = 12;
val v:String = "Hello!";
v.toInt();
}
catch(e:Exception) {
e.printStackTrace();
}
finally {
println("This is an example of unchecked exception handling using the try-catch block.");
}
}
輸出:
點選這裡檢視示例程式碼的演示。
在 Kotlin 中建立自定義異常
我們還可以使用 throw
關鍵字在 Kotlin 中建立自定義異常。讓我們建立一個宣告整數變數的程式碼。
然後我們將檢查該數字是否等於或大於 18。如果是,我們將列印一條訊息,說你有資格投票
;否則,我們將丟擲自定義錯誤訊息。
fun main(args: Array<String>) {
val v:Int;
v = 16;
if(v >= 18)
{
println("Welcome!! You are eligible to vote.")
}
else
{
//throwing custom exception using the throw keyword
throw customExceptionExample("Sorry! You have to wait to cast a vote.")
}
}
//custom exception class
class customExceptionExample(message: String) : Exception(message)
輸出:
點選這裡檢視示例程式碼的演示。
在 Kotlin 中使用 @Throws
註釋處理異常
雖然沒有檢查異常,但我們仍然可以在 Kotlin 中處理它們。我們可以通過使用 Kotlin @Throws
異常註釋來做到這一點。
@Throws
註釋有助於 Java 互操作性。因此,如果我們需要將任何已檢查的異常程式碼轉換為 Java,我們可以為 JVM 機器使用 @Throws
註釋。
這是一個示例程式碼來演示相同的內容。
import java.io.*
import kotlin.jvm.Throws
fun main(args: Array<String>) {
val va=0
var res=0
try {
res=va/0 // Since nothing is divisible by 0, it will throw an exception
} catch (excep: Exception) {
// While this is an Airthmetic exception,
// we will throw a NullPointerException using the function call
excep().throwJavaChecked()
}
}
class excep{
@Throws(NullPointerException::class)
fun throwJavaChecked() {
throw NullPointerException()
}
}
輸出:
點選這裡檢視示例程式碼的演示。
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn