How to Create and Use Static Variables in Kotlin
- Declare Static Variables in Kotlin
-
Use the
Companion
Object to Create Kotlin Static Variables -
Use the
@JvmStatic
Annotation to Create Kotlin Static Variables
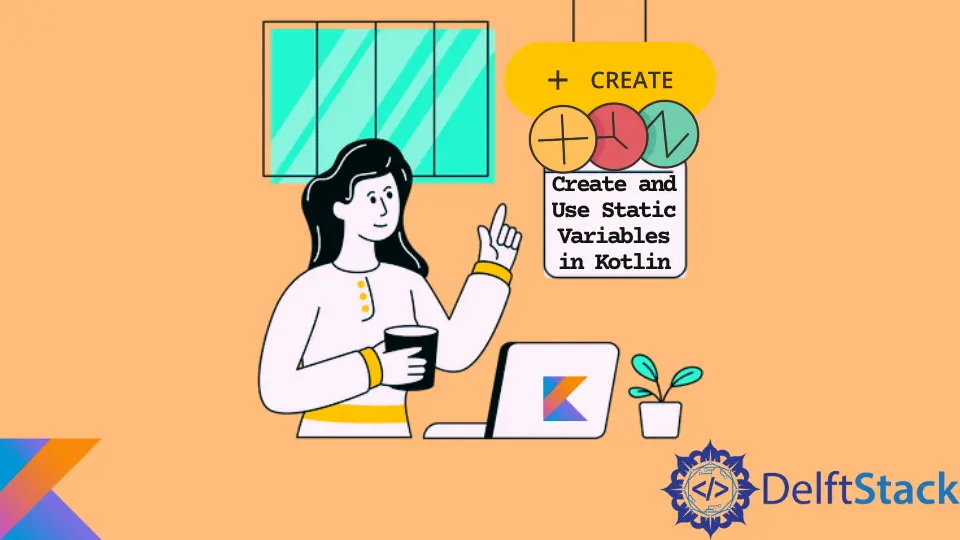
When we declare a variable as static in Java, we can use it in different classes without the need for creating objects. It means that the memory of a static variable is allocated only once.
Since JVM does not allocate memory multiple times to a static variable, they are used for efficient memory management; however, there is no static
keyword in Kotlin. So, how do we declare static variables in Kotlin?
This article introduces how we can implement the concept of static variables in Kotlin.
Declare Static Variables in Kotlin
While Kotlin does not have the static
keyword, we can still achieve the same memory management efficiency using:
- the
Companion
object - the
@JvmStatic
annotation
We will go through both methods and see examples for implementing Kotlin static variables.
Use the Companion
Object to Create Kotlin Static Variables
We can use the companion
keyword to create a companion
object to help achieve static variables functionality. We need to use the companion
keyword before the object.
These objects can access the private members of a class; hence, there is no need to allocate the memory twice. We can use the class’s name to access these members.
Here’s an example of using the companion
objects to achieve static functionality in Kotlin.
fun main(args: Array<String>) {
println("We are accessing a class variable without creating object.\n" + staticExample.privateVar)
}
class staticExample{
companion object {
val privateVar = "Hi, you are accessing a static variable."
}
}
Output:
Click here to check the demo of the example.
Use the @JvmStatic
Annotation to Create Kotlin Static Variables
While the companion
object members are like static variables in Java, they have a small difference. During the runtime, companion
objects are still members of real objects; hence, they can also implement interfaces.
But one way to declare static variables in Kotlin is by using the @JvmStatic
annotation. When we use the @JvmStatic
annotation while declaring variables, JVM considers them actual static variables.
Here’s an example of using the @JvmStatic
annotation to declare a variable.
fun main(args: Array<String>) {
println("We are accessing a class variable without creating object.\n" + staticExample.privateVar)
}
object staticExample{
@JvmStatic
val privateVar = "Hi, you are accessing a static variable."
}
Output:
Click here to check the demo of the example.
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn