Equivalent of Java Static Functions in Kotlin
- Kotlin’s Equivalent for Java’s Static Functions
-
Use the
Companion
Objects to Achieve Static Functionality in Kotlin -
Use the
@JvmStatic
Annotation to Achieve Static Functionality in Kotlin - Use the Package-Level Function to Achieve Static Functionality in Kotlin
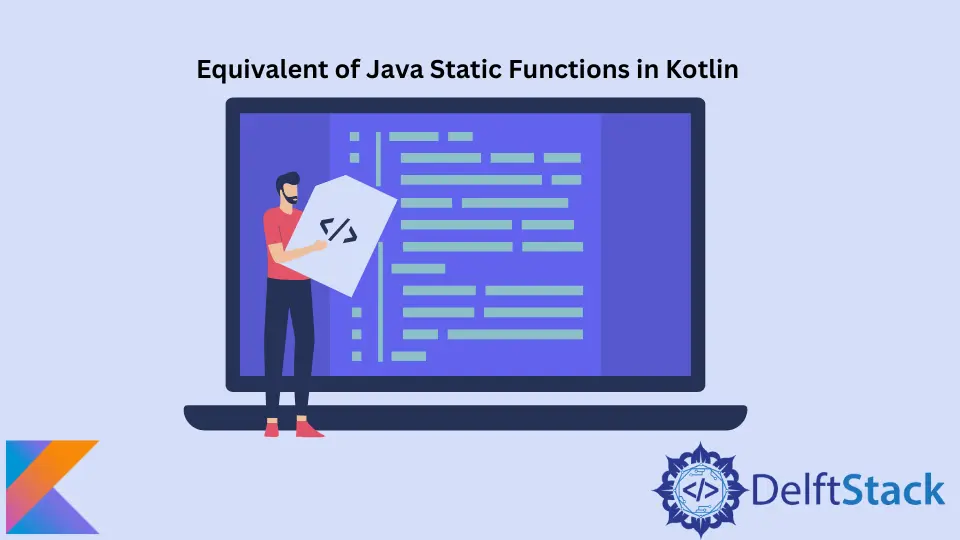
Android developers that use the Java programming language are well aware of the static
keyword. Java’s static
keyword is a non-access modifier.
We can create static variables and methods in Java with the static
keyword. It helps with memory management efficiency.
Java Virtual Machine (JVM) allocates memory to these variables and functions only once, making them memory efficient. But Kotlin is now the official language for android development.
As developers move from Java to Kotlin, one of the biggest hurdles is creating static functions in Kotlin. There’s no such keyword as static
in Kotlin.
In this article, we will learn the equivalent of Java’s static
keyword in Kotlin.
Kotlin’s Equivalent for Java’s Static Functions
While the Kotlin programming language does not have the static
keyword, we can still achieve the static functionality in Kotlin.
The core benefit of the static method is memory management efficiency. Hence, to achieve static functionality, we need to find a way that ensures that the memory is allocated only once and the value is not changeable.
There are three ways to achieve static functionality in Kotlin:
- Using the
companion
object - Using the
@JvmStatic
annotation - Using the package-level function
We will go through each of the three methods. We will also look at examples of how to implement them.
Use the Companion
Objects to Achieve Static Functionality in Kotlin
We can create a companion object using the companion
keyword. JVM will store the companion objects in the same file as the class; hence, the object can access all the private variables and functions of the class.
The companion objects are initialized along with the class; hence, the memory allocation occurs only once. The semantics of a companion object is similar to that of a Java static
initializer.
Now that we know what companion objects are and how they work, let us look at an example. In this example, we will create a companion object and see how it handles memory management to achieve static functionality in Kotlin.
fun main(args: Array<String>) {
// Accessing static variables and methods without creating objects
println("Welcome!"+'\n' + "Accessing function of the ExampleClass without creating an object."
+ '\n' + ExampleClass.staticFunction());
}
class ExampleClass{
companion object {
fun staticFunction(): String {
return "Static Method!"
}
}
}
Output:
Click here to check the demo of the example code above.
If we try to change the variable in the above code, it will throw an error. Since static
methods and variables are allocated memory only once, changing the variables will give an error.
Use the @JvmStatic
Annotation to Achieve Static Functionality in Kotlin
Besides using companion
objects, we can also use the @JvmStatic
annotation to achieve static functionality. According to the official documentation, this annotation specifies that the JVM should create an additional static method.
Using the @JvmStatic
annotation is pretty straightforward. We only need to add the annotation before the function we want to be static
.
Here’s an example to demonstrate the same.
fun main(args: Array<String>) {
// Accessing static variables and methods without creating objects
println("Hello!"+'\n' + ExampleClass.staticFunction());
}
object ExampleClass{
@JvmStatic
fun staticFunction(): String {
return "Static Method!"
}
}
Output:
Click here to check the demo of the example code above.
Use the Package-Level Function to Achieve Static Functionality in Kotlin
We can also achieve static functionality in Kotlin by declaring the method or variable a package-level member. Using package-level functions is a convenient way to achieve static functionality.
You first must create a basic .kt
file in any package and then define the method. Once defined, you can import the package and call the method from any class.
Here’s how you can do it.
// create a .kt file first in any package and define the method
package example.app
fun product(x: Int, y: Int){
return x*y
}
// after defining the method, import the package and call it from any class
import example.app.product
product (3*7)
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn